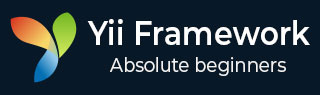
- Yii 教程
- Yii - 首页
- Yii - 概述
- Yii - 安装
- Yii - 创建页面
- Yii - 应用结构
- Yii - 入口脚本
- Yii - 控制器
- Yii - 使用控制器
- Yii - 使用操作
- Yii - 模型
- Yii - 小部件
- Yii - 模块
- Yii - 视图
- Yii - 布局
- Yii - 资源
- Yii - 资源转换
- Yii - 扩展
- Yii - 创建扩展
- Yii - HTTP 请求
- Yii - 响应
- Yii - URL 格式
- Yii - URL 路由
- Yii - URL 规则
- Yii - HTML 表单
- Yii - 验证
- Yii - 即时验证
- Yii - AJAX 验证
- Yii - 会话
- Yii - 使用 Flash 数据
- Yii - Cookie
- Yii - 使用 Cookie
- Yii - 文件上传
- Yii - 格式化
- Yii - 分页
- Yii - 排序
- Yii - 属性
- Yii - 数据提供者
- Yii - 数据小部件
- Yii - ListView 小部件
- Yii - GridView 小部件
- Yii - 事件
- Yii - 创建事件
- Yii - 行为
- Yii - 创建行为
- Yii - 配置
- Yii - 依赖注入
- Yii - 数据库访问
- Yii - 数据访问对象
- Yii - 查询构建器
- Yii - 活动记录
- Yii - 数据库迁移
- Yii - 主题
- Yii - RESTful API
- Yii - RESTful API 实践
- Yii - 字段
- Yii - 测试
- Yii - 缓存
- Yii - 碎片缓存
- Yii - 别名
- Yii - 日志
- Yii - 错误处理
- Yii - 身份验证
- Yii - 授权
- Yii - 本地化
- Yii - Gii
- Gii – 创建模型
- Gii – 生成控制器
- Gii – 生成模块
- Yii 有用资源
- Yii - 快速指南
- Yii - 有用资源
- Yii - 讨论
Yii - 创建事件
本章将介绍如何在 Yii 中创建事件。为了演示事件的实际应用,我们需要一些数据。
准备数据库
步骤 1 - 创建一个新的数据库。数据库可以通过以下两种方式创建。
在终端运行 mysql -u root –p
使用 CREATE DATABASE helloworld CHARACTER SET utf8 COLLATE utf8_general_ci; 创建一个新的数据库。
步骤 2 - 在 config/db.php 文件中配置数据库连接。以下配置适用于当前使用的系统。
<?php return [ 'class' => 'yii\db\Connection', 'dsn' => 'mysql:host=localhost;dbname=helloworld', 'username' => 'vladimir', 'password' => '12345', 'charset' => 'utf8', ]; ?>
步骤 3 - 在根文件夹内运行 ./yii migrate/create test_table。此命令将创建一个数据库迁移文件来管理我们的数据库。迁移文件应该出现在项目根目录的 migrations 文件夹中。
步骤 4 - 修改迁移文件(在本例中为 m160106_163154_test_table.php)。
<?php use yii\db\Schema; use yii\db\Migration; class m160106_163154_test_table extends Migration { public function safeUp() { $this->createTable("user", [ "id" => Schema::TYPE_PK, "name" => Schema::TYPE_STRING, "email" => Schema::TYPE_STRING, ]); $this->batchInsert("user", ["name", "email"], [ ["User1", "user1@gmail.com"], ["User2", "user2@gmail.com"], ["User3", "user3@gmail.com"], ["User4", "user4@gmail.com"], ["User5", "user5@gmail.com"], ["User6", "user6@gmail.com"], ["User7", "user7@gmail.com"], ["User8", "user8@gmail.com"], ["User9", "user9@gmail.com"], ["User10", "user10@gmail.com"], ["User11", "user11@gmail.com"], ]); } public function safeDown() { $this->dropTable('user'); } } ?>
上述迁移创建了一个名为 user 的表,包含 id、name 和 email 字段。它还添加了一些演示用户。
步骤 5 - 在项目根目录内运行 ./yii migrate 将迁移应用到数据库。
步骤 6 - 现在,我们需要为我们的 user 表创建一个模型。为简便起见,我们将使用 Gii 代码生成工具。打开此 url: http://localhost:8080/index.php?r=gii。然后,点击“Model generator”下的“Start”按钮。填写表名(“user”)和模型类名(“MyUser”),点击“Preview”按钮,最后点击“Generate”按钮。
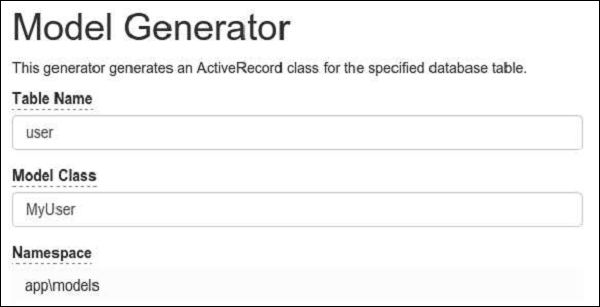
MyUser 模型应该出现在 models 目录中。
创建事件
假设我们希望每当有新用户注册到我们的网站时,向管理员发送一封电子邮件。
步骤 1 - 修改 models/MyUser.php 文件。
<?php namespace app\models; use Yii; /** * This is the model class for table "user". * * @property integer $id * @property string $name * @property string $email */ class MyUser extends \yii\db\ActiveRecord { const EVENT_NEW_USER = 'new-user'; public function init() { // first parameter is the name of the event and second is the handler. $this->on(self::EVENT_NEW_USER, [$this, 'sendMailToAdmin']); } /** * @inheritdoc */ public static function tableName() { return 'user'; } /** * @inheritdoc */ public function rules() { return [ [['name', 'email'], 'string', 'max' => 255] ]; } /** * @inheritdoc */ public function attributeLabels() { return [ 'id' => 'ID', 'name' => 'Name', 'email' => 'Email', ]; } public function sendMailToAdmin($event) { echo 'mail sent to admin using the event'; } } ?>
在上面的代码中,我们定义了一个“new-user”事件。然后,在 init() 方法中,我们将 sendMailToAdmin 函数附加到“new-user”事件。现在,我们需要触发此事件。
步骤 2 - 在 SiteController 中创建一个名为 actionTestEvent 的方法。
public function actionTestEvent() { $model = new MyUser(); $model->name = "John"; $model->email = "john@gmail.com"; if($model->save()) { $model->trigger(MyUser::EVENT_NEW_USER); } }
在上面的代码中,我们创建了一个新用户并触发了“new-user”事件。
步骤 3 - 现在输入 http://localhost:8080/index.php?r=site/test-event,您将看到以下内容。
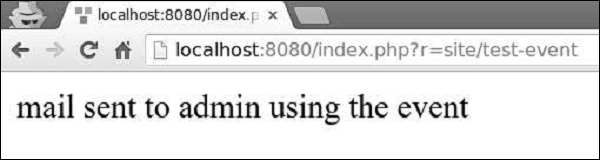