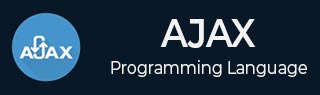
- AJAX 教程
- AJAX - 首页
- AJAX - 什么是 AJAX?
- AJAX - 历史
- AJAX - 动态网站与静态网站
- AJAX - 技术
- AJAX - 操作
- AJAX - XMLHttpRequest
- AJAX - 发送请求
- AJAX - 请求类型
- AJAX - 处理响应
- AJAX - 处理二进制数据
- AJAX - 提交表单
- AJAX - 文件上传
- AJAX - FormData 对象
- AJAX - 发送 POST 请求
- AJAX - 发送 PUT 请求
- AJAX - 发送 JSON 数据
- AJAX - 发送数据对象
- AJAX - 监控进度
- AJAX - 状态码
- AJAX - 应用
- AJAX - 浏览器兼容性
- AJAX - 示例
- AJAX - 浏览器支持
- AJAX - XMLHttpRequest
- AJAX - 数据库操作
- AJAX - 安全性
- AJAX - 问题
- Fetch API 基础
- Fetch API - 基础
- Fetch API 与 XMLHttpRequest
- Fetch API - 浏览器兼容性
- Fetch API - 头信息
- Fetch API - 请求
- Fetch API - 响应
- Fetch API - 主体数据
- Fetch API - 凭据
- Fetch API - 发送 GET 请求
- Fetch API - 发送 POST 请求
- Fetch API - 发送 PUT 请求
- Fetch API - 发送 JSON 数据
- Fetch API - 发送数据对象
- Fetch API - 自定义请求对象
- Fetch API - 上传文件
- Fetch API - 处理二进制数据
- Fetch API - 状态码
- Stream API 基础
- Stream API - 基础
- Stream API - 可读流
- Stream API - 可写流
- Stream API - 变换流
- Stream API - 请求对象
- Stream API - 响应主体
- Stream API - 错误处理
- AJAX 有用资源
- AJAX - 快速指南
- AJAX - 有用资源
- AJAX - 讨论
Fetch API - 上传文件
Fetch API 提供了一种灵活的方式来创建 HTTP 请求,该请求将文件上传到服务器。我们可以使用 fetch() 函数以及 FormData 对象在请求中发送单个或多个文件。让我们借助以下示例来讨论这个概念:
示例 - 上传单个文件
在以下程序中,我们使用 Fetch API 每次上传一个文件。这里我们使用 FormData 对象存储文件,然后使用 fetch() 函数将其发送到给定的 URL,包括 POST 请求方法和 FormData 对象。在将请求发送到服务器后,我们现在使用 then() 函数来处理响应。如果遇到错误,则 catch() 函数将处理该错误。
<!DOCTYPE html> <html> <body> <!-- Creating a form to upload a file--> <form id = "myForm"> <input type="file" id="file"><br><br> <button type="submit">Upload File</button> </form> <script> document.getElementById('myForm').addEventListener('submit', function(x){ // Prevent from page refreshing x.preventDefault(); // Select the file from the system // Here we are going to upload one file at a time const myFile = document.getElementById('file').files[0]; // Create a FormData to store the file const myData = new FormData(); // Add file in the FormData myData.append("newFiles", myFile); // Send the file to the given URL fetch("https://httpbin.org/post", { // POST request with Fetch API method: "POST", // Adding FormData to the request body: myData }) // Converting the response in JSON // using response.json() function .then(response => response.json()) .then(finalData => { // Handling the response console.log("File has been uploaded successfully"); }) .catch(err=>{ // Handling the error console.log("Error Found:", err) }); }) </script> </body> </html>
输出
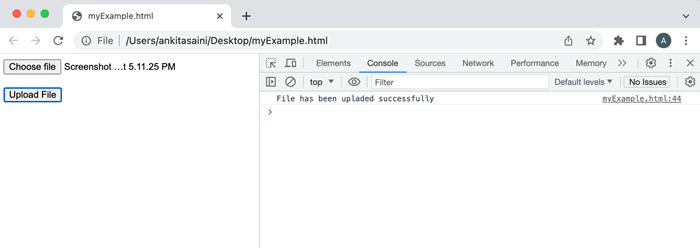
示例 - 为单个输入上传多个文件
在以下程序中,我们将使用 Fetch API 从单个输入上传多个文件。这里我们在 <input> 标签中添加了一个 "multiple" 属性来添加多个文件。然后我们使用 FormData 对象存储多个文件,然后使用 fetch() 函数将其发送到给定的 URL,包括 POST 请求方法和 FormData 对象。在将请求发送到服务器后,我们现在使用 then() 函数来处理响应。如果遇到错误,则 catch() 函数将处理该错误。
<!DOCTYPE html> <html> <body> <!-- Creating a form to upload a file--> <h2> Uploading Multiple files</h2> <form id = "myForm"> <p>Maximum number of files should be 2</p> <input type="file" id="file" multiple><br><br> <button type="submit">Upload File</button> </form> <script> document.getElementById('myForm').addEventListener('submit', function(x){ // Prevent from page refreshing x.preventDefault(); // Select the file from the system // Here we are going to upload multiple files at a time const myFile = document.getElementById('file').files[0]; // Create a FormData to store the file const myData = new FormData(); // Add file in the FormData myData.append("newFiles", myFile); // Send the file to the given URL fetch("https://httpbin.org/post", { // POST request with Fetch API method: "POST", // Adding FormData to the request body: myData }) // Converting the response in JSON // using response.json() function .then(response => response.json()) .then(finalData => { // Handling the response console.log("Files has been uploaded successfully"); }) .catch(err=>{ // Handling the error console.log("Error Found:", err) }); }) </script> </body> </html>
输出
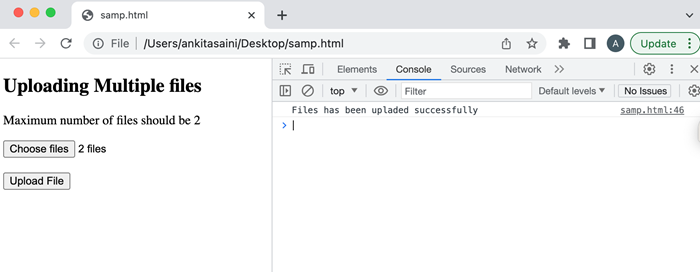
示例 - 上传多个文件
在以下程序中,我们将使用 Fetch API 上传多个文件。这里我们在 DOM 中使用文件类型的属性从系统中选择两个文件。然后我们将输入文件添加到数组中。然后我们创建一个 FormData 对象并将输入文件附加到该对象。然后我们使用 fetch() 函数将其发送到给定的 URL,包括 POST 请求方法和 FormData 对象。在将请求发送到服务器后,我们现在使用 then() 函数来处理响应。如果遇到错误,则 catch() 函数将处理该错误。
<!DOCTYPE html> <html> <body> <!-- Creating a form to upload multiple files--> <h2> Uploading Multiple files</h2> <input type="file"> <input type="file"> <button>Submit</button> <script> const myButton = document.querySelector('button'); myButton.addEventListener('click', () => { // Get all the input files in DOM with attribute type "file": const inputFiles = document.querySelectorAll('input[type="file"]'); // Add input files in the array const myfiles = []; inputFiles.forEach((inputFiles) => myfiles.push(inputFiles.files[0])); // Creating a FormData const myformdata = new FormData(); // Append files in the FormData object for (const [index, file] of myfiles.entries()){ // It contained reference name, file, set file name myformdata.append(`file${index}`, file, file.name); } // Upload the FormData object fetch('https://httpbin.org/post', { method: "POST", body: myformdata, }) // Handle the response .then(response => response.json()) .then(response => console.log(response)) // Handle the error .catch(err => console.log("Error Found:", err)) }) </script> </body> </html>
输出
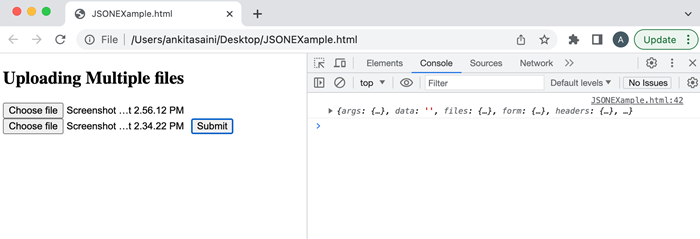
结论
因此,这就是我们如何使用 fetch() API 将文件上传到给定 URL 的方法。在这里,我们可以上传任何类型的文件,例如 jpg、pdf、word 等,并可以一次上传任意数量的文件,例如一次上传一个文件或一次上传多个文件。现在,在下一篇文章中,我们将学习 Fetch API 如何处理响应。