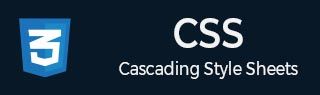
- CSS 教程
- CSS - 首页
- CSS - 路线图
- CSS - 简介
- CSS - 语法
- CSS - 选择器
- CSS - 包含
- CSS - 测量单位
- CSS - 颜色
- CSS - 背景
- CSS - 字体
- CSS - 文本
- CSS - 图片
- CSS - 链接
- CSS - 表格
- CSS - 边框
- CSS - 块级边框
- CSS - 内联边框
- CSS - 外边距
- CSS - 列表
- CSS - 内边距
- CSS - 光标
- CSS - 轮廓
- CSS - 尺寸
- CSS - 滚动条
- CSS - 块级内联
- CSS - 下拉菜单
- CSS - 可见性
- CSS - 溢出
- CSS - 清除浮动
- CSS - 浮动
- CSS - 箭头
- CSS - 调整大小
- CSS - 引号
- CSS - 顺序
- CSS - 定位
- CSS - 连字符
- CSS - 悬停
- CSS - 显示
- CSS - 聚焦
- CSS - 缩放
- CSS - 位移
- CSS - 高度
- CSS - 连字符字符
- CSS - 宽度
- CSS - 不透明度
- CSS - Z-Index
- CSS - 底部
- CSS - 导航栏
- CSS - 叠加层
- CSS - 表单
- CSS - 对齐
- CSS - 图标
- CSS - 图片库
- CSS - 注释
- CSS - 加载器
- CSS - 属性选择器
- CSS - 组合器
- CSS - 根元素
- CSS - 盒模型
- CSS - 计数器
- CSS - 剪裁
- CSS - 书写模式
- CSS - Unicode-bidi
- CSS - min-content
- CSS - 全部
- CSS - 内嵌
- CSS - 隔离
- CSS - 溢出滚动
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - 指针事件
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - 最大块级尺寸
- CSS - 最小块级尺寸
- CSS - 混合模式
- CSS - 最大内联尺寸
- CSS - 最小内联尺寸
- CSS - 偏移
- CSS - 强调色
- CSS - 用户选择
- CSS 高级
- CSS - 网格
- CSS - 网格布局
- CSS - Flexbox
- CSS - 可见性
- CSS - 定位
- CSS - 层
- CSS - 伪类
- CSS - 伪元素
- CSS - @规则
- CSS - 文字效果
- CSS - 分页媒体
- CSS - 打印
- CSS - 布局
- CSS - 验证
- CSS - 图片精灵
- CSS - !important
- CSS - 数据类型
- CSS3 教程
- CSS3 - 教程
- CSS - 圆角
- CSS - 边框图片
- CSS - 多重背景
- CSS - 颜色
- CSS - 渐变
- CSS - 盒阴影
- CSS - 盒装饰中断
- CSS - 光标颜色
- CSS - 文本阴影
- CSS - 文本
- CSS - 二维变换
- CSS - 三维变换
- CSS - 过渡
- CSS - 动画
- CSS - 多列
- CSS - 盒尺寸
- CSS - 工具提示
- CSS - 按钮
- CSS - 分页
- CSS - 变量
- CSS - 媒体查询
- CSS - 函数
- CSS - 数学函数
- CSS - 遮罩
- CSS - 形状
- CSS - 样式图片
- CSS - 特效性
- CSS - 自定义属性
- CSS 响应式
- CSS RWD - 简介
- CSS RWD - 视口
- CSS RWD - 网格视图
- CSS RWD - 媒体查询
- CSS RWD - 图片
- CSS RWD - 视频
- CSS RWD - 框架
- CSS 工具
- CSS - PX 到 EM 转换器
- CSS - 颜色选择器和动画
- CSS 资源
- CSS - 有用资源
- CSS - 讨论
CSS - 加载器
**CSS 加载器**是用于指示网页加载过程的动画效果。它们使用 CSS 实现,可以应用于网页上的各种元素,例如旋转器或加载进度条。CSS 加载器通常用于通过视觉方式指示内容正在加载或处理来改善用户体验。
CSS 加载器示例
在这里你可以看到什么是 CSS 加载器,你可能在不同的网站上见过这种加载动画。
目录
如何创建一个 CSS 加载器?
要创建 CSS 加载器,请按照以下步骤操作。
- **创建 HTML 结构:** 定义一个外部 div 容器元素来容纳加载器内容,以及一个内部 div 容器用于加载器。
- **设置加载器容器样式:** 设置加载器容器的**宽度**和**高度**。使用 flex 布局将加载器元素正确地居中在容器内。
- **设置加载器元素样式:** 也要设置加载器元素的高度和宽度。然后根据所需的加载器宽度和颜色设置**border-top**属性。还可以使用border-radius值为 50% 以确保加载器为圆形。
- **向加载器添加动画:** 使用**CSS 动画**来创建加载效果。通过这种方式,你可以添加旋转效果、缩放或任何其他变换。
- **定义动画的关键帧:** 为你的动画指定**@keyframes 规则**,详细说明加载器如何随时间推移移动或变化。
你可以为加载器使用各种颜色组合、形状、图案和动画技巧。尝试使用 CSS 属性来创建你的加载器。
对于不支持动画和变换属性的浏览器,你需要在代码中添加**-webkit-**前缀。
基本的 CSS 加载器示例
以下示例演示了根据上一节中讨论的内容使用 CSS 创建加载器。
示例
<!DOCTYPE html> <html> <head> <style> .container { display: flex; justify-content: space-around; align-items: center; height: 150px; background-color: #e0f7e9; margin: 0; } .loader { border: 8px solid #e0f7e9; border-top: 8px solid #34a853; border-radius: 50%; width: 60px; height: 60px; animation: innerFrame-spin 2s ease infinite; } @keyframes innerFrame-spin { 0% { transform: rotate(0deg); } 100% { transform: rotate(360deg); } } </style> </head> <body> <div class="container"> <div class="loader"> </div> </div> </body> </html>
创建脉冲点加载器
脉冲点加载器通常在启动系统时用于 Windows 操作系统。我们使用加载器内的六个 div 元素创建了这个加载器,每个元素都使用伪类**:nth-child**设置了不同的动画延迟。
示例
<!DOCTYPE html> <html> <head> <style> .container { display: flex; justify-content: space-around; align-items: center; height: 150px; background-color: #e0f7e9; margin: 0; } .PulsingDotsLoader { display: flex; justify-content: space-around; align-items: center; width: 60px; height: 60px; } .PulsingDotsLoader div { width: 12px; height: 12px; background-color: #34a853; border-radius: 50%; animation: PulsingDotsLoader-animation 1.2s ease infinite; } .PulsingDotsLoader div:nth-child(1) { animation-delay: -0.6s; } .PulsingDotsLoader div:nth-child(2) { animation-delay: -0.5s; } .PulsingDotsLoader div:nth-child(3) { animation-delay: -0.4s; } .PulsingDotsLoader div:nth-child(4) { animation-delay: -0.3s; } .PulsingDotsLoader div:nth-child(5) { animation-delay: -0.2s; } .PulsingDotsLoader div:nth-child(6) { animation-delay: -0.1s; } @keyframes PulsingDotsLoader-animation { 0%, 100% { transform: scale(1); opacity: 1; } 50% { transform: scale(0.5); opacity: 0.3; } } </style> </head> <body> <div class="container"> <div class="PulsingDotsLoader"> <div> </div> <div> </div> <div> </div> <div> </div> <div> </div> <div> </div> </div> </div> </body> </html>
不同类型的旋转加载器
此示例显示了具有多个旋转器的加载器。
- 第一个加载器有两个旋转器,因为我们定义了属性**border-top**和**border-bottom**。
- 对于第二个加载器,我们除了之前定义的顶部和底部值之外,还定义了**border-right**,这使得它成为一个三个旋转器的加载器。
- 对于第三个加载器,我们为 border 属性定义了四个不同的值,这使得它成为一个四个旋转器的加载器。
示例
<!DOCTYPE html> <html> <head> <style> body { display: flex; justify-content: space-around; align-items: center; height: 200px; background-color: #f0f0f0; } .loader { border: 16px solid #f3f3f3; /* Light grey */ border-top: 16px solid blue; border-bottom: 16px solid blue; border-radius: 50%; width: 70px; height: 70px; animation: spin 2s linear infinite; } .second{ border-right: 16px solid blue; } .third{ border-top: 16px solid #ADD8E6; /* Light Blue */ border-right: 16px solid #87CEEB; /* Sky Blue */ border-bottom: 16px solid #1E90FF; /* Dodger Blue */ border-left: 16px solid #4169E1; /* Royal Blue */ } @keyframes spin { 0% { transform: rotate(0deg); } 100% { transform: rotate(360deg); } } </style> </head> <body> <div class="loader"> </div> <div class="loader second"> </div> <div class="loader third"> </div> </body> </html>
使用渐变的 CSS 加载器
CSS **渐变**可以通过在两种或多种颜色之间创建平滑过渡来设计加载器元素的自定义颜色。
示例
<html> <head> <style> .loader-test { width: 50px; height: 50px; padding: 10px; aspect-ratio: 1; border-radius: 50%; margin: 20px; background: linear-gradient(10deg,#ccc,red); mask: conic-gradient(#0000,#000), linear-gradient(#000 0 0) content-box; -webkit-mask-composite: source-out; mask-composite: subtract; animation: load 1s linear infinite; } @keyframes load { to{ transform: rotate(1turn) } } </style> </head> <body> <div class="loader-test"> </div> </body> </html>
广告