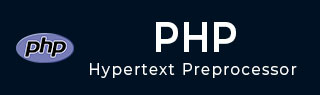
- PHP 教程
- PHP - 首页
- PHP - 路线图
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔法常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混合
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc 和 Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 宇宙飞船运算符
- PHP 控制语句
- PHP - 决策制定
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - Final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET 和 POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 对输入进行消毒
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP - Ds\PriorityQueue::jsonSerialize() 函数
PHP 的 Ds\PriorityQueue::jsonSerialize() 函数用于返回可以转换为 JSON 的表示形式。我们永远不应该直接调用此函数。
语法
以下是 PHP Ds\PriorityQueue::jsonSerialize() 函数的语法:
public Ds\PriorityQueue::jsonSerialize(): array
参数
jsonSerialize() 函数不接受任何参数。
返回值
此函数返回一个表示元素的数组。
PHP 版本
jsonSerialize() 函数从 Ds 扩展的 1.0.0 版本开始可用。
示例 1
首先,我们将向您展示 PHP Ds\PriorityQueue::jsonSerialize() 函数的基本示例,以了解其工作原理。
<?php // Create a new priority queue $queue = new \Ds\PriorityQueue(); // Add some elements with priorities $queue->push("apple", 2); $queue->push("banana", 1); $queue->push("cherry", 3); // Convert the priority queue to JSON $json = json_encode($queue->jsonSerialize()); // Display the JSON string echo $json; ?>
输出
以上代码将产生类似以下的结果:
["cherry","apple","banana"]
示例 2
现在,我们将使用 jsonSerialize() 函数处理嵌套的优先级队列并显示结果。
<?php // Create a new PriorityQueue $queue1 = new \Ds\PriorityQueue(); $queue2 = new \Ds\PriorityQueue(); // Add elements to the first queue $queue1->push("apple", 2); $queue1->push("banana", 1); // Add the first queue to the second queue $queue2->push($queue1, 3); $queue2->push("cherry", 2); // Serialize the outer priority queue to JSON $json = json_encode($queue2->jsonSerialize()); // Display the JSON string echo $json; ?>
输出
这将生成以下输出:
[["apple","banana"],"cherry"]
示例 3
现在,在下面的代码中,我们将使用 jsonSerialize() 函数序列化并显示按优先级排序的元素,并将其打印出来。
<?php // Create a new PriorityQueue $queue = new \Ds\PriorityQueue(); // Add elements with different priorities $queue->push("task1", 5); $queue->push("task2", 2); $queue->push("task3", 8); // Serialize the priority queue to JSON $json = json_encode($queue->jsonSerialize()); // Display the JSON string echo $json; ?>
输出
这将创建以下输出:
["task3","task1","task2"]
示例 4
在下面的示例中,我们使用 jsonSerialize() 函数添加带有其优先级的自定义对象。
<?php class Task { public $name; public $designation; public function __construct($name, $designation) { $this->name = $name; $this->designation = $designation; } } // Ensure the Ds extension is enabled $queue = new \Ds\PriorityQueue(); // Add custom objects with priorities $queue->push(new Task("Amit", "Engineer"), 2); $queue->push(new Task("Deepak", "Doctor"), 1); $queue->push(new Task("Sakshi", "Teacher"), 3); // Serialize the priority queue to JSON $json = json_encode($queue->jsonSerialize()); // Display the JSON string echo $json; ?>
输出
以下是以上代码的输出:
[{"name":"Sakshi","designation":"Teacher"},{"name":"Amit","designation":"Engineer"},{"name":"Deepak","designation":"Doctor"}]
php_function_reference.htm
广告