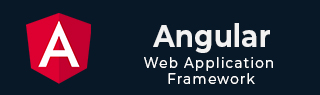
- Angular 教程
- Angular - 首页
- Angular - 概述
- Angular - 特性
- Angular - 优点与缺点
- Angular 基础
- Angular - 环境设置
- Angular - 第一个应用
- Angular - MVC 架构
- Angular 组件
- Angular - 组件
- Angular - 组件生命周期
- Angular - 视图封装
- Angular - 组件交互
- Angular - 组件样式
- Angular - 嵌套组件
- Angular - 内容投影
- Angular - 动态组件
- Angular - 元素
- Angular 模板
- Angular - 模板
- Angular - 文本插值
- Angular - 模板语句
- Angular - 模板中的变量
- Angular - SVG 作为模板
- Angular 绑定
- Angular - 绑定及其类型
- Angular - 数据绑定
- Angular - 事件绑定
- Angular - 属性绑定
- Angular - 属性绑定
- Angular - 类和样式绑定
- Angular 指令
- Angular - 指令
- Angular - 内置指令
- Angular 管道
- Angular - 管道
- Angular - 使用管道转换数据
- Angular 依赖注入
- Angular - 依赖注入
- Angular HTTP 客户端编程
- Angular - 服务
- Angular - HTTP 客户端
- Angular - 请求
- Angular - 响应
- Angular - GET
- Angular - PUT
- Angular - DELETE
- Angular - JSON-P
- Angular - 使用 HTTP 进行 CRUD 操作
- Angular 路由
- Angular - 路由
- Angular - 导航
- Angular - Angular Material
- Angular 动画
- Angular - 动画
- Angular 表单
- Angular - 表单
- Angular - 表单验证
- Angular Service Workers 和 PWA
- Angular - Service Workers 和 PWA
- Angular 测试
- Angular - 测试概述
- Angular NgModule
- Angular - 模块介绍
- Angular 高级
- Angular - 身份验证和授权
- Angular - 国际化
- Angular - 可访问性
- Angular - Web Workers
- Angular - 服务器端渲染
- Angular - Ivy 编译器
- Angular - 使用 Bazel 构建
- Angular - 向后兼容性
- Angular - 响应式编程
- Angular - 指令和组件之间的数据共享
- Angular 工具
- Angular - CLI
- Angular 其他
- Angular - 第三方控件
- Angular - 配置
- Angular - 显示数据
- Angular - 装饰器和元数据
- Angular - 基本示例
- Angular - 错误处理
- Angular - 测试和构建项目
- Angular - 生命周期钩子
- Angular - 用户输入
- Angular - 最新动态?
- Angular 有用资源
- Angular - 快速指南
- Angular - 有用资源
- Angular - 讨论
Angular - 属性绑定
属性绑定是 Angular 提供的最简单的绑定方式。它用于设置 HTML 元素属性的值。Angular 通过与属性名称匹配的属性公开 HTML 元素的属性,并将属性名称转换为 camelCase。例如,colspan 属性对应的 Angular 属性是 colSpan。
尽管 Angular 尝试将所有 HTML 元素属性作为属性提供,但它仍然缺少一些 SVG 元素、aria(Web 可访问性)元素等的属性。当 HTML 元素的属性不可作为属性使用时,可以使用属性绑定。此外,可以对所有 HTML 元素的属性使用属性绑定。
语法
在模板中表示 HTML 元素属性的语法如下:
<HTMLTag [attr.<attribute_name>]="<template variable>" />
可以将 attr. 字符串组合到 HTML 元素的实际属性名称中,以创建 Angular 属性表示。属性的值基本上是一个模板变量。在从模板生成视图时,Angular 将通过处理模板变量来设置属性的值。
应用属性绑定
让我们创建一个简单的注册表单来了解属性绑定。我们的注册表单将有三个输入字段,如下所示,以及一个提交注册表单的按钮。
- 用户名
- 密码
- 确认密码
步骤 1:使用 Angular CLI 创建一个新应用程序 my-app,如下所示:
ng new my-app
步骤 2:使用 Angular CLI 创建一个新的注册表单组件 RegisterForm,如下所示:
ng generate component RegisterForm
步骤 3:打开注册表单组件的模板,并添加带有用户名、密码和确认密码的用户注册表单。
<div> <form method="post"> <div class="container"> <label for="username"><b>Username</b></label> <input type="text" name="username" required> <label for="password"><b>Password</b></label> <input type="password" name="password" required> <label for="confirm_password"><b>Confirm Password</b></label> <input type="password" name="confirm_password" required> <button type="submit">Register</button> </div> </form> </div>
步骤 4:打开注册表单组件的样式,并使用 CSS 样式化注册表单,如下所示:
.container { padding: 15px; } input[type=text], input[type=password] { width: 100%; padding: 10px 20px; margin: 10px 0; display: inline-block; border: 1px solid #ccc; box-sizing: border-box; } button { background-color: blue; color: white; padding: 15px 20px; margin: 10px 0; border: none; cursor: pointer; width: 100%; }
步骤 5:将我们的注册表单组件包含在应用程序模板文件 app.component.html 中。
<app-register-form />
步骤 6:运行应用程序并测试注册表单。
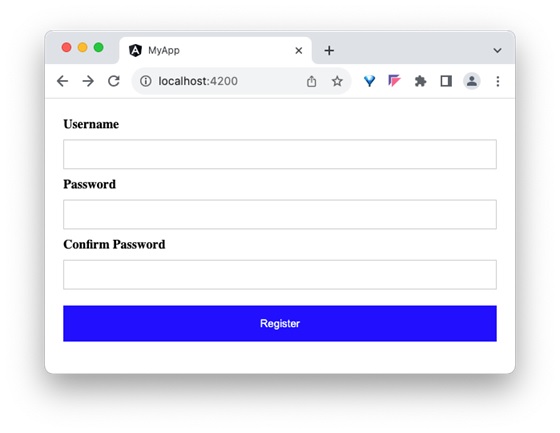
步骤 7:接下来,我们将尝试使用属性绑定为所有输入字段设置占位符文本。在组件中添加三个成员变量来表示用户名、密码和确认密码输入字段的占位符文本。
placeholder1: string = "Enter username" placeholder2: string = "Enter password" placeholder3: string = "Repeat password"
步骤 8:使用 [attr.placeholder] 属性,将上面声明的组件成员变量分别分配给用户名、密码和确认密码输入的占位符属性,如下所示:
<div> <form method="post"> <div class="container"> <label for="username"><b>Username</b></label> <input type="text" [attr.placeholder]="placeholder1" name="username" required> <label for="password"><b>Password</b></label> <input type="password" [attr.placeholder]="placeholder2" name="password" required> <label for="confirm_password"><b>Confirm Password</b></label> <input type="password" [attr.placeholder]="placeholder3" name="confirm_password" required> <button type="submit">Register</button> </div> </form> </div>
这里:
attr.placeholder 表示输入元素的占位符属性。
步骤 9:让我们向输入字段添加 ARIA 属性 aria-label。aria-label 用于辅助功能。
<div> <form method="post"> <div class="container"> <label for="username"><b>Username</b></label> <input type="text" [attr.aria-label]="placeholder1" [attr.placeholder]="placeholder1" name="username" required> <label for="password"><b>Password</b></label> <input type="password" [attr.aria-label]="placeholder2" [attr.placeholder]="placeholder2" name="password" required> <label for="confirm_password"><b>Confirm Password</b></label> <input type="password" [attr.aria-label]="placeholder3" [attr.placeholder]="placeholder3" name="confirm_password" required> <button type="submit">Register</button> </div> </form> </div>
步骤 10:接下来,运行应用程序并检查输出。
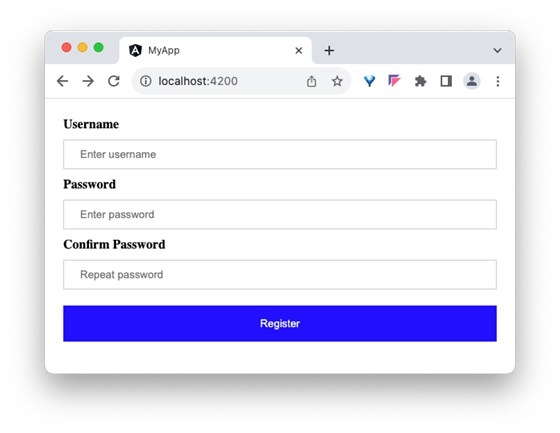
步骤 11:由于可以使用属性绑定设置 HTML 元素的任何属性,让我们使用属性绑定应用类 container。
步骤 12:在组件中创建一个新的成员变量 myContainerClass,如下所示:
myContainerClass: string = "container"
步骤 13:在模板中应用成员变量,如下所示:
<div [attr.class]="myContainerClass"> <!-- form --> </div>
步骤 14:组件的完整列表如下:
import { Component } from '@angular/core'; @Component({ selector: 'app-login-form', templateUrl: './register-form.component.html', styleUrls: ['./register-form.component.css'] }) export class RegisterFormComponent { myContainerClass: string = "container" placeholder1: string = "Enter username" placeholder2: string = "Enter password" placeholder3: string = "Repeat password" }
步骤 15:组件模板的完整列表如下:
<div> <form method="post"> <div class="container"> <label for="username"><b>Username</b></label> <input type="text" [attr.aria-label]="placeholder1" [attr.placeholder]="placeholder1" name="username" required> <label for="password"><b>Password</b></label> <input type="password" [attr.aria-label]="placeholder2" [attr.placeholder]="placeholder2" name="password" required> <label for="confirm_password"><b>Confirm Password</b></label> <input type="password" [attr.aria-label]="placeholder3" [attr.placeholder]="placeholder3" name="confirm_password" required> <button type="submit">Register</button> </div> </form> </div>
步骤 16:接下来,运行应用程序并检查输出。
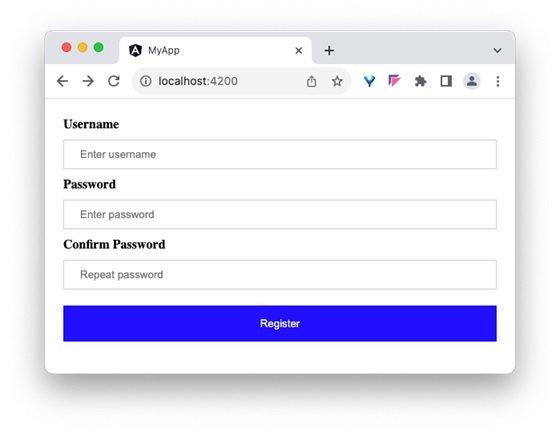
结论
属性绑定使开发人员能够为任何 HTML 元素的属性设置动态值,即使是将来可能定义的元素。