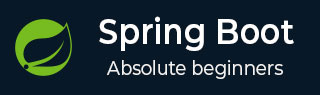
- Spring Boot 教程
- Spring Boot - 首页
- Spring Boot - 简介
- Spring Boot - 快速入门
- Spring Boot - 引导
- Spring Tool Suite
- Spring Boot - Tomcat 部署
- Spring Boot - 构建系统
- Spring Boot - 代码结构
- Spring Bean & 依赖注入
- Spring Boot - 运行器
- Spring Boot - 启动器
- Spring Boot - 应用程序属性
- Spring Boot - 配置
- Spring Boot - 注解
- Spring Boot - 日志
- 构建 RESTful Web 服务
- Spring Boot - 异常处理
- Spring Boot - 拦截器
- Spring Boot - Servlet 过滤器
- Spring Boot - Tomcat 端口号
- Spring Boot - Rest 模板
- Spring Boot - 文件处理
- Spring Boot - 服务组件
- Spring Boot - Thymeleaf
- 消费 RESTful Web 服务
- Spring Boot - CORS 支持
- Spring Boot - 国际化
- Spring Boot - 调度
- Spring Boot - 启用 HTTPS
- Spring Boot - Eureka 服务器
- 使用 Eureka 进行服务注册
- 网关代理服务器和路由
- Spring Cloud 配置服务器
- Spring Cloud 配置客户端
- Spring Boot - Actuator
- Spring Boot - Admin 服务器
- Spring Boot - Admin 客户端
- Spring Boot - 启用 Swagger2
- Spring Boot - 使用 SpringDoc OpenAPI
- Spring Boot - 创建 Docker 镜像
- 追踪微服务日志
- Spring Boot - Flyway 数据库
- Spring Boot - 发送邮件
- Spring Boot - Hystrix
- Spring Boot - WebSocket
- Spring Boot - 批处理服务
- Spring Boot - Apache Kafka
- Spring Boot - Twilio
- Spring Boot - 单元测试用例
- Rest Controller 单元测试
- Spring Boot - 数据库处理
- 保护 Web 应用程序
- Spring Boot - 带 JWT 的 OAuth2
- Spring Boot - Google Cloud Platform
- Spring Boot - Google OAuth2 登录
- Spring Boot 资源
- Spring Boot - 快速指南
- Spring Boot - 有用资源
- Spring Boot - 讨论
Spring Boot - Tomcat 部署
通过使用 Spring Boot 应用程序,我们可以创建一个 war 文件来部署到 Web 服务器。在本节中,您将学习如何创建 WAR 文件并在 Tomcat Web 服务器中部署 Spring Boot 应用程序。
Spring Boot Servlet 初始化器
传统的部署方式是使 Spring Boot 应用程序的 @SpringBootApplication 类扩展 SpringBootServletInitializer 类。Spring Boot Servlet 初始化器类文件允许您在使用 Servlet 容器启动应用程序时对其进行配置。
以下是 JAR 文件部署的 Spring Boot 应用程序类文件的代码 -
package com.tutorialspoint.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
我们需要扩展 SpringBootServletInitializer 类以支持 WAR 文件部署。以下是 Spring Boot 应用程序类文件的代码 -
package com.tutorialspoint.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.builder.SpringApplicationBuilder; import org.springframework.boot.web.servlet.support.SpringBootServletInitializer; @SpringBootApplication public class DemoApplication extends SpringBootServletInitializer { @Override protected SpringApplicationBuilder configure(SpringApplicationBuilder application) { return application.sources(DemoApplication.class); } public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
设置主类
在 Spring Boot 中,我们需要在构建文件中指定应该启动的主类。为此,您可以使用以下代码片段 -
对于 Maven,在 pom.xml 属性中添加启动类,如下所示 -
<start-class>com.tutorialspoint.demo.DemoApplication</start-class>
对于 Gradle,在 build.gradle 中添加主类名称,如下所示 -
mainClassName="com.tutorialspoint.demo.DemoApplication"
Learn Spring Boot in-depth with real-world projects through our Spring Boot certification course. Enroll and become a certified expert to boost your career.
将打包方式从 JAR 更新为 WAR
我们必须使用以下代码片段将打包方式从 JAR 更新为 WAR -
对于 Maven,在 pom.xml 中将打包方式添加为 WAR,如下所示 -
<packaging>war</packaging>
对于 Gradle,在 build.gradle 中添加应用程序插件和 war 插件,如下所示 -
apply plugin: 'war' apply plugin: 'application'
现在,让我们编写一个简单的 Rest 端点来返回字符串“Hello World from Tomcat”。要编写 Rest 端点,我们需要将 Spring Boot Web 启动器依赖项添加到我们的构建文件中。
对于 Maven,在 pom.xml 中使用以下代码添加 Spring Boot 启动器依赖项 -
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
对于 Gradle,在 build.gradle 中使用以下代码添加 Spring Boot 启动器依赖项 -
dependencies { compile('org.springframework.boot:spring-boot-starter-web') }
现在,在 Spring Boot 应用程序类文件中使用以下代码编写一个简单的 Rest 端点 -
package com.tutorialspoint.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.builder.SpringApplicationBuilder; import org.springframework.boot.web.servlet.support.SpringBootServletInitializer; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication @RestController public class DemoApplication extends SpringBootServletInitializer { @Override protected SpringApplicationBuilder configure(SpringApplicationBuilder application) { return application.sources(DemoApplication.class); } public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } @GetMapping(value = "/") public String hello() { return "Hello World from Tomcat"; } }
打包您的应用程序
现在,使用 Maven 和 Gradle 命令创建 WAR 文件以部署到 Tomcat 服务器,如下所示,用于打包您的应用程序 -
对于 Maven,使用命令 mvn package 打包您的应用程序。然后,将创建 WAR 文件,您可以在 target 目录中找到它 -
E:\dev\demo>mvn package
您将看到类似于以下的结果
[INFO] Scanning for projects... [INFO] [INFO] [1m----------------------< [0;36mcom.tutorialspoint:demo[0;1m >-----------------------[m [INFO] [1mBuilding demo 0.0.1-SNAPSHOT[m [INFO] from pom.xml [INFO] [1m--------------------------------[ war ]---------------------------------[m [INFO] [INFO] [1m--- [0;32mresources:3.3.1:resources[m [1m(default-resources)[m @ [36mdemo[0;1m ---[m [INFO] Copying 1 resource from src\main\resources to target\classes [INFO] Copying 0 resource from src\main\resources to target\classes ... [INFO] [INFO] ------------------------------------------------------- [INFO] T E S T S [INFO] ------------------------------------------------------- [INFO] Running com.tutorialspoint.demo.[1mDemoApplicationTests[m 14:41:52.687 [main] INFO org.springframework.test.context.support.AnnotationConfigContextLoaderUtils -- Could not detect default configuration classes for test class [com.tutorialspoint.demo.DemoApplicationTests]: DemoApplicationTests does not declare any static, non-private, non-final, nested classes annotated with @Configuration. 14:41:52.817 [main] INFO org.springframework.boot.test.context.SpringBootTestContextBootstrapper -- Found @SpringBootConfiguration com.tutorialspoint.demo.DemoApplication for test class com.tutorialspoint.demo.DemoApplicationTests . ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v3.3.3) 2024-09-03T14:41:53.311+05:30 INFO 7572 --- [demo] [ main] c.t.demo.DemoApplicationTests : Starting DemoApplicationTests using Java 21.0.3 with PID 7572 (started by Tutorialspoint in E:\Dev\demo) 2024-09-03T14:41:53.313+05:30 INFO 7572 --- [demo] [ main] c.t.demo.DemoApplicationTests : No active profile set, falling back to 1 default profile: "default" 2024-09-03T14:41:54.704+05:30 INFO 7572 --- [demo] [ main] c.t.demo.DemoApplicationTests : Started DemoApplicationTests in 1.722 seconds (process running for 3.248) ... [INFO] Packaging webapp [INFO] Assembling webapp [demo] in [E:\Dev\demo\target\demo-0.0.1-SNAPSHOT] [INFO] Processing war project [INFO] Copying webapp resources [E:\Dev\demo\src\main\webapp] [INFO] Building war: E:\Dev\demo\target\demo-0.0.1-SNAPSHOT.war [INFO] [INFO] [1m--- [0;32mspring-boot:3.3.3:repackage[m [1m(repackage)[m @ [36mdemo[0;1m ---[m [INFO] Replacing main artifact E:\Dev\demo\target\demo-0.0.1-SNAPSHOT.war with repackaged archive, adding nested dependencies in BOOT-INF/. [INFO] The original artifact has been renamed to E:\Dev\demo\target\demo-0.0.1-SNAPSHOT.war.original [INFO] [1m------------------------------------------------------------------------[m [INFO] [1;32mBUILD SUCCESS[m [INFO] [1m------------------------------------------------------------------------[m [INFO] Total time: 11.809 s [INFO] Finished at: 2024-09-03T14:41:59+05:30 [INFO] [1m------------------------------------------------------------------------[m
类似地,对于 Gradle,您可以使用命令 gradle clean build 打包您的应用程序。然后,将创建您的 WAR 文件,您可以在 build/libs 目录下找到它。
部署到 Tomcat
现在,运行 Tomcat 服务器,并将 WAR 文件部署到 webapps 目录下。观察此处显示的屏幕截图以更好地理解 -
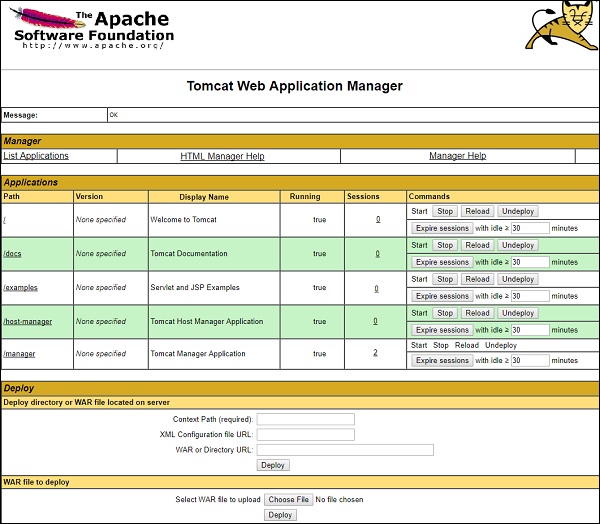

部署成功后,在 Web 浏览器中访问 URL https://127.0.0.1:8080/demo-0.0.1-SNAPSHOT/,并观察输出将如下面的屏幕截图所示 -
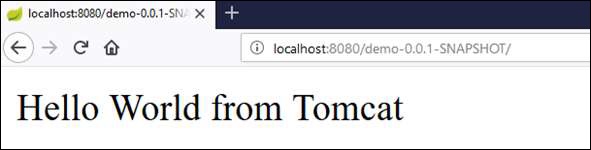
以下提供了此目的的完整代码。
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>3.3.3</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.tutorialspoint</groupId> <artifactId>demo</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>demo</name> <description>Demo project for Spring Boot</description> <url/> <licenses> <license/> </licenses> <developers> <developer/> </developers> <scm> <connection/> <developerConnection/> <tag/> <url/> </scm> <properties> <java.version>21</java.version> <start-class>com.tutorialspoint.demo.DemoApplication</start-class> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
build.gradle
buildscript { ext { springBootVersion = '3.3.3' } repositories { mavenCentral() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") } } apply plugin: 'java' apply plugin: 'eclipse' apply plugin: 'org.springframework.boot' apply plugin: 'war' apply plugin: 'application' group = 'com.tutorialspoint' version = '0.0.1-SNAPSHOT' sourceCompatibility = 21 mainClassName = "com.tutorialspoint.demo.DemoApplication" repositories { mavenCentral() } dependencies { compile('org.springframework.boot:spring-boot-starter-web') testCompile('org.springframework.boot:spring-boot-starter-test') }
以下是主 Spring Boot 应用程序类文件的代码 -
package com.tutorialspoint.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.builder.SpringApplicationBuilder; import org.springframework.boot.web.servlet.support.SpringBootServletInitializer; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @SpringBootApplication @RestController public class DemoApplication extends SpringBootServletInitializer { @Override protected SpringApplicationBuilder configure(SpringApplicationBuilder application) { return application.sources(DemoApplication.class); } public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } @GetMapping(value = "/") public String hello() { return "Hello World from Tomcat"; } }