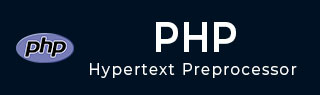
- PHP 教程
- PHP - 首页
- PHP - 路线图
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型强制转换
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较运算符(飞船运算符)
- PHP 控制语句
- PHP - 决策
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理CSV文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web开发
- PHP - Web概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET & POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session选项
- PHP - 发送邮件
- PHP - 输入过滤
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug调试
- PHP - 给C开发者
- PHP - 给PERL开发者
- PHP - 框架
- PHP - Core PHP vs. 框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望值
- PHP - Use语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI进程
- PHP - PDO扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP - Ds\Hashable::hash() 函数
PHP 的 Ds\Hashable::hash() 函数用于确定对象的哈希值。当使用对象作为基于哈希的集合(如哈希表或集合)的键时,此函数非常有用。
语法
以下是PHP Ds\Hashable::hash() 函数的语法:
mixed public Ds\Hashable::hash()
参数
hash() 函数不接受任何参数。
返回值
此函数返回一个标量值,用作哈希值。
PHP版本
hash() 函数从 PECL Ds 扩展的 1.0.0 版本开始可用。
示例1
首先,我们将向您展示PHP Ds\Hashable::hash() 函数的基本示例,以获取对象的哈希值。
<?php // Create a class Person and implement Ds\Hashable class Person implements Ds\Hashable { private $name; private $age; public function __construct($name, $age) { $this->name = $name; $this->age = $age; } public function hash() { return md5($this->name . $this->age); } public function equals($obj): bool { return $this->name === $obj->name && $this->age === $obj->age; } } $person = new Person("Ankit", 33); echo $person->hash(); ?>
输出
以上代码将产生类似这样的结果:
4ad162f31dbd28172e2dc04a2f033f47
示例2
此示例演示如何在一个集合中使用可哈希对象,以确保根据哈希值的唯一性。
<?php // Create a Product class and implement Ds\Hashable class Product implements Ds\Hashable { private $id; private $name; public function __construct($id, $name) { $this->id = $id; $this->name = $name; } public function hash() { return $this->id; } public function equals($obj): bool { return $this->id === $obj->id; } } $product1 = new Product(101, "Laptop"); $product2 = new Product(102, "Smartphone"); $set = new Ds\Set(); $set->add($product1); $set->add($product2); foreach ($set as $product) { echo $product->hash() . "\n"; } ?>
输出
运行上述程序后,它将生成以下输出:
101 102
示例3
现在,我们将使用hash()函数为组合多个属性的对象创建一个自定义哈希函数。
<?php // Create a class Book and implement Ds\Hashable class Book implements Ds\Hashable { private $title; private $author; public function __construct($title, $author) { $this->title = $title; $this->author = $author; } public function hash() { return sha1($this->title . $this->author); } public function equals($obj): bool { return $this->title === $obj->title && $this->author === $obj->author; } } $book = new Book("1984", "George Orwell"); echo "Hash value is as follows: \n"; echo $book->hash(); ?>
输出
这将创建以下输出:
Hash value is as follows: f54227c9321449aea0c1ddcd9d02228a09b6bcbc
示例4
在下面的示例中,我们使用hash()函数来比较两个对象,并查看它们根据其哈希值是否相等。
<?php // Create a Car class and implement Ds\Hashable class Car implements Ds\Hashable { private $make; private $model; public function __construct($make, $model) { $this->make = $make; $this->model = $model; } public function hash() { return crc32($this->make . $this->model); } public function equals($obj): bool { return $this->make === $obj->make && $this->model === $obj->model; } } $car1 = new Car("Maruti Suzuki", "Baleno"); $car2 = new Car("Maruti Suzuki", "Baleno"); if ($car1->equals($car2)) { echo "Cars are identical."; } else { echo "Cars are different."; } ?>
输出
运行上述程序后,它将产生以下输出:
Cars are identical.
php_function_reference.htm
广告