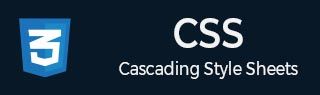
- CSS 教程
- CSS - 首页
- CSS - 路线图
- CSS - 简介
- CSS - 语法
- CSS - 选择器
- CSS - 包含
- CSS - 测量单位
- CSS - 颜色
- CSS - 背景
- CSS - 字体
- CSS - 文本
- CSS - 图片
- CSS - 链接
- CSS - 表格
- CSS - 边框
- CSS - 块级边框
- CSS - 行内边框
- CSS - 外边距
- CSS - 列表
- CSS - 内边距
- CSS - 光标
- CSS - 轮廓
- CSS - 尺寸
- CSS - 滚动条
- CSS - 行内块
- CSS - 下拉菜单
- CSS - 可见性
- CSS - 溢出
- CSS - 清除浮动
- CSS - 浮动
- CSS - 箭头
- CSS - 调整大小
- CSS - 引号
- CSS - 顺序
- CSS - 定位
- CSS - 连字符
- CSS - 悬停
- CSS - 显示
- CSS - 焦点
- CSS - 缩放
- CSS - 位移
- CSS - 高度
- CSS - 连字符字符
- CSS - 宽度
- CSS - 不透明度
- CSS - Z-index
- CSS - 底部
- CSS - 导航栏
- CSS - 叠加层
- CSS - 表单
- CSS - 对齐
- CSS - 图标
- CSS - 图片库
- CSS - 注释
- CSS - 加载器
- CSS - 属性选择器
- CSS - 组合器
- CSS - 根元素
- CSS - 盒模型
- CSS - 计数器
- CSS - 剪裁
- CSS - 书写模式
- CSS - Unicode-bidi
- CSS - min-content
- CSS - all
- CSS - inset
- CSS - isolation
- CSS - overscroll
- CSS - justify-items
- CSS - justify-self
- CSS - tab-size
- CSS - pointer-events
- CSS - place-content
- CSS - place-items
- CSS - place-self
- CSS - max-block-size
- CSS - min-block-size
- CSS - mix-blend-mode
- CSS - max-inline-size
- CSS - min-inline-size
- CSS - offset
- CSS - accent-color
- CSS - user-select
- CSS 高级
- CSS - 网格
- CSS - 网格布局
- CSS - Flexbox
- CSS - 可见性
- CSS - 定位
- CSS - 图层
- CSS - 伪类
- CSS - 伪元素
- CSS - @规则
- CSS - 文字效果
- CSS - 分页媒体
- CSS - 打印
- CSS - 布局
- CSS - 验证
- CSS - 图片精灵
- CSS - !important
- CSS - 数据类型
- CSS3 教程
- CSS3 - 教程
- CSS - 圆角
- CSS - 边框图片
- CSS - 多背景
- CSS - 颜色
- CSS - 渐变
- CSS - 盒阴影
- CSS - box-decoration-break
- CSS - caret-color
- CSS - 文本阴影
- CSS - 文本
- CSS - 2D 变换
- CSS - 3D 变换
- CSS - 过渡
- CSS - 动画
- CSS - 多列
- CSS - box-sizing
- CSS - 提示框
- CSS - 按钮
- CSS - 分页
- CSS - 变量
- CSS - 媒体查询
- CSS - 函数
- CSS - 数学函数
- CSS - 遮罩
- CSS - 形状
- CSS - 图片样式
- CSS - 特异性
- CSS - 自定义属性
- CSS 响应式
- CSS RWD - 简介
- CSS RWD - 视口
- CSS RWD - 网格视图
- CSS RWD - 媒体查询
- CSS RWD - 图片
- CSS RWD - 视频
- CSS RWD - 框架
- CSS 工具
- CSS - 像素到EM转换器
- CSS - 颜色选择器和动画
- CSS 资源
- CSS - 有用资源
- CSS - 讨论
CSS 响应式图片
在响应式网页设计中,确保图片在所有屏幕尺寸和分辨率下都能很好地显示非常重要。通过使用`max-width`和`min-width`等属性,图片可以自动调整大小以适应不同的屏幕。在本节中,我们将学习如何使图片具有响应性,了解响应式图片的关键属性,以及如何创建一个响应式图片库。
当图片上传到网页时,它将以其默认宽度和高度显示。我们可以使用CSS更改这些尺寸,以便图片根据布局中的可用空间进行调整。一种常用的设置图片尺寸的方法是,保持图片的宽度固定(例如屏幕的50%或25%),高度将根据图片的纵横比自动设置。
为了更好的适应性,我们应该始终为宽度属性使用相对单位,例如百分比,而不是绝对值,例如像素。绝对值会限制图片的响应性。
使用width属性实现响应式图片
为了使图片根据屏幕尺寸缩放,我们需要将图片的宽度属性设置为100%,并将高度设置为auto。
img { width: 100%; height: auto; }
这样设置样式会使图片占据其父元素宽度的100%,并且高度将被调整以保持图片的纵横比。此设置允许图片随屏幕尺寸缩放。但是,在非常大的屏幕上,图片可能会超出其自然宽度,使其显得变形。
示例
在此示例中,显示的图片将根据输出窗口的屏幕尺寸进行缩放。在Tutorialspoint的HTML编译器中运行此代码,以调整输出窗口宽度并进行验证。
<!DOCTYPE html> <html> <head> <style> img { width: 100%; height: auto; } </style> </head> <body> <p> The image will cover 100% width </p> <img src="/css/images/border.png"> </body> </html>
使用max-width属性实现响应式图片
上述方法有一个缺点,在大屏幕上,图片会超出其自然尺寸而被拉伸。为了防止这种情况,我们可以使用max-width属性代替'width'属性。
img { max-width: 100%; height: auto; }
通过这种方式设置图片属性,如果需要,图片会缩小,但永远不会放大到超过其原始尺寸。
示例
在此示例中,显示的图片将根据输出窗口的屏幕尺寸缩放,但永远不会超过其自然尺寸。在Tutorialspoint的HTML编译器中运行此代码,以调整输出窗口宽度并进行验证。
<!DOCTYPE html> <html> <head> <style> img { max-width: 100%; height: auto; } </style> </head> <body> <p> The image will cover 100% width if natural width is less than output screen width </p> <img src="/css/images/border.png"> </body> </html>
CSS 图片库
CSS 图片库用于以响应式和视觉上吸引人的格式组织和显示多张图片。CSS属性可用于控制图片的布局、大小、形状、间距、跨度以及许多其他视觉效果。
CSS 网格布局是最常用的用于设计图片库的布局系统,我们可以用它以二维方式排列图片。
示例
<!DOCTYPE html> <html> <head> <style> /* Gallery container */ .gallery { display: grid; gap: 10px; padding: 10px; font-family: Arial, sans-serif; } /* style image items */ .gallery img { width: 100%; height: 100px; /* Set a same height for all images */ object-fit: fit; display: block; border-radius: 8px; border: 3px solid #ccc; transition: all 0.3s ease; } /* Spanning the first image across two rows */ .gallery img:first-child { grid-row: span 2; height: 210px; /* Double the height of regular images */ } /* Spanning the sixth image across two columns */ .gallery img:nth-child(6) { grid-column: span 2; } /* Hover effect */ .gallery img:hover { transform: scale(1.02); border-color: #555 ; } </style> </head> <body> <div class="gallery"> <img src="/w3css/images/w3css_pdfcover.jpg" alt="Gallery Image 1"> <img src="/css/images/html.png" alt="Gallery Image 2"> <img src="/css/images/css.png" alt="Gallery Image 3"> <img src="/css/images/html.png" alt="Gallery Image 4"> <img src="/css/images/css.png" alt="Gallery Image 5"> <img src="/html/images/logo.png" alt="Gallery Image 6"> </div> </body> </html>
响应式图片库
我们可以使用CSS 媒体查询来创建一个响应式图片库,该库可以根据屏幕宽度缩放和重新排列其内容。以下是一个简单的媒体查询,它定义了大屏幕和小屏幕的图片库列数。
/* 4 columns in case of large screen */ @media (min-width: 600px) { .gallery { grid-template-columns: repeat(4, 1fr); } } /* 1 column in case of small screen */ @media (max-width: 599px) { .gallery { grid-template-columns: 1fr; } }
使用媒体查询,我们还可以为用户设备的特定方向(横向或纵向)定义样式。默认值为纵向。
示例
这是一个设计响应式图片库的示例。
<!DOCTYPE html> <html> <head> <style> /* Gallery container */ .gallery { display: grid; gap: 10px; padding: 10px; font-family: Arial, sans-serif; } /* 4 columns in case of large screen */ @media (min-width: 600px) { .gallery { grid-template-columns: repeat(4, 1fr); } } /* 1 column in case of small screen */ @media (max-width: 599px) { .gallery { grid-template-columns: 1fr; } } /* Individual image items */ .gallery img { width: 100%; height: 100px; /* Set a same height for all images */ object-fit: fit; /* Ensure images fits the area */ display: block; border-radius: 8px; border: 3px solid #ccc; /* Default border color */ transition: all 0.3s ease; } /* Spanning the first image across two rows */ .gallery img:first-child { grid-row: span 2; height: 210px; /* Double the height of regular images */ } /* Spanning the sixth image across two columns */ .gallery img:nth-child(6) { grid-column: span 2; } /* Hover effect */ .gallery img:hover { transform: scale(1.02); border-color: #555 ; } </style> </head> <body> <div class="gallery"> <img src="/w3css/images/w3css_pdfcover.jpg" alt="Gallery Image 1"> <img src="/css/images/html.png" alt="Gallery Image 2"> <img src="/css/images/css.png" alt="Gallery Image 3"> <img src="/css/images/html.png" alt="Gallery Image 4"> <img src="/css/images/css.png" alt="Gallery Image 5"> <img src="/html/images/logo.png" alt="Gallery Image 6"> </div> </body> </html>
大屏幕输出
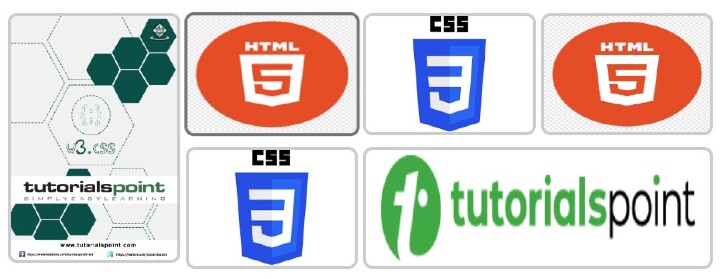
小屏幕输出
