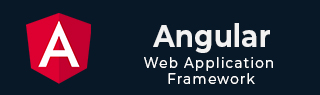
- Angular 教程
- Angular - 首页
- Angular - 概述
- Angular - 特性
- Angular - 优点与缺点
- Angular 基础
- Angular - 环境设置
- Angular - 第一个应用
- Angular - MVC 架构
- Angular 组件
- Angular - 组件
- Angular - 组件生命周期
- Angular - 视图封装
- Angular - 组件交互
- Angular - 组件样式
- Angular - 嵌套组件
- Angular - 内容投影
- Angular - 动态组件
- Angular - 元素
- Angular 模板
- Angular - 模板
- Angular - 文本插值
- Angular - 模板语句
- Angular - 模板中的变量
- Angular - SVG 作为模板
- Angular 绑定
- Angular - 绑定及其类型
- Angular - 数据绑定
- Angular - 事件绑定
- Angular - 属性绑定
- Angular - 属性绑定
- Angular - 类和样式绑定
- Angular 指令
- Angular - 指令
- Angular - 内置指令
- Angular 管道
- Angular - 管道
- Angular - 使用管道转换数据
- Angular 依赖注入
- Angular - 依赖注入
- Angular HTTP 客户端编程
- Angular - 服务
- Angular - HTTP 客户端
- Angular - 请求
- Angular - 响应
- Angular - 获取
- Angular - PUT
- Angular - DELETE
- Angular - JSON-P
- Angular - 使用 HTTP 进行 CRUD 操作
- Angular 路由
- Angular - 路由
- Angular - 导航
- Angular - Angular Material
- Angular 动画
- Angular - 动画
- Angular 表单
- Angular - 表单
- Angular - 表单验证
- Angular Service Workers 和 PWA
- Angular - Service Workers 和 PWA
- Angular 测试
- Angular - 测试概述
- Angular NgModules
- Angular - 模块简介
- Angular 高级
- Angular - 身份验证和授权
- Angular - 国际化
- Angular - 可访问性
- Angular - Web Workers
- Angular - 服务器端渲染
- Angular - Ivy 编译器
- Angular - 使用 Bazel 构建
- Angular - 向后兼容性
- Angular - 响应式编程
- Angular - 在指令和组件之间共享数据
- Angular 工具
- Angular - CLI
- Angular 其他
- Angular - 第三方控件
- Angular - 配置
- Angular - 显示数据
- Angular - 装饰器和元数据
- Angular - 基本示例
- Angular - 错误处理
- Angular - 测试和构建项目
- Angular - 生命周期钩子
- Angular - 用户输入
- Angular - 有什么新功能?
- Angular 有用资源
- Angular - 快速指南
- Angular - 有用资源
- Angular - 讨论
Angular - 获取
HttpClient get() 方法
要从服务器获取资源,可以使用 HTTP 动词 get。get 的目的是简单地请求一个资源。服务器将检查服务器中的资源并将其发送回,如果服务器中存在特定的资源。
Angular HttpClient 提供了一个方法 get(),用于使用 get HTTP 动词请求服务器。让我们在本节中学习 HttpClient get() 方法。
get() 方法的签名
get() 方法的签名如下:
get(<url as string>, <options as object>)
url 表示要请求的资源的 uri。
options 表示要与资源 url 一起发送的选项。
工作示例
为了实现 HTTP 客户端-服务器通信,我们需要设置一个 Web 应用程序,并需要公开一组 Web API。可以从客户端请求 Web API。让我们创建一个示例服务器应用程序,Expense API App,为费用提供 CRUD REST API(主要是 get 请求)。
步骤 1:转到您喜欢的 workspace,如下所示:
cd /go/to/your/favorite/workspace
步骤 2:创建一个新文件夹 expense-rest-api 并移动到该文件夹中
mkdir expense-rest-api && cd expense-rest-api
步骤 3:使用 npm 命令提供的 init 子命令创建一个新应用程序,如下所示:
npm init
以上命令会询问一些问题,并使用默认答案回答所有问题。
步骤 4:安装 express 和 cors 包以创建基于节点的 Web 应用程序。
npm install express cors --save
步骤 5:安装 sqlite 包以将费用存储在基于 sqlite 的数据库中
npm install sqlite3 --save
步骤 6:创建一个新文件 sqlitedb.js,并在其中添加以下代码以使用费用表和示例费用条目初始化数据库。费用表将用于存储费用项目
var sqlite3 = require('sqlite3').verbose() const DBSOURCE = "expensedb.sqlite" let db = new sqlite3.Database(DBSOURCE, (err) => { if (err) { console.error(err.message) throw err }else{ console.log('Connected to the SQLite database.') db.run(`CREATE TABLE IF NOT EXISTS expense ( id INTEGER PRIMARY KEY AUTOINCREMENT, item text, amount real, category text, location text, spendOn text, createdOn text )`, (err) => { if (err) { console.log(err); }else{ var insert = 'INSERT INTO expense (item, amount, category, location, spendOn, createdOn) VALUES (?,?,?,?,?,?)' db.run(insert, ['Pizza', 10, 'Food', 'KFC', '2020-05-26 10:10', '2020-05-26 10:10']) db.run(insert, ['Pizza', 9, 'Food', 'Mcdonald', '2020-05-28 11:10', '2020-05-28 11:10']) db.run(insert, ['Pizza', 12, 'Food', 'Mcdonald', '2020-05-29 09:22', '2020-05-29 09:22']) db.run(insert, ['Pizza', 15, 'Food', 'KFC', '2020-06-06 16:18', '2020-06-06 16:18']) db.run(insert, ['Pizza', 14, 'Food', 'Mcdonald', '2020-06-01 18:14', '2020-05-01 18:14']) } } ); } }); module.exports = db
步骤 7:打开 index.js 并更新以下代码:
var express = require("express") var cors = require('cors') var db = require("./sqlitedb.js") var app = express() app.use(cors()); var bodyParser = require("body-parser"); app.use(express.urlencoded({ extended: true })); app.use(express.json()); var HTTP_PORT = 8000 app.listen(HTTP_PORT, () => { console.log("Server running on port %PORT%".replace("%PORT%", HTTP_PORT)) }); app.get("/", (req, res, next) => { res.json({ "message": "Ok" }) }); app.get("/api/expense", (req, res, next) => { var sql = "select * from expense" var params = [] db.all(sql, params, (err, rows) => { if (err) { res.status(400).json({ "error": err.message }); return; } res.json(rows) }); }); app.get("/api/expense/:id", (req, res, next) => { var sql = "select * from expense where id = ?" var params = [req.params.id] db.get(sql, params, (err, row) => { if (err) { res.status(400).json({ "error": err.message }); return; } res.json(row) }); }); app.use(function (req, res) { res.status(404); });
这里,代码将创建以下六个提到的 REST API 端点。
/ 端点返回 OK 消息以确保应用程序正常工作。
/api/expense 端点返回数据库中所有可用的费用项目。
/api/expense/:id 端点根据费用条目 ID 返回费用条目。
步骤 8:运行应用程序,如下所示:
node index.js
步骤 9:要测试应用程序并确保其正常工作,请打开浏览器并转到 https://127.0.0.1:8000/。如果应用程序正常工作,它应该返回以下消息。
{ "message": "Ok" }
让我们创建一个可行的 Angular 示例,通过使用 HttpClient 服务类和 get() 方法从上述服务器应用程序获取所有费用项目。
步骤 1:通过运行 ng new 命令创建一个新的 Angular 应用程序,如下所示:
ng new my-http-app
启用 Angular 路由和 CSS,如下所示:
? Would you like to add Angular routing? Yes ? Which stylesheet format would you like to use? CSS
步骤 2:通过在模块配置文件 (app.module.ts) 中导入 HttpClientModule 来启用应用程序中的 http 通信。
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { HttpClientModule } from '@angular/common/http'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, AppRoutingModule, HttpClientModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
这里,
从 @angular/common/http 模块导入 HttpClientModule。
将 HttpClientModule 添加到 @NgModule 配置的 imports 部分。
步骤 3:创建一个新的接口 Expense 来表示我们的费用项目。
interface Expense { id: Number, item: String, amount: Number, category: String, location: String, spendOn: Date } export default Expense;
步骤 4:创建新的组件 ListExpenses 以显示来自服务器的费用项目。
ng generate component ListExpenses
它将创建如下所示的组件:
CREATE src/app/list-expenses/list-expenses.component.css (0 bytes) CREATE src/app/list-expenses/list-expenses.component.html (28 bytes) CREATE src/app/list-expenses/list-expenses.component.spec.ts (602 bytes) CREATE src/app/list-expenses/list-expenses.component.ts (229 bytes) UPDATE src/app/app.module.ts (581 bytes)
步骤 5:将我们的新组件包含到 App 根组件的视图 app.component.html 中,如下所示:
<app-list-expenses></app-list-expenses> <router-outlet></router-outlet>
步骤 6:通过构造函数将 HttpClient 注入到 ListExpenses 组件中,如下所示:
import { Component } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Component({ selector: 'app-list-expenses', templateUrl: './list-expenses.component.html', styleUrls: ['./list-expenses.component.css'] }) export class ListExpensesComponent { constructor(private http: HttpClient) { } }
步骤 7:实现 OnInit 生命周期钩子,以便在 ListExpenses 组件初始化后请求服务器的费用。
export class ListExpensesComponent implements OnInit{ constructor(private http: HttpClient) { } ngOnInit(): void { } }
步骤 8:创建一个局部变量 expenses 来保存来自服务器的费用。
export class ListExpensesComponent implements OnInit{ expenses: Expense[] = []; constructor(private http: HttpClient) { } ngOnInit(): void { } }
步骤 9:通过传递 url 和 options 调用 this.http(HttpClient 实例)对象的 get 方法,并从服务器获取费用对象。然后,将费用设置为我们的局部变量 expenses。
export class ListExpensesComponent implements OnInit{ expenses: Expense[] = []; constructor(private http: HttpClient) { } ngOnInit(): void { this.http.get<Expense[]>('https://127.0.0.1:8000/api/expense', { 'observe': 'body', 'responseType': 'json' }) .subscribe( data => { this.expenses = data as Expense[] console.log(this.expenses) }) } }
这里,
将 Expense[] 设置为服务器返回的对象的类型。服务器将在其主体中以 json 格式发送费用对象的数组。
订阅请求 (this.http.get) 对象。然后将订阅的数据解析为费用对象的数组,并将其设置为局部费用变量 (this.expenses)
步骤 10:ListExpensesComponent 的完整代码如下:
import { Component, OnInit } from '@angular/core'; import { HttpClient, HttpRequest, HttpResponse, HttpEvent } from '@angular/common/http'; import Expense from '../Expense'; @Component({ selector: 'app-list-expenses', templateUrl: './list-expenses.component.html', styleUrls: ['./list-expenses.component.css'] }) export class ListExpensesComponent implements OnInit { expenses: Expense[] = []; constructor(private http: HttpClient) { } ngOnInit(): void { this.http.get<Expense[]>('https://127.0.0.1:8000/api/expense', { 'observe': 'body', 'responseType': 'json' }) .subscribe( data => { this.expenses = data as Expense[] console.log(this.expenses) }) } }
步骤 11:接下来,从组件获取 expenses 对象并在我们的组件模板页面 (list-expenses.component.html) 中呈现它
<div><h3>Expenses</h3></div> <ul> <li *ngFor="let expense of expenses"> {{expense.item}} @ {{expense.location}} for {{expense.amount}} USD on {{expense.spendOn | date:'shortDate' }} </li> </ul>
步骤 12:最后,使用以下命令运行应用程序,
ng serve
步骤 13:打开浏览器并导航到 https://127.0.0.1:4200/ url 并检查输出
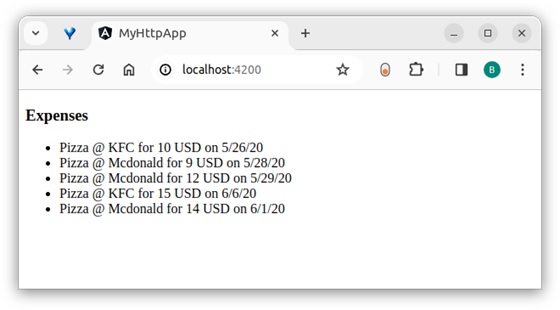
这里,输出显示我们的费用作为项目列表。
结论
Angular 提供了一种简单的方法通过 HttpClient 对象请求服务器。get() 是一个用于从服务器获取资源的特定方法。我们将在接下来的章节中学习更多 HTTP 方法来定位其他 HTTP 动词。