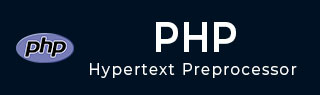
- PHP 教程
- PHP - 首页
- PHP - 简介
- PHP - 安装
- PHP - 历史
- PHP - 特性
- PHP - 语法
- PHP - Hello World
- PHP - 注释
- PHP - 变量
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ 和 $$ 变量
- PHP - 常量
- PHP - 魔术常量
- PHP - 数据类型
- PHP - 类型转换
- PHP - 类型强制转换
- PHP - 字符串
- PHP - 布尔值
- PHP - 整数
- PHP - 文件与 I/O
- PHP - 数学函数
- PHP - Heredoc & Nowdoc
- PHP - 复合类型
- PHP - 文件包含
- PHP - 日期与时间
- PHP - 标量类型声明
- PHP - 返回类型声明
- PHP 运算符
- PHP - 运算符
- PHP - 算术运算符
- PHP - 比较运算符
- PHP - 逻辑运算符
- PHP - 赋值运算符
- PHP - 字符串运算符
- PHP - 数组运算符
- PHP - 条件运算符
- PHP - 展开运算符
- PHP - 空值合并运算符
- PHP - 宇宙飞船运算符
- PHP 控制语句
- PHP - 决策制定
- PHP - If…Else 语句
- PHP - Switch 语句
- PHP - 循环类型
- PHP - For 循环
- PHP - Foreach 循环
- PHP - While 循环
- PHP - Do…While 循环
- PHP - Break 语句
- PHP - Continue 语句
- PHP 函数
- PHP - 函数
- PHP - 函数参数
- PHP - 按值传递
- PHP - 按引用传递
- PHP - 默认参数
- PHP - 命名参数
- PHP - 可变参数
- PHP - 返回值
- PHP - 传递函数
- PHP - 递归函数
- PHP - 类型提示
- PHP - 变量作用域
- PHP - 严格类型
- PHP - 匿名函数
- PHP - 箭头函数
- PHP - 可变函数
- PHP - 局部变量
- PHP - 全局变量
- PHP 超全局变量
- PHP - 超全局变量
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP 文件处理
- PHP - 文件处理
- PHP - 打开文件
- PHP - 读取文件
- PHP - 写入文件
- PHP - 文件是否存在
- PHP - 下载文件
- PHP - 复制文件
- PHP - 追加文件
- PHP - 删除文件
- PHP - 处理 CSV 文件
- PHP - 文件权限
- PHP - 创建目录
- PHP - 列出文件
- 面向对象的 PHP
- PHP - 面向对象编程
- PHP - 类和对象
- PHP - 构造函数和析构函数
- PHP - 访问修饰符
- PHP - 继承
- PHP - 类常量
- PHP - 抽象类
- PHP - 接口
- PHP - Traits
- PHP - 静态方法
- PHP - 静态属性
- PHP - 命名空间
- PHP - 对象迭代
- PHP - 封装
- PHP - final 关键字
- PHP - 重载
- PHP - 克隆对象
- PHP - 匿名类
- PHP Web 开发
- PHP - Web 概念
- PHP - 表单处理
- PHP - 表单验证
- PHP - 表单邮件/URL
- PHP - 完整表单
- PHP - 文件包含
- PHP - GET & POST
- PHP - 文件上传
- PHP - Cookie
- PHP - Session
- PHP - Session 选项
- PHP - 发送邮件
- PHP - 过滤输入
- PHP - Post-Redirect-Get (PRG)
- PHP - 闪存消息
- PHP 高级
- PHP - MySQL
- PHP.INI 文件配置
- PHP - 数组解构
- PHP - 编码规范
- PHP - 正则表达式
- PHP - 错误处理
- PHP - Try…Catch
- PHP - Bug 调试
- PHP - 针对 C 开发人员
- PHP - 针对 PERL 开发人员
- PHP - 框架
- PHP - Core PHP vs. 框架
- PHP - 设计模式
- PHP - 过滤器
- PHP - JSON
- PHP - 异常
- PHP - 特殊类型
- PHP - 哈希
- PHP - 加密
- PHP - is_null() 函数
- PHP - 系统调用
- PHP - HTTP 认证
- PHP - 交换变量
- PHP - Closure::call()
- PHP - 过滤后的 unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - 期望值
- PHP - Use 语句
- PHP - 整数除法
- PHP - 已弃用的特性
- PHP - 已移除的扩展和 SAPI
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI 进程
- PHP - PDO 扩展
- PHP - 内置函数
- PHP 有用资源
- PHP - 速查表
- PHP - 问答
- PHP - 快速指南
- PHP - 在线编译器
- PHP - 有用资源
- PHP - 讨论
PHP 文件系统 stat() 函数
PHP 文件系统stat()函数用于返回有关文件的信息。此函数可以收集名为 filename 的文件统计信息。如果 filename 是符号链接,则统计信息来自文件本身,而不是符号链接。lstat() 函数类似于 stat() 函数,但它可以基于符号链接的状态。
语法
以下是 PHP 文件系统stat()函数的语法:
array stat ( string $filename )
参数
以下是stat()函数唯一必需的参数:
序号 | 参数及描述 |
---|---|
1 |
$filename(必需) 这是要获取其信息的的文件。 |
返回值
stat()函数在成功时返回一个包含文件详细信息的数组,如果出错则返回 FALSE。
数组元素
以下是数组的主要元素:
索引 | 字段 | 描述 |
---|---|---|
[0] | dev | 设备号 |
[1] | ino | inode 号 |
[2] | mode | 文件模式(保护) |
[3] | nlink | 链接数 |
[4] | uid | 所有者的用户 ID |
[5] | gid | 所有者的组 ID |
[6] | rdev | 设备类型(如果为 inode 设备) |
[7] | size | 文件大小(字节) |
[8] | atime | 上次访问时间 |
[9] | mtime | 上次修改时间 |
[10] | ctime | 上次更改时间 |
[11] | blksize | 文件系统 I/O 的块大小 |
[12] | blocks | 已分配的 512B 块数 |
PHP 版本
stat()函数最初作为核心 PHP 4 的一部分引入,并能很好地与 PHP 5、PHP 7 和 PHP 8 兼容。
示例
这是一个基本示例,演示如何使用 PHP 文件系统stat()函数获取文件信息。
<?php // Get file stat $stat = stat("/PhpProject/sample.txt"); // Print file access time, this is the same as calling fileatime() echo "Acces time: " .$stat["atime"]; //Print file modification time, this is the same as calling filemtime() echo "\nModification time: " .$stat["mtime"]; ?>
输出
以下是以下代码的结果:
Acces time: 1590217956 Modification time: 1591617832 Device number: 1245376677
示例
这是一个另一个示例,演示如何使用stat()函数处理使用此函数时的错误。
<?php $stat = stat("/PhpProject/sample.txt"); if(!$stat) { echo "stat() call failed..."; } else { $atime = $stat["atime"] + 604800; } if(!touch("/PhpProject1/sampl2.txt", time(), $atime)) { echo "failed to touch file..."; } else { echo "touch() returned success..."; } ?>
输出
这将产生以下结果:
touch() returned success...
示例
这是一个示例,用于检查提供文件是否存在,如果存在,则使用stat()函数获取信息。
<?php $filename = "/PhpProjects/myfile.txt"; if (file_exists($filename)) { $fileinfo = stat($filename); echo "File exists!\n"; echo "File size: " . $fileinfo['size'] . " bytes\n"; echo "Last modified: " . date('Y-m-d H:i:s', $fileinfo['mtime']) . "\n"; } else { echo "File does not exist.
"; } ?>
输出
这将生成以下输出:
File exists! File size: 74 bytes Last modified: 2024-06-25 09:08:39
示例
这是一个示例,使用stat()函数获取文件信息,例如文件大小、上次修改日期和权限。
<?php $filename = "/PhpProjects/myfile.txt"; // Get file status $fileinfo = stat($filename); // Display file size in bytes echo "File size: " . $fileinfo['size'] . " bytes\n"; // Display last modified timestamp echo "Last modified: " . date('Y-m-d H:i:s', $fileinfo['mtime']) . "\n"; // Display file permissions echo "Permissions: " . decoct($fileinfo['mode'] & 0777) . "\n"; ?>
输出
这将导致以下输出:
File size: 74 bytes Last modified: 2024-06-25 09:08:39 Permissions: 644
总结
stat()方法是一个内置函数,用于以数组的形式获取有关给定文件的信息。它对于获取文件的不同属性非常有用,例如权限、大小、修改时间和类型。
php_function_reference.htm
广告