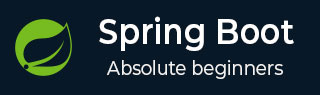
- Spring Boot 教程
- Spring Boot - 首页
- Spring Boot - 简介
- Spring Boot - 快速入门
- Spring Boot - 启动引导
- Spring Tool Suite
- Spring Boot - Tomcat 部署
- Spring Boot - 构建系统
- Spring Boot - 代码结构
- Spring Bean & 依赖注入
- Spring Boot - 运行器
- Spring Boot - 启动器
- Spring Boot - 应用属性
- Spring Boot - 配置
- Spring Boot - 注解
- Spring Boot - 日志记录
- 构建 RESTful Web 服务
- Spring Boot - 异常处理
- Spring Boot - 拦截器
- Spring Boot - Servlet 过滤器
- Spring Boot - Tomcat 端口号
- Spring Boot - Rest Template
- Spring Boot - 文件处理
- Spring Boot - 服务组件
- Spring Boot - Thymeleaf
- 使用 RESTful Web 服务
- Spring Boot - CORS 支持
- Spring Boot - 国际化
- Spring Boot - 定时任务
- Spring Boot - 启用 HTTPS
- Spring Boot - Eureka 服务器
- 使用 Eureka 进行服务注册
- 网关代理服务器和路由
- Spring Cloud 配置服务器
- Spring Cloud 配置客户端
- Spring Boot - Actuator
- Spring Boot - Admin 服务器
- Spring Boot - Admin 客户端
- Spring Boot - 启用 Swagger2
- Spring Boot - 使用 SpringDoc OpenAPI
- Spring Boot - 创建 Docker 镜像
- 追踪微服务日志
- Spring Boot - Flyway 数据库
- Spring Boot - 发送邮件
- Spring Boot - Hystrix
- Spring Boot - Web Socket
- Spring Boot - 批处理服务
- Spring Boot - Apache Kafka
- Spring Boot - Twilio
- Spring Boot - 单元测试用例
- Rest Controller 单元测试
- Spring Boot - 数据库处理
- 保护 Web 应用程序
- Spring Boot - 带 JWT 的 OAuth2
- Spring Boot - Google Cloud Platform
- Spring Boot - Google OAuth2 登录
- Spring Boot 资源
- Spring Boot - 快速指南
- Spring Boot - 有用资源
- Spring Boot - 讨论
Spring Boot - 使用 SpringDoc OpenAPI
springdoc-openapi 是一个开源项目,用于为基于 Spring Boot 的项目生成 API 文档。它在运行时检查应用程序的 API 语义,这些语义基于 Spring 配置、类结构和注解。它支持以下 API。
OpenAPI 3
Spring Boot 3
JSR-303 规范,包括 @NotNull、@Min、@Max 和 @Size。
Swagger-ui
OAuth 2
GraalVM 原生镜像
在这个示例中,我们正在启用 Swagger UI。要在 Spring Boot 应用程序中启用 Swagger,需要在构建配置文件中添加以下依赖项。
<dependency> <groupId>org.springdoc</groupId> <artifactId>springdoc-openapi-starter-webmvc-ui</artifactId> <version>2.6.0</version> </dependency>
对于 Gradle 用户,请在您的 build.gradle 文件中添加以下依赖项。
compile group: 'org.springdoc', name: 'springdoc-openapi-starter-webmvc-ui', version: '2.6.0'
就是这样。不需要其他配置。我们可以使用以下 URL 检查 JSON 格式的 API 规范:
http://server:port/<context-path>/v3/api-docs
Swagger UI 可通过以下 URL 访问:
http://server:port/<context-path>/swagger-ui/index.html
让我们将 OpenAPI 文档支持添加到我们在Spring Boot - 使用 RESTful Web 服务章节中讨论的 REST API 示例中。
完整的构建配置文件如下所示:
Maven – pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>3.3.3</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.tutorialspoint</groupId> <artifactId>demo</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>demo</name> <description>Demo project for Spring Boot</description> <url/> <licenses> <license/> </licenses> <developers> <developer/> </developers> <scm> <connection/> <developerConnection/> <tag/> <url/> </scm> <properties> <java.version>21</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springdoc</groupId> <artifactId>springdoc-openapi-starter-webmvc-ui</artifactId> <version>2.6.0</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Gradle – build.gradle
buildscript { ext { springBootVersion = '3.3.3' } repositories { mavenCentral() } dependencies { classpath("org.springframework.boot:spring-boot-gradle-plugin:${springBootVersion}") } } apply plugin: 'java' apply plugin: 'eclipse' apply plugin: 'org.springframework.boot' group = 'com.tutorialspoint' version = '0.0.1-SNAPSHOT' sourceCompatibility = 1.8 repositories { mavenCentral() } dependencies { compile('org.springframework.boot:spring-boot-starter-web') testCompile('org.springframework.boot:spring-boot-starter-test') compile group: 'org.springdoc', name: 'springdoc-openapi-starter-webmvc-ui', version: '2.6.0' }
编译和执行
您可以创建一个可执行的 JAR 文件,并使用以下 Maven 或 Gradle 命令运行 Spring Boot 应用程序,如下所示:
对于 Maven,使用以下命令:
mvn clean install
“BUILD SUCCESS”之后,您可以在 target 目录下找到 JAR 文件。
对于 Gradle,使用以下命令:
gradle clean build
“BUILD SUCCESSFUL”之后,您可以在 build/libs 目录下找到 JAR 文件。
您可以使用以下命令运行 JAR 文件:
java –jar <JARFILE>
这将在 Tomcat 端口 8080 上启动应用程序,如下所示:
. ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ [32m :: Spring Boot :: [39m [2m (v3.3.3)[0;39m [2024-09-17T17:37:50Z] [org.springframework.boot.StartupInfoLogger] [main] [50] [INFO ] Starting DemoApplication using Java 21.0.3 with PID 7760 (E:\Dev\demo\target\classes started by Tutorialspoint in E:\Dev\demo) [2024-09-17T17:37:50Z] [org.springframework.boot.SpringApplication] [main] [654] [INFO ] No active profile set, falling back to 1 default profile: "default" [2024-09-17T17:37:51Z] [org.springframework.boot.web.embedded.tomcat.TomcatWebServer] [main] [111] [INFO ] Tomcat initialized with port 8080 (http) [2024-09-17T17:37:51Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Initializing ProtocolHandler ["http-nio-8080"] [2024-09-17T17:37:51Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Starting service [Tomcat] [2024-09-17T17:37:51Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Starting Servlet engine: [Apache Tomcat/10.1.28] [2024-09-17T17:37:51Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Initializing Spring embedded WebApplicationContext [2024-09-17T17:37:51Z] [org.springframework.boot.web.servlet.context.ServletWebServerApplicationContext] [main] [296] [INFO ] Root WebApplicationContext: initialization completed in 1106 ms [2024-09-17T17:37:51Z] [org.springframework.boot.autoconfigure.web.servlet.WelcomePageHandlerMapping] [main] [59] [INFO ] Adding welcome page template: index [2024-09-17T17:37:52Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Starting ProtocolHandler ["http-nio-8080"] [2024-09-17T17:37:52Z] [org.springframework.boot.web.embedded.tomcat.TomcatWebServer] [main] [243] [INFO ] Tomcat started on port 8080 (http) with context path '/' [2024-09-17T17:37:52Z] [org.springframework.boot.web.embedded.tomcat.TomcatWebServer] [main] [111] [INFO ] Tomcat initialized with port 9000 (http) [2024-09-17T17:37:52Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Initializing ProtocolHandler ["http-nio-9000"] [2024-09-17T17:37:52Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Starting service [Tomcat] [2024-09-17T17:37:52Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Starting Servlet engine: [Apache Tomcat/10.1.28] [2024-09-17T17:37:52Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Initializing Spring embedded WebApplicationContext [2024-09-17T17:37:52Z] [org.springframework.boot.web.servlet.context.ServletWebServerApplicationContext] [main] [296] [INFO ] Root WebApplicationContext: initialization completed in 80 ms [2024-09-17T17:37:52Z] [org.springframework.boot.actuate.endpoint.web.EndpointLinksResolver] [main] [60] [INFO ] Exposing 1 endpoint beneath base path '/actuator' [2024-09-17T17:37:52Z] [org.apache.juli.logging.DirectJDKLog] [main] [173] [INFO ] Starting ProtocolHandler ["http-nio-9000"] [2024-09-17T17:37:52Z] [org.springframework.boot.web.embedded.tomcat.TomcatWebServer] [main] [243] [INFO ] Tomcat started on port 9000 (http) with context path '/' [2024-09-17T17:37:52Z] [org.springframework.boot.StartupInfoLogger] [main] [56] [INFO ] Started DemoApplication in 2.378 seconds (process running for 3.241) [2024-09-17T17:37:53Z] [org.apache.juli.logging.DirectJDKLog] [RMI TCP Connection(4)-127.0.0.1] [173] [INFO ] Initializing Spring DispatcherServlet 'dispatcherServlet' [2024-09-17T17:37:53Z] [org.springframework.web.servlet.FrameworkServlet] [RMI TCP Connection(4)-127.0.0.1] [532] [INFO ] Initializing Servlet 'dispatcherServlet' [2024-09-17T17:37:53Z] [org.springframework.web.servlet.FrameworkServlet] [RMI TCP Connection(4)-127.0.0.1] [554] [INFO ] Completed initialization in 1 ms Remote Host:0:0:0:0:0:0:0:1 Remote Address:0:0:0:0:0:0:0:1 Pre Handle method is Calling [2024-09-17T17:38:43Z] [org.springdoc.api.AbstractOpenApiResource] [http-nio-8080-exec-1] [390] [INFO ] Init duration for springdoc-openapi is: 148 ms
输出
现在,在浏览器中点击以下 URL 查看 JSON 格式的 API:
https://127.0.0.1:8080/v3/api-docs
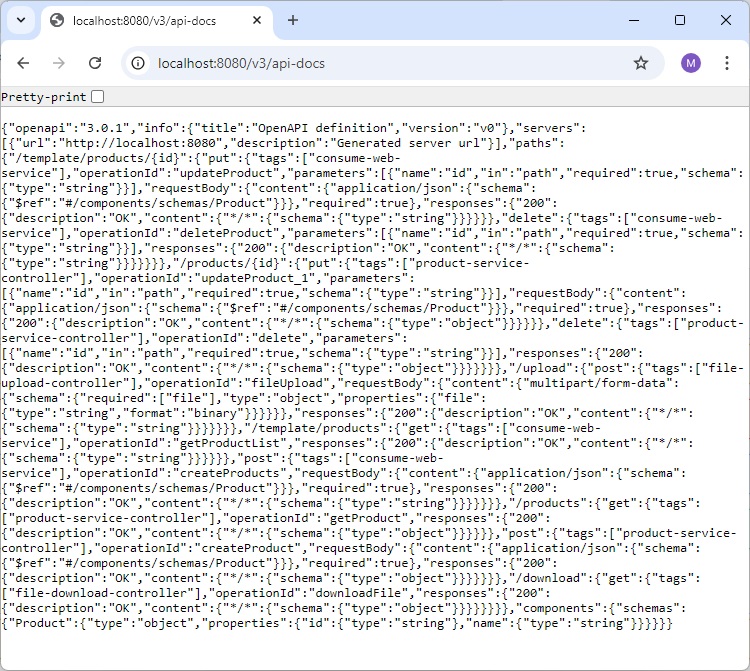
现在,在浏览器中点击以下 URL 查看 Swagger UI 中的 API 文档:
https://127.0.0.1:8080/swagger-ui/index.html
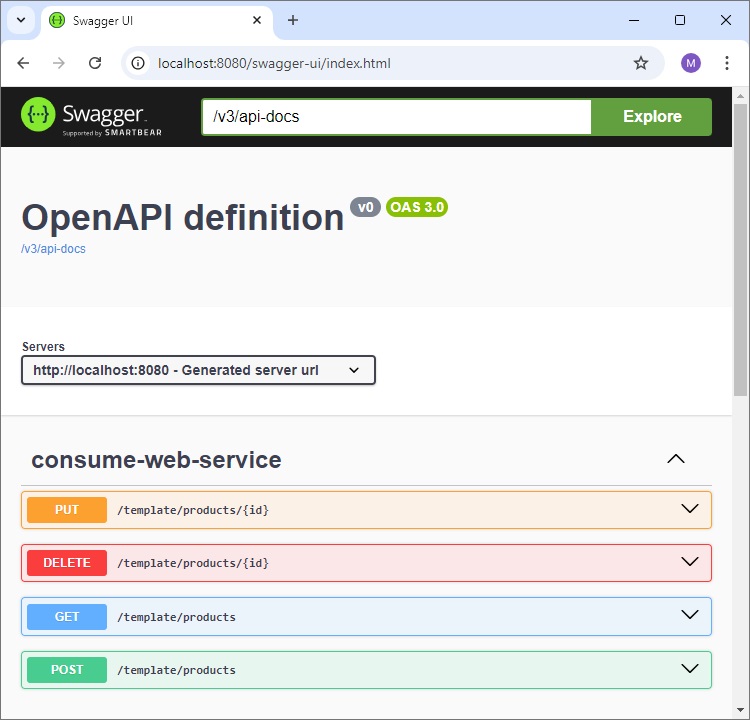