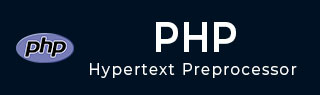
- PHP Tutorial
- PHP - Home
- PHP - Roadmap
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmetic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP Arrays
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP AJAX
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP XML
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - JSON
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - PEAR
- PHP - CSRF
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
PHP - URL urlencode() Function
The PHP URL urlencode() function is used to encode a string to be used in a URL. It is useful when an encoded string is used in the URL's query part to send variables to the next page.
Syntax
Below is the syntax of the PHP URL urlencode() function −
string urlencode( string $str )
Parameters
This function accepts $str parameter which is the string to be encoded.
Return Value
The urlencode() function returns a string in which all non-alphanumeric characters except "-_." are replaced with a percent (%) sign followed by two hex digits and spaces encoded as plus (+) symbols. It is encoded in the same way as uploaded data from a WWW form is encoded, which is the same as the media type application/x-www-form-urlencoded. It differs from RFC 3986 encoding for historical reasons, with spaces encoded as plus (+) signs.
PHP Version
Introduced in core PHP 4, the urlencode() function continues to function easily in PHP 5, PHP 7, and PHP 8.
Example 1
Here we will show you the basic example of the PHP URL urlencode() function to encode a user input string which contains special characters.
<?php // Mention user input here $userInput = 'ExampleInput#&=+'; echo "UserInput: $userInput\n"; echo '<a href="mycgi?foo=', urlencode($userInput), '">'; ?>
Output
The above code will result something like this −
UserInput: ExampleInput#&=+ <a href="mycgi?foo=ExampleInput%23%26%3D%2B">
Example 2
Now we will try to use the urlencode() function and encode multiple urls at the same time.
<?php // encode the given urls echo urlencode("https://tutorialspoint.com") . "\n"; echo urlencode("https://tutorialspoint.com/php/index.htm") . "\n"; echo urlencode("https://tutorialspoint.com/html/index.htm") . "\n"; echo urlencode("https://tutorialspoint.com/tutorialslibrary.htm") . "\n"; ?>
Output
After running the above program, it generates the following output −
https%3A%2F%2Fwww.tutorialspoint.com https%3A%2F%2Fwww.tutorialspoint.com%2Fphp%2Findex.htm https%3A%2F%2Fwww.tutorialspoint.com%2Fhtml%2Findex.htm https%3A%2F%2Fwww.tutorialspoint.com%2Ftutorialslibrary.htm
Example 3
This example shows how to encode multiple user input strings for safe inclusion in a URL query string. The encoded strings are then contained in the href attribute of an anchor (<a>) tag.
<?php // Mention user inputs here $input1 = 'Special@Input$%*'; $input2 = "Not the same content as $input1"; echo "input1: $input1\n"; echo "input2: $input2\n"; $query_string = 'input1=' . urlencode($input1) . '&input2=' . urlencode($input2); echo '<a href="mycgi?' . htmlentities($query_string) . '">'; ?>
Output
This will create the below output −
input1: Special@Input$%* input2: Not the same content as Special@Input$%* <a href="mycgi?input1=Special%40Input%24%25%2A&input2=Not+the+same+content+as+Special%40Input%24%25%2A">
Example 4
This example will use the urlencode() function to encode a string which contains special characters like @, #, and &.
<?php // Mention the email address here $str = "[email protected]&subject=Hello#World!"; $encodedStr = urlencode($str); echo $encodedStr; ?>
Output
When the above program is executed, it will produce the below output −
email%40tutorialspoint.com%26subject%3DHello%23World%21