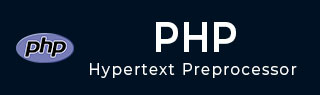
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型混淆
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc & Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 比较符
- PHP 控制语句
- PHP - 决策
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 具名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象的 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - 特性
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET & POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 输入过滤
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP 与框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望值
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
PHP closedir() 函数
PHP 的 closedir() 函数代表“关闭目录”。此函数用于关闭目录句柄。
要对文件和文件夹执行任何操作,我们必须打开包含这些文件和其他文件夹的目录。当我们打开目录时,opendir() 函数会为我们提供一个目录句柄。它可以用于执行进一步的相关任务。最后,使用 closedir() 函数关闭目录句柄资源。
重要提示
- 打开的目录可以用于多种用途。因此,打开的资源不会过期,除非您手动关闭它。因此,closedir() 函数通过关闭目录句柄来提高资源效率。
- 在关闭文件句柄之前,使用 is_resource() 函数确定它是否是资源。这可以避免您遇到错误。
- 如果您尝试关闭已关闭的目录句柄,则会发生 Uncaught TypeError 错误。因此,在关闭它之前,您需要确认已定义的目录句柄是一个资源。
语法
以下是 PHP 的 closedir() 函数的语法:
void closedir ( resource $dir_handle );
参数
以下是 closedir() 函数的必需和可选参数:
序号 | 参数及描述 |
---|---|
1 |
dir_handle(可选) 这是使用 opendir() 方法先前打开的目录的句柄。 |
返回值
该函数不返回值。
PHP 版本
closedir() 函数在 PHP 4 核心版本中引入,并与 PHP 5、PHP 7 和 PHP 8 兼容。
示例
在这个示例中,我们将向您展示 PHP closedir() 函数的基本用法。我们将结合使用 opendir() 和 readdir() 函数以及 closedir()。如上所述,要关闭特定目录,我们必须使用 opendir() 和 readdir() 函数打开并读取它。因此,读取内容后,我们可以使用 closedir() 函数关闭它。
<?php // open the directory using opendir() $dir = opendir("/var/www/images"); // Check if the directory handle is valid if ($dir) { // Loop through the directory and read each file while (($file = readdir($dir)) !== false) { echo "filename: " . $file . "<br>"; } // Close the directory closedir($dir); // Show message that directory is closed echo "The directory has been closed."; } else { echo "Could not open the directory."; } ?>
输出
这将产生以下结果:
filename: . filename: .. filename: logo.gif filename: mohd.gif The directory has been closed.
示例
在这个 PHP 示例中,我们将使用 closedir() 函数,并且不会在函数中提及目录句柄。并显示一条消息来告知用户目录已关闭。
<?php $dir = opendir("/var/www/images"); if ($dir) { while (($file = readdir($dir)) !== false) { echo "filename: " . $file . "<br>"; } // close the directory without mentioning the directory handle closedir($dir); echo "The directory has been closed."; } else { echo "Could not open the directory."; } ?>
输出
这将给出以下结果:
filename: . filename: .. filename: logo.gif filename: mohd.gif The directory has been closed.
示例
在此 PHP 代码中,我们将通过确认用户是否要关闭它来安全地关闭目录。因此,使用这种方法,我们为用户提供了额外的保障。显示一条消息,表明目录已关闭。如果 $confirmClose 为 false,则显示一条消息,指出目录未关闭。
<?php // open the directory $dir = opendir("/var/www/images"); // check if the directory handle is valid if ($dir) { // loop over the directory and read each file while (($file = readdir($dir)) !== false) { echo "Filename: " . $file . "<br>"; } // Confirm before closing the directory handle $confirmClose = true; if ($confirmClose) { // close the directory using closedir() closedir($dir); // show message that directory is closed echo "The directory has been closed."; } else { echo "The directory was not closed."; } } else { echo "Could not open the directory."; } ?>
输出
这将导致以下结果:
Filename: . Filename: .. Filename: index.php Filename: new dir Filename: images Filename: my.php The directory has been closed.
总结
使用完目录句柄后,需要将其关闭。我们可以通过内置的目录函数 closedir() 来完成此任务。
php_function_reference.htm
广告