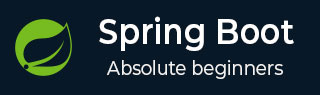
- Spring Boot 教程
- Spring Boot - 首页
- Spring Boot - 简介
- Spring Boot - 快速入门
- Spring Boot - 引导启动
- Spring Tool Suite
- Spring Boot - Tomcat 部署
- Spring Boot - 构建系统
- Spring Boot - 代码结构
- Spring Bean & 依赖注入
- Spring Boot - 运行器
- Spring Boot - 启动器
- Spring Boot - 应用程序属性
- Spring Boot - 配置
- Spring Boot - 注解
- Spring Boot - 日志
- 构建 RESTful Web 服务
- Spring Boot - 异常处理
- Spring Boot - 拦截器
- Spring Boot - Servlet 过滤器
- Spring Boot - Tomcat 端口号
- Spring Boot - Rest 模板
- Spring Boot - 文件处理
- Spring Boot - 服务组件
- Spring Boot - Thymeleaf
- 消费 RESTful Web 服务
- Spring Boot - CORS 支持
- Spring Boot - 国际化
- Spring Boot - 调度
- Spring Boot - 启用 HTTPS
- Spring Boot - Eureka 服务器
- 使用 Eureka 进行服务注册
- 网关代理服务器和路由
- Spring Cloud 配置服务器
- Spring Cloud 配置客户端
- Spring Boot - Actuator
- Spring Boot - Admin 服务器
- Spring Boot - Admin 客户端
- Spring Boot - 启用 Swagger2
- Spring Boot - 使用 SpringDoc OpenAPI
- Spring Boot - 创建 Docker 镜像
- 跟踪微服务日志
- Spring Boot - Flyway 数据库
- Spring Boot - 发送邮件
- Spring Boot - Hystrix
- Spring Boot - WebSocket
- Spring Boot - 批处理服务
- Spring Boot - Apache Kafka
- Spring Boot - Twilio
- Spring Boot - 单元测试用例
- Rest Controller 单元测试
- Spring Boot - 数据库处理
- 保护 Web 应用程序
- Spring Boot - 使用 JWT 的 OAuth2
- Spring Boot - Google Cloud Platform
- Spring Boot - Google OAuth2 登录
- Spring Boot 资源
- Spring Boot - 快速指南
- Spring Boot - 有用资源
- Spring Boot - 讨论
Spring Boot - 引导启动
本章将向您解释如何在 Spring Boot 应用程序上执行引导启动。
Spring Initializer
引导启动 Spring Boot 应用程序的一种方法是使用 Spring Initializer。为此,您需要访问 Spring Initializer 网页 www.start.spring.io 并选择您的构建、Spring Boot 版本和平台。此外,您需要提供一个组、工件和运行应用程序所需的依赖项。
观察以下屏幕截图,其中显示了一个示例,我们在其中添加了spring-boot-starter-web依赖项以编写 REST 端点。
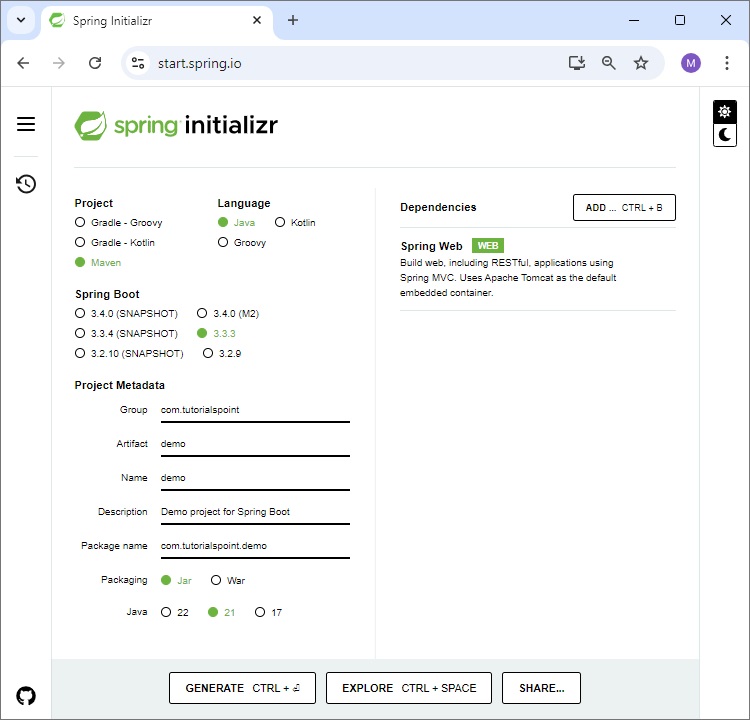
提供完组、工件、依赖项、构建项目、平台和版本后,单击生成项目按钮。zip 文件将下载,并且文件将被提取。
本节将通过使用 Maven 和 Gradle 来解释示例。
Maven
下载项目后,解压缩文件。现在,您的pom.xml文件如下所示:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>3.3.3</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.tutorialspoint</groupId> <artifactId>demo</artifactId> <version>0.0.1-SNAPSHOT</version> <name>demo</name> <description>Demo project for Spring Boot</description> <url/> <licenses> <license/> </licenses> <developers> <developer/> </developers> <scm> <connection/> <developerConnection/> <tag/> <url/> </scm> <properties> <java.version>21</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Gradle
对于 Gradle,您可以检查 build.gradle 如下
build.gradle
buildscript { ext { springBootVersion = '3.3.3' } repositories { mavenCentral() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") } } apply plugin: 'java' apply plugin: 'eclipse' apply plugin: 'org.springframework.boot' group = 'com.tutorialspoint' version = '0.0.1-SNAPSHOT' sourceCompatibility = 21 repositories { mavenCentral() } dependencies { compile('org.springframework.boot:spring-boot-starter-web') testCompile('org.springframework.boot:spring-boot-starter-test') }
类路径依赖项
Spring Boot 提供了许多启动器以将 jar 添加到我们的类路径中。例如,要编写 Rest 端点,我们需要在类路径中添加spring-boot-starter-web依赖项。观察下面显示的代码以更好地理解:
Maven 依赖项
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies>
Gradle 依赖项
dependencies { compile('org.springframework.boot:spring-boot-starter-web') }
主方法
主方法应该编写 Spring Boot 应用程序类。此类应使用@SpringBootApplication进行注释。这是启动 Spring Boot 应用程序的入口点。您可以在src/java/main目录下找到默认包的主类文件。
在此示例中,主类文件位于src/java/main目录下,默认包为com.tutorialspoint.demo。观察此处显示的代码以更好地理解:
package com.tutorialspoint.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
编写 Rest 端点
要在 Spring Boot 应用程序的主类文件中编写一个简单的 Hello World Rest 端点,请按照以下步骤操作:
首先,在类的顶部添加@RestController注释。
现在,使用@GetMapping注释编写一个请求 URI 方法。
然后,请求 URI 方法应返回Hello World字符串。
现在,您的 Spring Boot 应用程序主类文件将如下所示:
package com.tutorialspoint.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController @SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } @GetMapping(value = "/") public String hello() { return "Hello World"; } }
创建可执行 JAR
让我们使用命令提示符中的 Maven 命令创建一个可执行 JAR 文件以运行 Spring Boot 应用程序,如下所示:
使用 Maven 命令 mvn clean install,如下所示:
E:\dev\demo>mvn clean install
您将看到类似于以下的结果
[INFO] Scanning for projects... [INFO] [INFO] [1m----------------------< [0;36mcom.tutorialspoint:demo[0;1m >-----------------------[m [INFO] [1mBuilding demo 0.0.1-SNAPSHOT[m [INFO] from pom.xml [INFO] [1m--------------------------------[ jar ]---------------------------------[m [INFO] Downloading from central: https://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-install-plugin/3.1.3/maven-install-plugin-3.1.3.pom .... [INFO] [INFO] [1m--- [0;32mclean:3.3.2:clean[m [1m(default-clean)[m @ [36mdemo[0;1m ---[m [INFO] Deleting E:\Dev\demo\target [INFO] [INFO] [1m--- [0;32mresources:3.3.1:resources[m [1m(default-resources)[m @ [36mdemo[0;1m ---[m [INFO] Copying 1 resource from src\main\resources to target\classes [INFO] Copying 0 resource from src\main\resources to target\classes [INFO] ... [INFO] [INFO] [1m--- [0;32msurefire:3.2.5:test[m [1m(default-test)[m @ [36mdemo[0;1m ---[m [INFO] Using auto detected provider org.apache.maven.surefire.junitplatform.JUnitPlatformProvider [INFO] Downloading from central: https://repo.maven.apache.org/maven2/org/junit/platform/junit-platform-launcher/1.10.3/junit-platform-launcher-1.10.3.pom [INFO] Downloaded from central: https://repo.maven.apache.org/maven2/org/junit/platform/junit-platform-launcher/1.10.3/junit-platform-launcher-1.10.3.pom (3.0 kB at 13 kB/s) [INFO] [INFO] ------------------------------------------------------- [INFO] T E S T S [INFO] ------------------------------------------------------- [INFO] Running com.tutorialspoint.demo.[1mDemoApplicationTests[m 17:35:15.626 [main] INFO org.springframework.test.context.support.AnnotationConfigContextLoaderUtils -- Could not detect default configuration classes for test class [com.tutorialspoint.demo.DemoApplicationTests]: DemoApplicationTests does not declare any static, non-private, non-final, nested classes annotated with @Configuration. 17:35:15.802 [main] INFO org.springframework.boot.test.context.SpringBootTestContextBootstrapper -- Found @SpringBootConfiguration com.tutorialspoint.demo.DemoApplication for test class com.tutorialspoint.demo.DemoApplicationTests . ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v3.3.3) 2024-09-02T17:35:16.380+05:30 INFO 6204 --- [demo] [ main] c.t.demo.DemoApplicationTests : Starting DemoApplicationTests using Java 21.0.3 with PID 6204 (started by Tutorialspoint in E:\Dev\demo) 2024-09-02T17:35:16.381+05:30 INFO 6204 --- [demo] [ main] c.t.demo.DemoApplicationTests : No active profile set, falling back to 1 default profile: "default" 2024-09-02T17:35:18.173+05:30 INFO 6204 --- [demo] [ main] c.t.demo.DemoApplicationTests : Started DemoApplicationTests in 2.162 seconds (process running for 4.295) ... [INFO] Results: [INFO] [INFO] [1;32mTests run: 1, Failures: 0, Errors: 0, Skipped: 0[m [INFO] [INFO] [INFO] [1m--- [0;32mjar:3.4.2:jar[m [1m(default-jar)[m @ [36mdemo[0;1m ---[m [INFO] Building jar: E:\Dev\demo\target\demo-0.0.1-SNAPSHOT.jar [INFO] [INFO] [1m--- [0;32mspring-boot:3.3.3:repackage[m [1m(repackage)[m @ [36mdemo[0;1m ---[m [INFO] Replacing main artifact E:\Dev\demo\target\demo-0.0.1-SNAPSHOT.jar with repackaged archive, adding nested dependencies in BOOT-INF/. [INFO] The original artifact has been renamed to E:\Dev\demo\target\demo-0.0.1-SNAPSHOT.jar.original [INFO] [INFO] [1m--- [0;32minstall:3.1.3:install[m [1m(default-install)[m @ [36mdemo[0;1m ---[m .... [INFO] Downloaded from central: https://repo.maven.apache.org/maven2/org/codehaus/plexus/plexus-xml/3.0.1/plexus-xml-3.0.1.jar (94 kB at 806 kB/s) [INFO] Installing E:\Dev\demo\pom.xml to C:\Users\Tutorialspoint\.m2\repository\com\tutorialspoint\demo\0.0.1-SNAPSHOT\demo-0.0.1-SNAPSHOT.pom [INFO] Installing E:\Dev\demo\target\demo-0.0.1-SNAPSHOT.jar to C:\Users\Tutorialspoint\.m2\repository\com\tutorialspoint\demo\0.0.1-SNAPSHOT\demo-0.0.1-SNAPSHOT.jar [INFO] [1m------------------------------------------------------------------------[m [INFO] [1;32mBUILD SUCCESS[m [INFO] [1m------------------------------------------------------------------------[m [INFO] Total time: 18.938 s [INFO] Finished at: 2024-09-02T17:35:25+05:30 [INFO] [1m------------------------------------------------------------------------[m
同样,对于 Gradle,您可以运行以下命令来构建 jar。
gradle clean build
使用 Java 运行 Hello World
创建可执行 JAR 文件后,您可以在 target 目录下找到它。在我们的例子中,它位于E: > Dev > demo > target > demo-0.0.1-SNAPSHOT.jar.
现在,使用命令java –jar <JARFILE>运行 JAR 文件。观察在上述示例中,JAR 文件名为demo-0.0.1-SNAPSHOT.jar
E:\dev\demo\target>java -jar demo-0.0.1-SNAPSHOT.jar
运行 jar 文件后,您可以在控制台窗口中看到输出,如下所示:
. ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v3.3.3) 2024-09-02T17:42:38.623+05:30 INFO 4712 --- [demo] [ main] c.tutorialspoint.demo.DemoApplication : Starting DemoApplication v0.0.1-SNAPSHOT using Java 21.0.2 with PID 4712 (E:\Dev\demo\target\demo-0.0.1-SNAPSHOT.jar started by Tutorialspoint in E:\Dev\demo\target) 2024-09-02T17:42:38.626+05:30 INFO 4712 --- [demo] [ main] c.tutorialspoint.demo.DemoApplication : No active profile set, falling back to 1 default profile: "default" 2024-09-02T17:42:40.250+05:30 INFO 4712 --- [demo] [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port 8080 (http) 2024-09-02T17:42:40.266+05:30 INFO 4712 --- [demo] [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat] 2024-09-02T17:42:40.266+05:30 INFO 4712 --- [demo] [ main] o.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/10.1.28] 2024-09-02T17:42:40.320+05:30 INFO 4712 --- [demo] [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext 2024-09-02T17:42:40.322+05:30 INFO 4712 --- [demo] [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 1574 ms 2024-09-02T17:42:40.943+05:30 INFO 4712 --- [demo] [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port 8080 (http) with context path '/' 2024-09-02T17:42:40.969+05:30 INFO 4712 --- [demo] [ main] c.tutorialspoint.demo.DemoApplication : Started DemoApplication in 3.122 seconds (process running for 3.902)
现在,查看控制台,Tomcat 在端口 8080 (http) 上启动。现在,转到 Web 浏览器并访问 URL https://127.0.0.1:8080/,您将看到如下所示的输出:
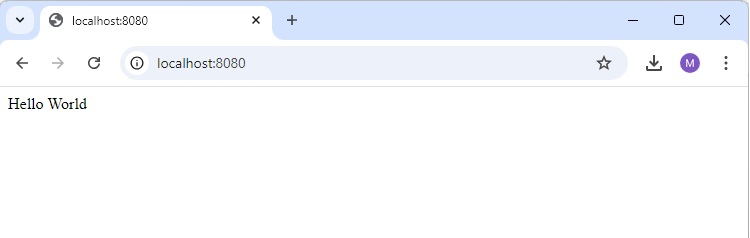