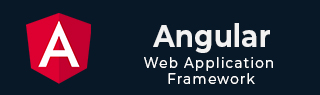
- Angular 教程
- Angular - 首页
- Angular - 概述
- Angular - 特性
- Angular - 优点与缺点
- Angular 基础
- Angular - 环境搭建
- Angular - 第一个应用
- Angular - MVC 架构
- Angular 组件
- Angular - 组件
- Angular - 组件生命周期
- Angular - 视图封装
- Angular - 组件交互
- Angular - 组件样式
- Angular - 嵌套组件
- Angular - 内容投影
- Angular - 动态组件
- Angular - 元素
- Angular 模板
- Angular - 模板
- Angular - 文本插值
- Angular - 模板语句
- Angular - 模板中的变量
- Angular - SVG 作为模板
- Angular 绑定
- Angular - 绑定及其类型
- Angular - 数据绑定
- Angular - 事件绑定
- Angular - 属性绑定
- Angular - 属性绑定
- Angular - 类和样式绑定
- Angular 指令
- Angular - 指令
- Angular - 内置指令
- Angular 管道
- Angular - 管道
- Angular - 使用管道转换数据
- Angular 依赖注入
- Angular - 依赖注入
- Angular HTTP 客户端编程
- Angular - 服务
- Angular - HTTP 客户端
- Angular - 请求
- Angular - 响应
- Angular - 获取
- Angular - PUT
- Angular - DELETE
- Angular - JSON-P
- Angular - 使用 HTTP 进行 CRUD 操作
- Angular 路由
- Angular - 路由
- Angular - 导航
- Angular - Angular Material
- Angular 动画
- Angular - 动画
- Angular 表单
- Angular - 表单
- Angular - 表单验证
- Angular Service Workers & PWA
- Angular - Service Workers & PWA
- Angular 测试
- Angular - 测试概述
- Angular NgModules
- Angular - 模块简介
- Angular 高级
- Angular - 身份验证和授权
- Angular - 国际化
- Angular - 可访问性
- Angular - Web Workers
- Angular - 服务器端渲染
- Angular - Ivy 编译器
- Angular - 使用 Bazel 构建
- Angular - 向后兼容性
- Angular - 响应式编程
- Angular - 指令和组件之间共享数据
- Angular 工具
- Angular - CLI
- Angular 其他
- Angular - 第三方控件
- Angular - 配置
- Angular - 显示数据
- Angular - 装饰器和元数据
- Angular - 基本示例
- Angular - 错误处理
- Angular - 测试和构建项目
- Angular - 生命周期钩子
- Angular - 用户输入
- Angular - 有什么新变化?
- Angular 有用资源
- Angular - 快速指南
- Angular - 有用资源
- Angular - 讨论
Angular - 内置指令
Angular 提供了许多内置指令。内置指令可以分为两种类型:
- 属性指令
- 结构指令
一些重要的指令如下:
- NgModel
- NgClass
- NgStyle
- NgNonBindable
- NgIf
- NgFor
- NgSwitch、NgSwitchCase 和 NgSwitchDefault
让我们在本节学习如何使用内置指令。
NgModel
NgModel 用于为 HTML 表单元素启用双向数据绑定。它基于属性和事件绑定。它由一个单独的模块 FormsModule 提供。一个简单的示例,用于对具有 name 属性的用户对象进行双向绑定,如下所示:
<input type="text" [(ngModel)]="user.name" />
每当用户更改输入字段时,更新的用户姓名将反映到组件中的 user.name 变量。类似地,如果组件的变量 user.name 发生更改,输入字段的值也将更新。
让我们创建一个简单的注册表单来理解 NgModel 指令。我们的注册表单将有三个输入字段,如下所示,以及一个提交注册表单的按钮。
- 用户名
- 密码
- 确认密码
步骤 1:使用 angular CLI 创建一个新的应用程序 my-app,如下所示:
ng new my-app
步骤 2:使用 angular CLI 创建一个新的注册表单组件 RegisterForm,如下所示:
ng generate component RegisterForm
步骤 3:打开注册表单组件的模板,并创建一个包含用户名、密码和确认密码的用户。
<div> <form method="post"> <div class="container"> <label for="username"><b>Username</b></label> <input type="text" name="username" required> <label for="password"><b>Password</b></label> <input type="password" name="password" required> <label for="confirm_password"><b>Confirm Password</b></label> <input type="password" name="confirm_password" required> <button type="submit">Register</button> </div> </form> </div>
步骤 4:打开注册表单组件的样式,并使用 CSS 样式化表单,如下所示:
.container { padding: 15px; } input[type=text], input[type=password] { width: 100%; padding: 10px 20px; margin: 10px 0; display: inline-block; border: 1px solid #ccc; box-sizing: border-box; } button { background-color: blue; color: white; padding: 15px 20px; margin: 10px 0; border: none; cursor: pointer; width: 100%; }
步骤 5:将我们的注册表单组件包含在应用程序模板文件 app.component.html 中。
<app-register-form />
步骤 6:运行应用程序并测试注册表单。
步骤 7:接下来,在应用程序模块文件 app.module.ts 中导入 FormsModule。
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { FormsModule } from '@angular/forms' import { AppComponent } from './app.component'; import { RegisterFormComponent } from './register-form/register-form.component'; @NgModule({ declarations: [ AppComponent, RegisterFormComponent ], imports: [ BrowserModule, FormsModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
步骤 8:接下来,让我们添加一个对象 user,它具有 Username、password 和 confirmPassword 属性。user 对象最初将为空字符串。一旦用户输入数据,它将通过 NgModel 指令填充。
user: any = { username: '', password: '', confirmPassword: '' }
步骤 9:将上述声明的组件成员变量分配给用户名、密码和确认密码输入的 NgModel 指令,如下所示:
<input type="text" name="username" [(ngModel)]="user.username" required> <input type="password" name="password" [(ngModel)]="user.password" required> <input type="password" name="confirm_password" [(ngModel)]="user.confirmPassword" required>
这里,
ngModel 将值从 user 对象设置为 HTML 输入元素。它也反向将用户输入的值设置到 user 对象。
步骤 10:在组件类中创建一个新的成员方法 showInfo。该方法的目的是收集当前用户信息并通过 alert() 方法显示它。
showInfo(e: Event) { e.preventDefault(); let info: string = ''; info += 'Username = ' + this.user.username; info += '\nPassword = ' + this.user.password; info += '\nConfirm password = ' + this.user.confirmPassword; alert(info) }
这里,
事件对象的 preventDefault() 方法将阻止提交按钮的默认操作。
alert() 方法将向用户显示消息。
步骤 11:使用事件绑定将 showInfo 方法分配给按钮的点击事件。
<button type="submit" (click)="showInfo($event)">Register</button>
步骤 12:组件的完整列表如下:
import { Component } from '@angular/core'; @Component({ selector: 'app-register-form', templateUrl: './register-form.component.html', styleUrls: ['./register-form.component.css'] }) export class RegisterFormComponent { user: any = { username: '', password: '', confirmPassword: '' } showInfo(e: Event) { e.preventDefault(); let info: string = ''; info += 'Username = ' + this.user.username; info += '\nPassword = ' + this.user.password; info += '\nConfirm password = ' + this.user.confirmPassword; alert(info) } }
步骤 13:组件模板的完整列表如下:
<div> <form method="post"> <div class="container"> <label for="username"><b>Username</b></label> <input type="text" name="username" [(ngModel)]="user.username" required> <label for="password"><b>Password</b></label> <input type="password" name="password" [(ngModel)]="user.password" required> <label for="confirm_password"><b>Confirm Password</b></label> <input type="password" name="confirm_password" [(ngModel)]="user.confirmPassword" required> <button type="submit" (click)="showInfo($event)">Register</button> </div> </form> </div>
步骤 14:接下来,运行应用程序,填写表单并单击注册按钮。它将通过 ngModel 绑定收集信息,并通过 alert 函数显示它。
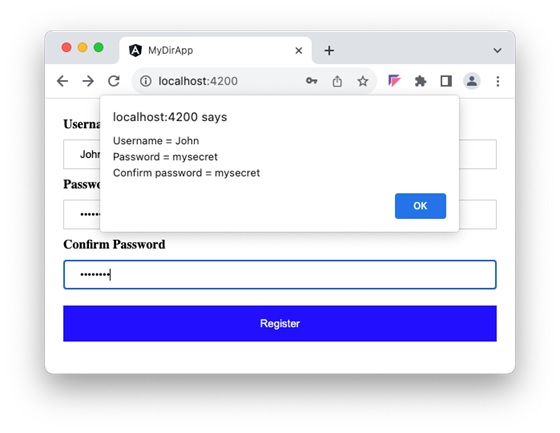
NgStyle
NgStyle 用于向宿主 HTML 元素或组件添加或删除一组 HTML 样式。
'''html <div NgStyle="stylelist">
这里,style-list 是 Record<string, string> 类型的对象。所有键都表示 CSS 属性,其对应的值表示其 CSS 值。一个示例对象如下所示:
classlist: Record<string, boolean> = { 'color': 'red', 'text-decoration': 'underline', 'font-size': '12px' }
让我们扩展我们的注册表单组件以支持验证,并使用 ngStyle 高亮显示必填字段。
步骤 1:在组件中添加新的成员变量 inputStyle。
inputStyle: Record<string, string> = { 'border-color': '#ccc' }
步骤 2:更新 showInfo() 方法以包含验证逻辑并更新 inputStyle 对象。
NgClass
NgClass 用于向宿主 HTML 元素或组件添加或删除一组类。
```html <div NgClass=“classlist”>
这里,classlist 是 Record<string, boolean> 类型的对象。所有具有真值的键都将设置为宿主元素的类。一个示例对象如下所示:
classlist: Record<string, boolean> = { 'c1': true, 'c2': false, 'c3': true }
NgNonBindable
NgNonBindable 用于阻止对一段文本的 Angular 模板进行处理。只需将文本用具有 ngNonBindable 属性的 HTML 元素括起来即可。它将按原样发出接收到的模板,而不会处理模板表达式、模板语句、指令和组件。
```html
<!-- any angular expression / directive / component --> Hi, angular don't evaluate me and it can have any angular expression such as {{ 1 + 1 }}, {{ name | uppercase }}. I can have any component as well. <mycomp input="Hello" />
它用于在 Angular 应用程序本身中展示 Angular 代码,而不会受到模板处理的影响。
NgIf
Angular 模板没有条件逻辑。条件逻辑是使用 NgIf 指令完成的。它根据作为值接受的条件(模板表达式)将宿主 HTML 元素/组件添加到 DOM 布局或从 DOM 布局中删除。
<div *ngIf="canShow"> <!-- content --> </div>
如果 canShow 为真,则显示内部内容。否则,内容不会添加到 DOM 布局中。
NgFor
Angular 模板也没有循环逻辑。为了弥补循环逻辑的缺失,Angular 提供了 NgFor 指令来循环遍历项目数组并将其显示为列表、画廊、行等。
<ul *ngFor="let item of items"> <li>{{ item }}</li> </ul>
它将在无序列表中生成 n 个项目,其中 n 表示 items 数组中可用的项目数。
NgSwitch、NgSwitchCase 和 NgSwitchDefault
Angular 模板也没有 switch 语句。为了弥补切换逻辑的缺失,Angular 提供了 NgSwitch 及其相关指令来选择集合(项目)中的任何一个项目。
<div [ngSwitch]="role"> <div *ngSwitchCase="'admin'"> Hi, I have access to admin items </div> <div *ngSwitchCase="'user'"> Hi, I have access to user items only </div> <div *ngSwitchDefault> Hi, I have access to guest items only. Please login to access more items </div> </div>
这里,将根据用户的类型显示任何一个部分。