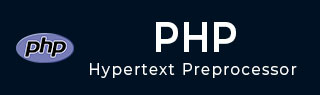
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型强制转换
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc & Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 飞船运算符
- PHP 控制语句
- PHP - 决策
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - Traits
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮箱/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET & POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 净化输入
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash 消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 代码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - 调试 Bug
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP vs 框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 散列
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望值
- PHP - use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP - Ds\Collection::toArray() 函数
PHP 的Ds\Collection::toArray()函数用于将当前集合转换为数组,并返回数组格式的集合元素。无论初始输入是集合、牌组、堆栈、向量等,此函数都会直接将其转换为数组格式。
在 PHP 中,数组是一种特殊的变量,可以在单个名称下保存多个值。您可以通过引用索引号或名称来访问这些值。
语法
以下是 PHP Ds\Collection::toArray() 函数的语法:
abstract public array Ds\Collection::toArray( void )
参数
此函数不接受任何参数。
返回值
此函数返回当前集合的元素,格式为数组。
示例 1
以下程序演示了 PHP Ds\Collection::toArray() 函数的用法:
<?php $collection = new \Ds\Vector([10, 15, 20, 25, 30]); echo "The vector elements are: \n"; print_r($collection); #using the toArray() function echo "An array elements are: \n"; var_dump($collection->toArray()); ?>
输出
以上程序产生以下输出:
The vector elements are: Ds\Vector Object ( [0] => 10 [1] => 15 [2] => 20 [3] => 25 [4] => 30 ) An array elements are: array(5) { [0]=> int(10) [1]=> int(15) [2]=> int(20) [3]=> int(25) [4]=> int(30) }
示例 2
以下是 PHP Ds\Collection::toArray() 函数的另一个示例。我们使用此函数将当前集合转换为数组格式:
<?php $set = new \Ds\Set(); $set->add('Sunday'); $set->add('Monday'); $set->add('Tuesday'); $set->add('Wednesday'); $set->add('Thursday'); $set->add('Friday'); $set->add('Saturday'); echo "The set elements are: \n"; print_r($set); #using toArray() function $res=$set->toArray(); echo "An array elements are: \n"; print_r($res); ?>
输出
执行上述程序后,将显示以下输出:
The set elements are: Ds\Set Object ( [0] => Sunday [1] => Monday [2] => Tuesday [3] => Wednesday [4] => Thursday [5] => Friday [6] => Saturday ) An array elements are: Array ( [0] => Sunday [1] => Monday [2] => Tuesday [3] => Wednesday [4] => Thursday [5] => Friday [6] => Saturday )
示例 3
在下面的示例中,我们使用 PHP Ds\Collection::toArray() 函数将当前集合转换为数组格式:
<?php $seq = new \Ds\Vector(["test_string", 1525, false]); echo "The vector elements are: \n"; var_dump($seq); #using toArray() function $res = $seq->toArray(); echo "An array elements are: \n"; var_dump($res); ?>
输出
执行上述程序后,将生成以下输出:
The vector elements are: object(Ds\Vector)#1 (3) { [0]=> string(11) "test_string" [1]=> int(1525) [2]=> bool(false) } An array elements are: array(3) { [0]=> string(11) "test_string" [1]=> int(1525) [2]=> bool(false) }
示例 4
让我们创建一个名为 stack([]) 的另一个集合,并使用相同的 toArray() 函数将其转换为数组格式:
<?php $stack = new \Ds\Stack(); $stack->push(2412); $stack->push(1262); $stack->push(457, 57, 58584); $stack->push(...[558, 6566]); echo "Stack elements are: \n"; print_r($stack); #using toArray() function $res=$stack->toArray(); echo "An array elements are: \n"; print_r($res); ?>
输出
执行上述程序后,将显示以下输出:
Stack elements are: Ds\Stack Object ( [0] => 6566 [1] => 558 [2] => 58584 [3] => 57 [4] => 457 [5] => 1262 [6] => 2412 ) An array elements are: Array ( [0] => 6566 [1] => 558 [2] => 58584 [3] => 57 [4] => 457 [5] => 1262 [6] => 2412 )
php_function_reference.htm
广告