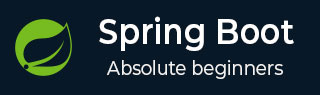
- Spring Boot 教程
- Spring Boot - 首页
- Spring Boot - 简介
- Spring Boot - 快速入门
- Spring Boot - 引导
- Spring Tool Suite
- Spring Boot - Tomcat 部署
- Spring Boot - 构建系统
- Spring Boot - 代码结构
- Spring Bean & 依赖注入
- Spring Boot - 运行器
- Spring Boot - 启动器
- Spring Boot - 应用属性
- Spring Boot - 配置
- Spring Boot - 注解
- Spring Boot - 日志
- 构建 RESTful Web 服务
- Spring Boot - 异常处理
- Spring Boot - 拦截器
- Spring Boot - Servlet 过滤器
- Spring Boot - Tomcat 端口号
- Spring Boot - Rest 模板
- Spring Boot - 文件处理
- Spring Boot - 服务组件
- Spring Boot - Thymeleaf
- 消费 RESTful Web 服务
- Spring Boot - CORS 支持
- Spring Boot - 国际化
- Spring Boot - 定时任务
- Spring Boot - 启用 HTTPS
- Spring Boot - Eureka 服务器
- 使用 Eureka 注册服务
- 网关代理服务器和路由
- Spring Cloud 配置服务器
- Spring Cloud 配置客户端
- Spring Boot - Actuator
- Spring Boot - Admin 服务器
- Spring Boot - Admin 客户端
- Spring Boot - 启用 Swagger2
- Spring Boot - 使用 SpringDoc OpenAPI
- Spring Boot - 创建 Docker 镜像
- 跟踪微服务日志
- Spring Boot - Flyway 数据库
- Spring Boot - 发送邮件
- Spring Boot - Hystrix
- Spring Boot - Web Socket
- Spring Boot - 批处理服务
- Spring Boot - Apache Kafka
- Spring Boot - Twilio
- Spring Boot - 单元测试用例
- Rest Controller 单元测试
- Spring Boot - 数据库处理
- 保护 Web 应用程序
- Spring Boot - 使用 JWT 的 OAuth2
- Spring Boot - Google Cloud Platform
- Spring Boot - Google OAuth2 登录
- Spring Boot 资源
- Spring Boot - 快速指南
- Spring Boot - 有用资源
- Spring Boot - 讨论
Spring Boot - 定时任务
调度是为特定时间段执行任务的过程。Spring Boot 为在 Spring 应用程序上编写调度程序提供了良好的支持。
Java Cron 表达式
Java Cron 表达式用于配置 CronTrigger 的实例,CronTrigger 是 org.quartz.Trigger 的子类。有关 Java cron 表达式的更多信息,您可以参考此链接:
https://docs.oracle.com/cd/E12058_01/doc/doc.1014/e12030/cron_expressions.htm
@EnableScheduling 注解用于为您的应用程序启用调度程序。此注解应添加到主 Spring Boot 应用程序类文件。
DemoApplication.java
package com.tutorialspoint.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.scheduling.annotation.EnableScheduling; @SpringBootApplication @EnableScheduling public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>3.3.3</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.tutorialspoint</groupId> <artifactId>demo</artifactId> <version>0.0.1-SNAPSHOT</version> <name>demo</name> <description>Demo project for Spring Boot</description> <url/> <licenses> <license/> </licenses> <developers> <developer/> </developers> <scm> <connection/> <developerConnection/> <tag/> <url/> </scm> <properties> <java.version>21</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
@Scheduled 注解用于为特定时间段触发调度程序。
@Scheduled(cron = "0 0-5 13 * * ?") public void cronJobSch() throws Exception { }
以下是一个示例代码,演示了如何在接下来的五分钟内,从下午 1:00 开始,每分钟执行一次任务。
Scheduler.java
package com.tutorialspoint.demo.scheduler; import java.text.SimpleDateFormat; import java.util.Date; import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Component; @Component public class Scheduler { @Scheduled(cron = "0 0-5 13 * * ?") public void cronJobSch() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS"); Date now = new Date(); String strDate = sdf.format(now); System.out.println("Java cron job expression:: " + strDate); } }
输出
以下屏幕截图显示了应用程序如何在 13:03:00 启动,并且从该时间起每分钟执行一次 cron 作业调度程序任务,根据 cron 作业表达式,它将在接下来的五分钟内执行。
. ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ [32m :: Spring Boot :: [39m [2m (v3.3.3)[0;39m [2024-09-10T13:02:12Z] [org.springframework.boot.StartupInfoLogger] [main] [50] [INFO ] Starting DemoApplication using Java 21.0.3 with PID 13256 (E:\Dev\demo\target\classes started by Tutorialspoint in E:\Dev\demo) [2024-09-10T13:02:12Z] [org.springframework.boot.SpringApplication] [main] [654] [INFO ] No active profile set, falling back to 1 default profile: "default" [2024-09-10T13:02:13Z] [org.springframework.boot.StartupInfoLogger] [main] [56] [INFO ] Started DemoApplication in 1.043 seconds (process running for 1.995) Java cron job expression:: 2024-09-10 13:03:00.091 Java cron job expression:: 2024-09-10 13:04:00.013 Java cron job expression:: 2024-09-10 13:05:00.015
固定速率
固定速率调度程序用于在特定时间执行任务。它不会等待上一个任务完成。值应以毫秒为单位。示例代码如下所示:
@Scheduled(fixedRate = 1000) public void fixedRateSch() { }
以下是一个示例代码,演示了如何在应用程序启动后每秒执行一次任务:
Scheduler.java
package com.tutorialspoint.demo.scheduler; import java.text.SimpleDateFormat; import java.util.Date; import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Component; @Component public class Scheduler { @Scheduled(fixedRate = 1000) public void fixedRateSch() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS"); Date now = new Date(); String strDate = sdf.format(now); System.out.println("Fixed Rate scheduler:: " + strDate); } }
输出
观察以下屏幕截图,它显示了应用程序在 13:06:45 启动,之后每秒执行一次固定速率调度程序任务。
Fixed Rate scheduler:: 2024-09-10 13:06:45.832 Fixed Rate scheduler:: 2024-09-10 13:06:46.813 Fixed Rate scheduler:: 2024-09-10 13:06:47.809
固定延迟
固定延迟调度程序用于在特定时间执行任务。它应该等待上一个任务完成。值应以毫秒为单位。示例代码如下所示:
@Scheduled(fixedDelay = 1000, initialDelay = 1000) public void fixedDelaySch() { }
这里,initialDelay 是任务在初始延迟值后第一次执行的时间。
以下示例演示了如何在应用程序启动完成后 3 秒后,每秒执行一次任务:
Scheduler.java
package com.tutorialspoint.demo.scheduler; import java.text.SimpleDateFormat; import java.util.Date; import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Component; @Component public class Scheduler { @Scheduled(fixedDelay = 1000, initialDelay = 3000) public void fixedDelaySch() { SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS"); Date now = new Date(); String strDate = sdf.format(now); System.out.println("Fixed Delay scheduler:: " + strDate); } }
输出
观察以下屏幕截图,它显示了应用程序在 13:11:42 启动,并且每 3 秒执行一次固定延迟调度程序任务,每秒执行一次。
. ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ [32m :: Spring Boot :: [39m [2m (v3.3.3)[0;39m [2024-09-10T13:11:42Z] [org.springframework.boot.StartupInfoLogger] [main] [50] [INFO ] Starting DemoApplication using Java 21.0.3 with PID 13660 (E:\Dev\demo\target\classes started by Tutorialspoint in E:\Dev\demo) [2024-09-10T13:11:42Z] [org.springframework.boot.SpringApplication] [main] [654] [INFO ] No active profile set, falling back to 1 default profile: "default" [2024-09-10T13:11:42Z] [org.springframework.boot.StartupInfoLogger] [main] [56] [INFO ] Started DemoApplication in 1.066 seconds (process running for 2.035) Fixed Rate scheduler:: 2024-09-10 13:11:46.061 Fixed Rate scheduler:: 2024-09-10 13:11:47.063 Fixed Rate scheduler:: 2024-09-10 13:11:48.065