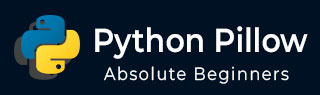
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境搭建
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 图像缩放
- Python Pillow - 图片翻转和旋转
- Python Pillow - 图像裁剪
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 图像剪切和粘贴
- Python Pillow - 图像滚动
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 非锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow - 使用 NumPy 进行机器学习
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 图片翻转和旋转
翻转和旋转图像是在图像处理中常用的基本任务,常用于增强可见性、校正方向或实现特定效果。Python Pillow 库提供了简单的方法来对图像执行这些操作。
翻转图像
翻转图像是一种图像处理操作,它涉及沿指定轴反转图像的方向。此操作可以水平进行(即从左到右)或垂直进行(即从上到下)。在数字图像处理中,翻转通常用于各种目的,例如镜像、校正相机方向或实现艺术效果。
在图像翻转的上下文中,主要有两种类型:水平翻转和垂直翻转。
水平翻转(从左到右)- 在水平翻转中,每一行像素都反转,有效地从左到右镜像图像。
垂直翻转(从上到下)- 在垂直翻转中,每一列像素都反转,有效地从上到下镜像图像。
这些可以通过使用 Pillow 库 Image 模块的 transpose() 方法来实现。
使用 transpose() 方法翻转图像
Pillow 中的 transpose() 方法用于操作图像的方向。它允许我们通过指定要应用的转换操作类型来对图像执行各种变换,例如旋转和镜像。
以下是 transpose() 方法的语法和参数。
PIL.Image.transpose(method)
其中,
method - 要应用的转换方法。它可以取以下值之一:
Image.Transpose.ROTATE_90 - 将图像逆时针旋转 90 度。
Image.Transpose.ROTATE_180 - 将图像旋转 180 度。
Image.Transpose.ROTATE_270 - 将图像顺时针旋转 90 度。
Image.Transpose.FLIP_LEFT_RIGHT - 水平翻转图像(即从左到右)。
Image.Transpose.FLIP_TOP_BOTTOM - 垂直翻转图像(即从上到下)。
以下是本章所有示例中使用的输入图像。
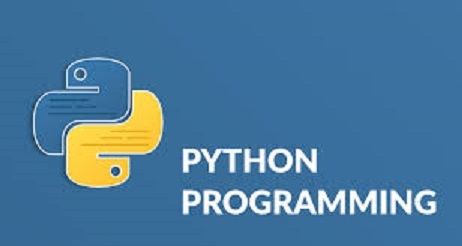
示例
在这个示例中,我们使用 transpose() 方法将输入图像逆时针旋转 90 度。
from PIL import Image #Open an image image = Image.open("Images/image_.jpg") #Rotate the image 90 degrees counterclockwise rotated_image = image.transpose(Image.Transpose.ROTATE_90) #Save or display the rotated image rotated_image.save("output Image/rotated_image.jpg") open_rotated_image = Image.open("output Image/rotated_image.jpg") open_rotated_image.show()
输出
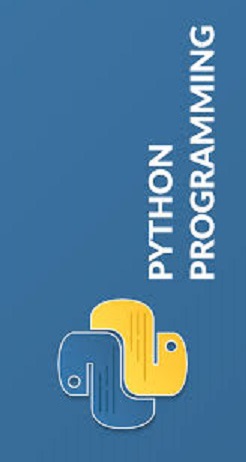
示例
在这个示例中,我们使用 transpose() 方法水平翻转输入图像。
from PIL import Image #Open an image image = Image.open("Images/image_.jpg") #Flip the image horizontally (left to right) flipped_image = image.transpose(Image.Transpose.FLIP_LEFT_RIGHT) #Save or display the rotated image flipped_image.save("output Image/flipped_image.jpg") open_flipped_image = Image.open("output Image/flipped_image.jpg") open_flipped_image.show()
输出
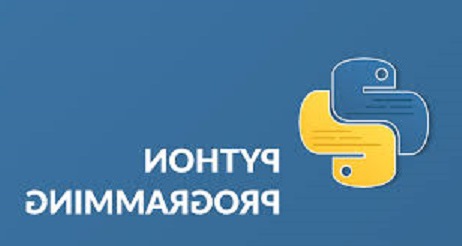
示例
在这个示例中,我们使用 transpose() 方法和 FLIP_TOP_BOTTOM 参数垂直翻转输入图像。
from PIL import Image #Open an image image = Image.open("Images/image_.jpg") #Flip the image horizontally (left to right) vflipped_image = image.transpose(Image.Transpose.FLIP_TOP_BOTTOM) #Save or display the rotated image vflipped_image.save("output Image/vflipped_image.jpg") open_flipped_image = Image.open("output Image/vflipped_image.jpg") open_flipped_image.show()
输出
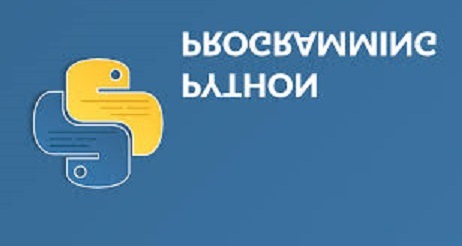
旋转图像
在 Pillow(Python Imaging Library)中旋转图像是指通过特定角度更改图像方向的过程。这对于各种目的都很有用,例如校正照片的方向、创建特殊效果或对齐图像。
Pillow 提供了 rotate() 方法来执行此操作。以下是关于在 Pillow 中旋转图像我们需要了解的几点。
按角度旋转 - rotate() 方法允许我们指定要旋转图像的角度(以度为单位)。正角度逆时针旋转图像,负角度顺时针旋转图像。
画布扩展 - 默认情况下,当我们旋转图像时,它可能无法完全适合原始图像画布,这可能导致裁剪。然后,我们可以使用 expand 参数来确保旋转后的图像适合画布,而不会被裁剪。
使用 rotate() 方法旋转图像
Image 模块中的 rotate() 方法用于执行给定输入图像的旋转。
以下是 rotate() 方法的基本语法。
PIL.Image.rotate(angle, expand=False, resample=3)
其中,
angle - 此参数指定旋转角度(以度为单位)。正值逆时针旋转图像,负值顺时针旋转图像。
expand (可选) - 如果设置为 True,它允许扩展图像画布以确保整个旋转后的图像适合其中。如果设置为“False”(默认值),则图像将被裁剪以适合原始画布。
resample (可选) - 可选的重采样滤镜。默认值为“3”,对应于抗锯齿高质量滤镜。我们可以从各种重采样滤镜中选择,例如“Image.NEAREST”、“Image.BOX”、“Image.BILINEAR”、“Image.HAMMING”、“Image.BICUBIC”、“Image.LANCZOS”等。
以下是本章所有示例中使用的输入图像。
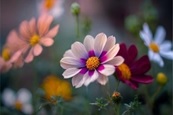
示例
在这个示例中,我们使用 rotate() 方法将给定的输入图像旋转 45 度。
from PIL import Image #Open an image image = Image.open("Images/flowers.jpg") #Rotate the image by 45 degrees counterclockwise rotated_image = image.rotate(45) #Save the rotated image rotated_image.save("output.jpg") rotated_image.show()
输出
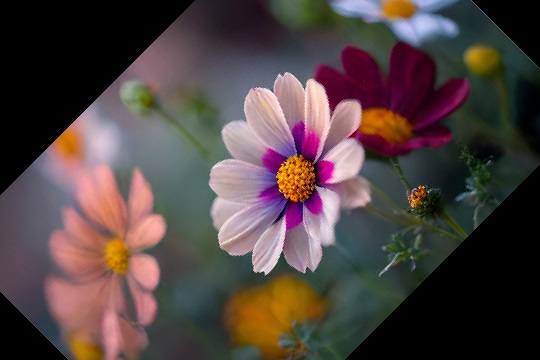
示例
在这个示例中,我们旋转输入图像,确保旋转后的图像适合画布,而不会被裁剪。这可以通过将 expand 参数设置为 True 来实现。
from PIL import Image #Open an image image = Image.open("Images/flowers.jpg") #Rotate the image by 45 degrees and expand the canvas to fit the entire rotated image rotated_image = image.rotate(45, expand=True) rotated_image.show()
输出
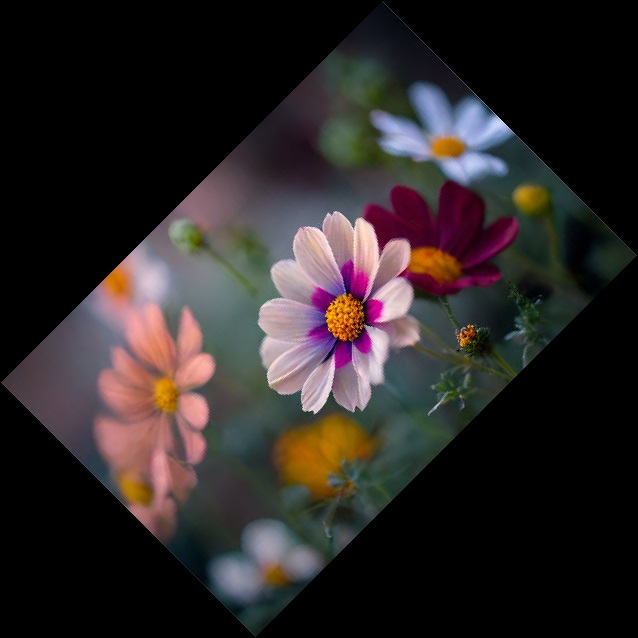
示例
在这个示例中,我们通过将负值 -45 度作为参数传递给 rotate() 方法,将图像逆时针旋转。
from PIL import Image #Open an image image = Image.open("Images/flowers.jpg") #Rotate the image by 45 degrees and expand the canvas to fit the entire rotated image rotated_image = image.rotate(-45, expand=True) rotated_image.show()
输出
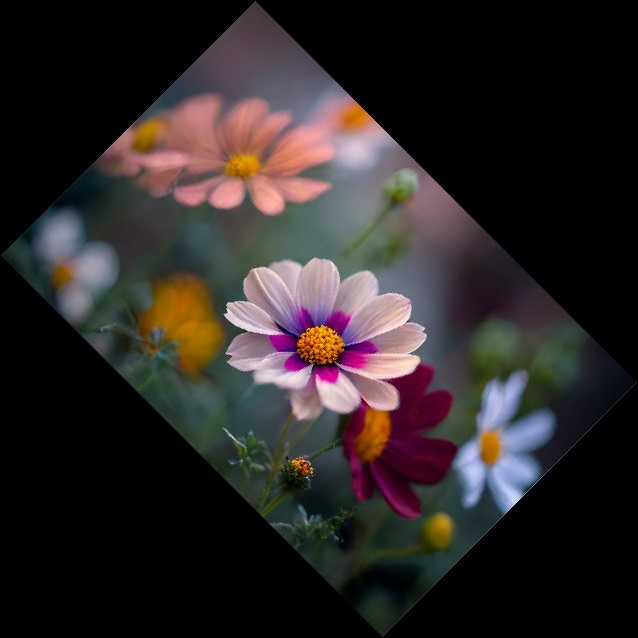