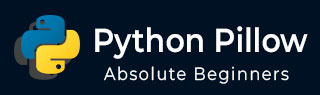
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 图像滚动
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 连接两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化遮罩滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 添加图像填充
- Python Pillow - 颜色反转
- Python Pillow 与 NumPy 结合使用
- Python Pillow 与 Tkinter 的 BitmapImage 和 PhotoImage 对象结合使用
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 添加图像填充
添加图像填充是指在图像周围添加边框。这是一种在调整图像大小而不改变其纵横比或裁剪图像的有用技术。可以在图像的顶部、底部、左侧和右侧添加填充。这在处理边缘具有重要信息的图像时尤其重要,您希望保留这些信息以进行分割等任务。
Pillow (PIL) 库在其 ImageOps 模块中提供了两个函数,pad() 和 expand(),用于向图像添加填充。
使用 ImageOps.pad() 函数填充图像
pad() 函数用于将图像调整大小并填充到指定的大小和纵横比。它允许您指定输出大小、重采样方法、背景颜色以及原始图像在填充区域中的位置。该函数的语法如下:
PIL.ImageOps.pad(image, size, method=Resampling.BICUBIC, color=None, centering=(0.5, 0.5))
其中:
image - 要调整大小和填充的图像。
size - 一个元组,指定请求的输出大小(以像素为单位),格式为 (宽度, 高度)。该函数将调整图像大小到此尺寸,同时保持纵横比。
method - 此参数确定调整大小期间使用的重采样方法。默认方法是 BICUBIC,这是一种插值类型。您可以指定 PIL 支持的其他重采样方法。常见的选项包括 NEAREST、BILINEAR 和 LANCZOS。
color - 此参数指定填充区域的背景颜色。它也支持 RGBA 元组,例如 (R, G, B, A)。如果未指定,则默认背景颜色为黑色。
centering - 此参数控制原始图像在填充版本中的位置。它指定为一个包含 0 到 1 之间两个值的元组。
示例
这是一个使用 ImageOps.pad() 函数向图像添加填充的示例。
from PIL import Image from PIL import ImageOps # Open the input image input_image = Image.open('Images/elephant.jpg') # Add padding to the image image_with_padding = ImageOps.pad(input_image, (700, 300), color=(130, 200, 230)) # Display the input image input_image.show() # Display the image with the padding image_with_padding.show()
输入
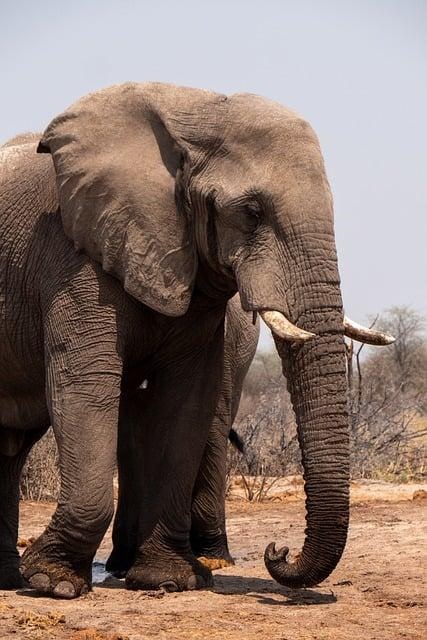
输出
添加填充后调整大小后的输出图像:
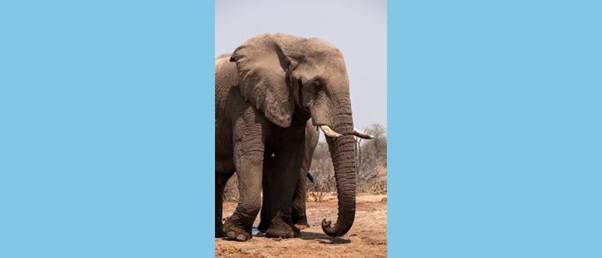
使用 ImageOps.expand() 函数添加边框
expand() 函数在图像周围添加指定宽度和颜色的边框。它可用于创建装饰性框架或强调图像内容。其语法如下:
PIL.ImageOps.expand(image, border=0, fill=0)
image - 要扩展边框的图像。
border - 要添加的边框宽度(以像素为单位)。它决定图像周围边框的宽度。默认值为 (0)。
fill - 像素填充值,表示边框的颜色。默认值为 0,对应于黑色。您可以使用适当的颜色值指定填充颜色。
示例
这是一个使用 ImageOps.expand() 函数向图像添加填充的示例。
from PIL import Image, ImageOps # Open the input image input_image = Image.open('Images/Car_2.jpg') # Add padding of 15-pixel border image_with_padding = ImageOps.expand(input_image, border=(15, 15, 15, 15), fill=(255, 180, 0)) # Display the input image input_image.show() # Display the output image with padding image_with_padding.show()
输入
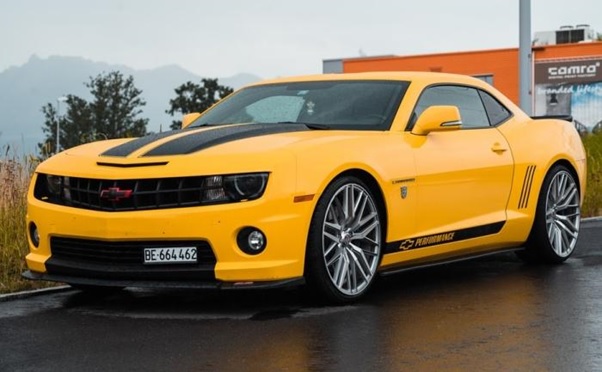
输出
添加填充后的输出图像:
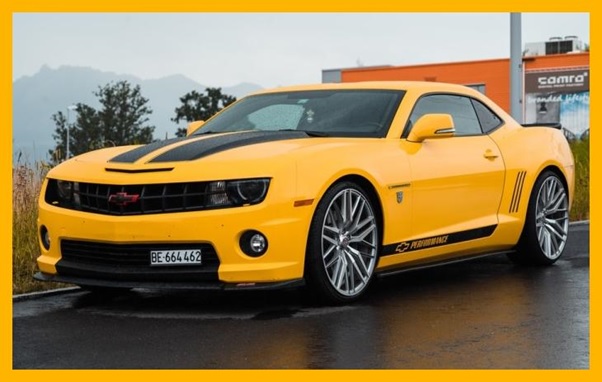