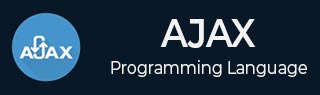
- AJAX 教程
- AJAX - 首页
- AJAX - 什么是 AJAX?
- AJAX - 历史
- AJAX - 动态网站与静态网站
- AJAX - 技术
- AJAX - 动作
- AJAX - XMLHttpRequest
- AJAX - 发送请求
- AJAX - 请求类型
- AJAX - 处理响应
- AJAX - 处理二进制数据
- AJAX - 提交表单
- AJAX - 文件上传
- AJAX - FormData 对象
- AJAX - 发送 POST 请求
- AJAX - 发送 PUT 请求
- AJAX - 发送 JSON 数据
- AJAX - 发送数据对象
- AJAX - 监控进度
- AJAX - 状态码
- AJAX - 应用
- AJAX - 浏览器兼容性
- AJAX - 示例
- AJAX - 浏览器支持
- AJAX - XMLHttpRequest
- AJAX - 数据库操作
- AJAX - 安全性
- AJAX - 问题
- Fetch API 基础
- Fetch API - 基础
- Fetch API 与 XMLHttpRequest
- Fetch API - 浏览器兼容性
- Fetch API - 头部信息
- Fetch API - 请求
- Fetch API - 响应
- Fetch API - 主体数据
- Fetch API - 凭据
- Fetch API - 发送 GET 请求
- Fetch API - 发送 POST 请求
- Fetch API - 发送 PUT 请求
- Fetch API - 发送 JSON 数据
- Fetch API - 发送数据对象
- Fetch API - 自定义请求对象
- Fetch API - 上传文件
- Fetch API - 处理二进制数据
- Fetch API - 状态码
- Stream API 基础
- Stream API - 基础
- Stream API - 可读流
- Stream API - 可写流
- Stream API - 变换流
- Stream API - 请求对象
- Stream API - 响应主体
- Stream API - 错误处理
- AJAX 有用资源
- AJAX - 快速指南
- AJAX - 有用资源
- AJAX - 讨论
AJAX - 文件上传
AJAX 提供了一种灵活的方式来创建 HTTP 请求,该请求将文件上传到服务器。我们可以使用 FormData 对象在请求中发送单个或多个文件。让我们通过以下示例来讨论这个概念:
示例 - 上传单个文件
在下面的示例中,我们将使用 XMLHttpRequest 上传单个文件。为此,我们首先创建一个简单的表单,其中包含一个文件上传按钮和一个提交按钮。现在,我们编写 JavaScript 代码,在其中获取表单元素并创建一个在点击上传文件按钮时触发的事件。在这个事件中,我们将上传的文件添加到 FormData 对象中,然后创建一个 XMLHttpRequest 对象,该对象将使用 FormData 对象将文件发送到服务器并处理服务器返回的响应。
<!DOCTYPE html> <html> <body> <!-- Creating a form to upload a file--> <form id = "myForm"> <input type="file" id="file"><br><br> <button type="submit">Upload File</button> </form> <script> document.getElementById('myForm').addEventListener('submit', function(x){ // Prevent from page refreshing x.preventDefault(); // Select the file from the system // Here we are going to upload one file at a time const myFile = document.getElementById('file').files[0]; // Create a FormData to store the file const myData = new FormData(); // Add file in the FormData myData.append("newFiles", myFile); // Creating XMLHttpRequest object var myhttp = new XMLHttpRequest(); // Callback function to handle the response myhttp.onreadystatechange = function(){ if (myhttp.readyState == 4 && myhttp.status == 200) { console.log("File uploaded Successfully") } }; // Open the connection with the web server myhttp.open("POST", "https://httpbin.org/post", true); // Setting headers myhttp.setRequestHeader("Content-Type", "multipart/form-data"); // Sending file to the server myhttp.send(myData); }) </script> </body> </html>
输出
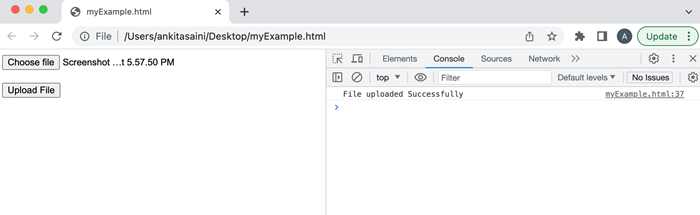
示例 - 上传多个文件
在下面的示例中,我们将使用 XMLHttpRequest 上传多个文件。在这里,我们使用文件类型的属性在 DOM 中选择系统中的两个文件。然后我们将输入文件添加到数组中。然后我们创建一个 FormData 对象并将输入文件添加到该对象。然后我们创建一个 XMLHttpRequest 对象,该对象将使用 FormData 对象将文件发送到服务器并处理服务器返回的响应。
<!DOCTYPE html> <html> <body> <!-- Creating a form to upload multiple files--> <h2> Uploading Multiple files</h2> <input type="file"> <input type="file"> <button>Submit</button> <script> const myButton = document.querySelector('button'); myButton.addEventListener('click', () => { // Get all the input files in DOM with attribute type "file": const inputFiles = document.querySelectorAll('input[type="file"]'); // Add input files in the array const myfiles = []; inputFiles.forEach((inputFiles) => myfiles.push(inputFiles.files[0])); // Creating a FormData const myformdata = new FormData(); // Append files in the FormData object for (const [index, file] of myfiles.entries()){ // It contained reference name, file, set file name myformdata.append(`file${index}`, file, file.name); } // Creating an XMLHttpRequest object var myhttp = new XMLHttpRequest(); // Callback function // To handle the response myhttp.onreadystatechange = function(){ if (myhttp.readyState == 4 && myhttp.status == 200) { console.log("File uploaded Successfully") } }; // Open the connection with the web server myhttp.open("POST", "https://httpbin.org/post", true); // Setting headers myhttp.setRequestHeader("Content-Type", "multipart/form-data"); // Sending file to the server myhttp.send(myformdata); }) </script> </body> </html>
输出
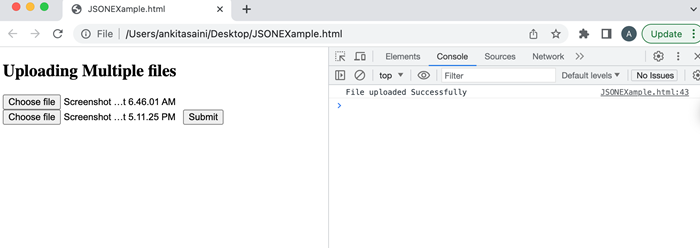
结论
这就是我们如何使用 XMLHttpRequest 将文件上传到给定 URL 的方法。在这里,我们可以上传任何类型的文件,例如 jpg、pdf、word 等,并且可以一次上传一个文件或多个文件。在下一篇文章中,我们将学习如何使用 XMLHttpRequest 创建 FormData 对象。
广告