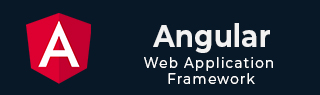
- Angular 教程
- Angular - 首页
- Angular - 概述
- Angular - 特性
- Angular - 优点与缺点
- Angular 基础
- Angular - 环境搭建
- Angular - 第一个应用
- Angular - MVC 架构
- Angular 组件
- Angular - 组件
- Angular - 组件生命周期
- Angular - 视图封装
- Angular - 组件交互
- Angular - 组件样式
- Angular - 嵌套组件
- Angular - 内容投影
- Angular - 动态组件
- Angular - 元素
- Angular 模板
- Angular - 模板
- Angular - 文本插值
- Angular - 模板语句
- Angular - 模板中的变量
- Angular - SVG 作为模板
- Angular 绑定
- Angular - 绑定及其类型
- Angular - 数据绑定
- Angular - 事件绑定
- Angular - 属性绑定
- Angular - 属性绑定
- Angular - 类和样式绑定
- Angular 指令
- Angular - 指令
- Angular - 内置指令
- Angular 管道
- Angular - 管道
- Angular - 使用管道转换数据
- Angular 依赖注入
- Angular - 依赖注入
- Angular HTTP 客户端编程
- Angular - 服务
- Angular - HTTP 客户端
- Angular - 请求
- Angular - 响应
- Angular - GET 请求
- Angular - PUT 请求
- Angular - DELETE 请求
- Angular - JSON-P
- Angular - 使用 HTTP 进行 CRUD 操作
- Angular 路由
- Angular - 路由
- Angular - 导航
- Angular - Angular Material
- Angular 动画
- Angular - 动画
- Angular 表单
- Angular - 表单
- Angular - 表单验证
- Angular Service Workers 和 PWA
- Angular - Service Workers 和 PWA
- Angular 测试
- Angular - 测试概述
- Angular NgModules
- Angular - 模块简介
- Angular 高级
- Angular - 认证与授权
- Angular - 国际化
- Angular - 可访问性
- Angular - Web Workers
- Angular - 服务器端渲染
- Angular - Ivy 编译器
- Angular - 使用 Bazel 构建
- Angular - 向后兼容性
- Angular - 响应式编程
- Angular - 在指令和组件之间共享数据
- Angular 工具
- Angular - CLI
- Angular 杂项
- Angular - 第三方控件
- Angular - 配置
- Angular - 显示数据
- Angular - 装饰器和元数据
- Angular - 基本示例
- Angular - 错误处理
- Angular - 测试和构建项目
- Angular - 生命周期钩子
- Angular - 用户输入
- Angular - 新特性?
- Angular 有用资源
- Angular - 快速指南
- Angular - 有用资源
- Angular - 讨论
Angular - 动画
动画使 Web 应用焕然一新,并提供了丰富的用户交互体验。在 HTML 中,动画基本上是在特定时间段内将 HTML 元素从一种 CSS 样式转换为另一种 CSS 样式的过程。例如,可以通过更改图像元素的宽度和高度来放大它。
如果在一段时间内(例如 10 秒)以步骤方式将图像的宽度和高度从初始值更改为最终值,则会产生动画效果。因此,动画的范围取决于 CSS 为设置 HTML 元素样式提供的功能/属性。
Angular 提供了一个单独的模块 **BrowserAnimationModule** 来执行动画。**BrowserAnimationModule** 提供了一种简单明了的方法来执行动画。
配置动画模块
让我们在本节中学习如何在应用中配置动画模块。
请按照以下步骤在应用中配置动画模块 **BrowserAnimationModule** 。
在 AppModule 中导入 **BrowserAnimationModule**。
import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; @NgModule({ imports: [ BrowserModule, BrowserAnimationsModule ], declarations: [ ], bootstrap: [ ] }) export class AppModule { }
在相关的组件中导入动画函数。
import { state, style, transition, animate, trigger } from '@angular/animations'
在相关的组件中添加 **animations** 元数据属性。
@Component({ animations: [ // animation functionality goes here ] }) export class MyAnimationComponent
概念
在 Angular 中,我们需要理解五个核心概念及其与动画的关系。
状态
状态指的是组件的特定状态。一个组件可以有多个定义的状态。状态是使用 state() 方法创建的。state() 方法有两个参数。
**name:** 状态的唯一名称。
**style:** 使用 style() 方法定义的状态样式。
animations: [ ... state('start', style( { width: 200px; } )) ... ]
这里,**start** 是状态的名称。
样式
**样式** 指的是在特定状态下应用的 CSS 样式。style() 方法用于设置组件特定状态的样式。它使用 CSS 属性,并且可以有多个项目。
animations: [ ... state('start', style( { width: 200px; opacity: 1 } )) ... ]
这里,**start** 状态定义了两个 CSS 属性,**width** 值为 200px,**opacity** 值为 1。
过渡
**过渡** 指的是从一个状态到另一个状态的转换。动画可以有多个过渡。每个过渡都是使用 transition() 函数定义的。transition() 接受两个参数。
指定两个过渡状态之间的方向。例如,**start => end** 表示初始状态为 **start**,最终状态为 **end**。实际上,它是一个具有丰富功能的表达式。
使用 **animate()** 函数指定动画细节。
animations: [ ... transition('start => end', [ animate('1s') ]) ... ]
这里,**transition()** 函数定义了从 start 状态到 end 状态的过渡,并在 **animate()** 方法中定义了动画。
动画
动画定义了从一个状态到另一个状态的转换方式。**animation()** 函数用于设置动画细节。**animate()** 接受一个以以下表达式形式表示的参数:
duration delay easing
**duration:** 指的是过渡的持续时间。表示为 1s、100ms 等。
**delay:** 指的是开始过渡的延迟时间。表示方式与 *duration* 相同。
**easing:** 指的是如何在给定的持续时间内加速/减速过渡。
触发器
每个动画都需要一个触发器来启动动画。trigger() 方法用于在一个地方设置所有动画信息(如状态、样式、过渡和动画),并为其提供一个唯一名称。唯一名称随后用于触发动画。
animations: [ trigger('enlarge', [ state('start', style({ height: '200px', })), state('end', style({ height: '500px', })), transition('start => end', [ animate('1s') ]), transition('end => start', [ animate('0.5s') ]) ]), ]
这里,**enlarge** 是给定动画的唯一名称。它有两个状态和相关的样式。它有两个过渡,一个是从 start 到 end,另一个是从 end 到 start。End 到 start 状态执行动画的反向操作。
**触发器** 可以附加到元素上,如下所示:
<div [@triggerName]="expression">...</div>;
例如,
<img [@enlarge]="isEnlarge ? 'end' : 'start'">...</img>;
这里,
**@enlarge:** 触发器设置为 image 标签并附加到表达式上。
如果 **isEnlarge** 的值更改为 true,则将设置 **end** 状态,并触发 **start => end** 过渡。
如果 **isEnlarge** 的值更改为 false,则将设置 **start** 状态,并触发 **end => start** 过渡。
简单的动画示例
让我们编写一个新的 Angular 应用,通过使用动画效果放大图像来更好地理解动画概念。
打开命令提示符并创建一个新的 Angular 应用。
cd /go/to/workspace ng new animation-app cd animation-app
在 **AppModule** (src/app/app.module.ts) 中配置 **BrowserAnimationModule** 。
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core' import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, BrowserAnimationsModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
打开 **AppComponent (src/app/app.component.ts)** 并导入必要的动画函数。
import { state, style, transition, animate, trigger } from '@angular/animations';
添加动画功能,这将在放大/缩小图像期间为图像设置动画。
@Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], animations: [ trigger('enlarge', [ state('start', style({ height: '150px' })), state('end', style({ height: '250px' })), transition('start => end', [ animate('1s 2s') ]), transition('end => start', [ animate('1s 2s') ]) ]) ] })
打开 **AppComponent** 模板 **src/app/app.component.html** 并删除示例代码。然后,包括一个带有应用标题、图像和一个用于放大/缩小图像的按钮的标题。
<h1>{{ title }}</h1> <img src="assets/puppy.jpeg" style="height: 200px" /> <br /> <button>{{ this.buttonText }}</button>
编写一个函数来更改动画表达式。
export class AppComponent { title = 'Animation Application'; isEnlarge: boolean = false; buttonText: string = "Enlarge"; triggerAnimation() { this.isEnlarge = !this.isEnlarge; if(this.isEnlarge) this.buttonText = "Shrink"; else this.buttonText = "Enlarge"; } }
在 image 标签中附加动画。此外,为按钮附加点击事件。
<h1>{{ title }}</h1> <img [@enlarge]="isEnlarge ? 'end' : 'start'" src="assets/puppy.jpeg" style="height: 200px" /> <br /> <button (click)='triggerAnimation()'>{{ this.buttonText }}</button>
完整的 **AppComponent** 代码如下:
import { Component } from '@angular/core'; import { state, style, transition, animate, trigger } from '@angular/animations'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], animations: [ trigger('enlarge', [ state('start', style({ height: '150px' })), state('end', style({ height: '250px' })), transition('start => end', [ animate('1s 2s') ]), transition('end => start', [ animate('1s 2s') ]) ]) ] }) export class AppComponent { title = 'Animation Application'; isEnlarge: boolean = false; buttonText: string = "Enlarge"; triggerAnimation() { this.isEnlarge = !this.isEnlarge; if(this.isEnlarge) this.buttonText = "Shrink"; else this.buttonText = "Enlarge"; } }
完整的 AppComponent 模板代码如下:
<h1>{{ title }}</h1> <img [@enlarge]="isEnlarge ? 'end' : 'start'" src="assets/puppy.jpeg" style="height: 200px" /> <br /> <button (click)='triggerAnimation()'>{{ this.buttonText }}</button>
使用以下命令运行应用:
ng serve
单击“放大”按钮,它将使用动画放大图像。结果将如下所示:
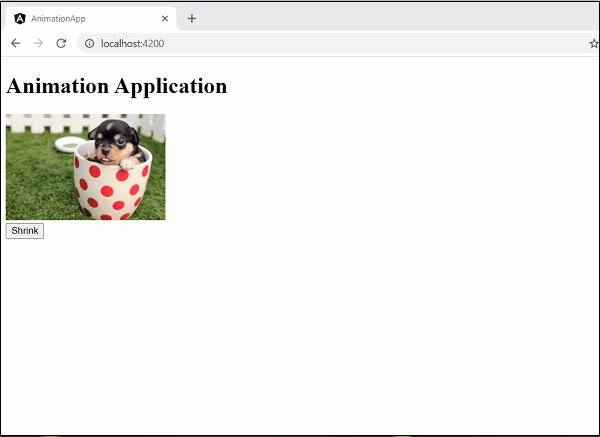
再次单击按钮以缩小它。结果将如下所示:
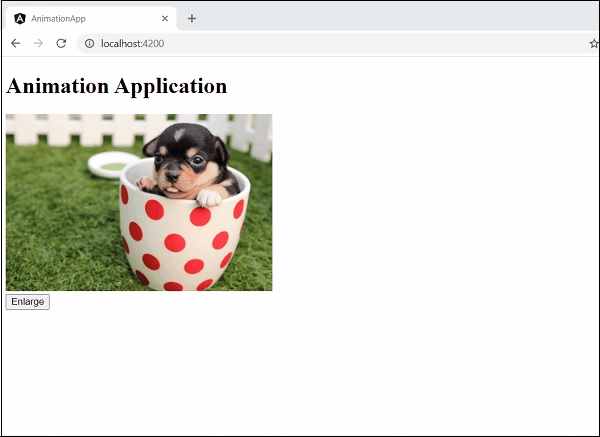