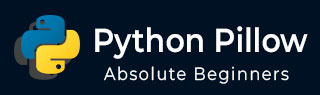
- Python Pillow 教程
- Python Pillow——首页
- Python Pillow——概述
- Python Pillow——环境搭建
- 基本图像操作
- Python Pillow——图像处理
- Python Pillow——图像缩放
- Python Pillow——图像翻转和旋转
- Python Pillow——图像裁剪
- Python Pillow——为图像添加边框
- Python Pillow——识别图像文件
- Python Pillow——图像合并
- Python Pillow——图像剪切和粘贴
- Python Pillow——图像滚动
- Python Pillow——在图像上添加文字
- Python Pillow——ImageDraw 模块
- Python Pillow——连接两张图像
- Python Pillow——创建缩略图
- Python Pillow——创建水印
- Python Pillow——图像序列
- Python Pillow 颜色转换
- Python Pillow——图像颜色
- Python Pillow——创建彩色图像
- Python Pillow——将颜色字符串转换为 RGB 颜色值
- Python Pillow——将颜色字符串转换为灰度值
- Python Pillow——通过更改像素值来更改颜色
- 图像处理
- Python Pillow——降噪
- Python Pillow——更改图像模式
- Python Pillow——图像合成
- Python Pillow——处理 Alpha 通道
- Python Pillow——应用透视变换
- 图像滤镜
- Python Pillow——为图像添加滤镜
- Python Pillow——卷积滤镜
- Python Pillow——图像模糊
- Python Pillow——边缘检测
- Python Pillow——图像浮雕
- Python Pillow——增强边缘
- Python Pillow——非锐化掩膜滤镜
- 图像增强和校正
- Python Pillow——增强对比度
- Python Pillow——增强锐度
- Python Pillow——增强色彩
- Python Pillow——校正色彩平衡
- Python Pillow——去除噪点
- 图像分析
- Python Pillow——提取图像元数据
- Python Pillow——识别颜色
- 高级主题
- Python Pillow——创建动画 GIF
- Python Pillow——批量处理图像
- Python Pillow——转换图像文件格式
- Python Pillow——为图像添加填充
- Python Pillow——颜色反转
- Python Pillow 与 NumPy
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow——图像混合
- Python Pillow 有用资源
- Python Pillow——快速指南
- Python Pillow——函数参考
- Python Pillow——有用资源
- Python Pillow——讨论
Python Pillow——图像处理
使用 Python Pillow 库处理图像的基本操作包括打开、写入、显示和保存图像。Python Pillow 库提供了广泛的图像处理工具,为这些基本操作提供了简单的方法和可定制的选项。
在本教程中,您将学习使用 Pillow 库处理图像的几个重要方面,包括读取、显示、写入和保存图像。
读取图像
读取图像指的是打开和读取图像文件,并使其在 Python 程序中可用于操作和处理的过程。
在 Pillow 中,Image 模块提供open() 函数来加载给定的输入图像。当我们调用Image.open() 时,它会读取指定的图像文件,解码图像并创建一个代表该图像的 Pillow Image 对象。然后,此对象可用于各种图像处理任务。
使用 open() 函数读取图像
Image.open() 函数能够加载不同的图像格式,例如 JPEG、PNG、GIF、BMP、TIFF、ICO 等。
以下是此函数的语法和参数。
PIL.Image.open(fp, mode='r', formats = None)
其中,
fp——图像的文件名或路径或 URL(字符串格式)。
mode (可选)——mode 参数用于设置图像的打开模式,应将其设置为r。
formats (可选)——要加载的格式列表或元组。这可以用作要加载的文件格式的限制。
此函数返回图像作为输出。在这里,为了显示图像,我们必须使用Image 模块的show() 函数。
示例
在这个例子中,我们通过字符串格式指定图像路径来加载图像,并将模式定义为r。
from PIL import Image #Load an image loaded_image = Image.open("Images/butterfly.jpg", mode = 'r') loaded_image.show() #Saving the image img.save("Output_Images/reading.png")
待使用的图像
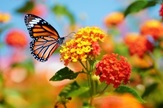
输出
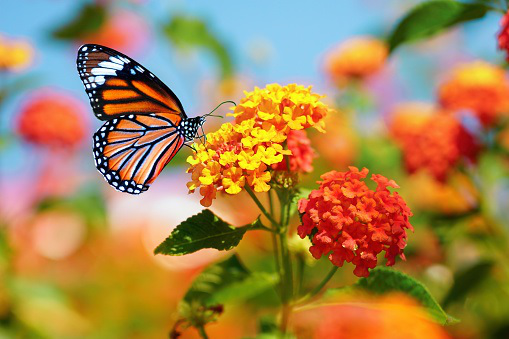
示例
在这个例子中,我们从图像 URL 加载图像。为此,我们必须使用urllib.request() 模块来读取图像 URL。
我们使用urllib.request 模块的urlretrieve() 函数从 URL 下载图像,并将其保存到本地文件系统中,并指定文件名。
import urllib.request from PIL import Image url = "https://tutorialspoint.com/images/logo.png" #Download the image using urllib urllib.request.urlretrieve(url, "image.png") #Open the downloaded image in PIL loading_image = Image.open("image.png", mode = 'r') #Show the image loading_image.show()
输出
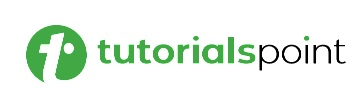
示例
在这个例子中,我们传入图像名称和formats = None 作为输入参数。
from PIL import Image #load the image loading_image = Image.open("pillow.jpg", formats = None) #Show the image loading_image.show()
输出
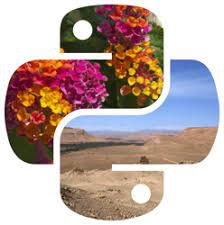
写入图像
写入图像无非是从头创建新的图像文件或修改现有图像(例如绘图、像素操作和合成)。然后可以使用其他 Pillow 函数和方法进一步处理生成的图像或将其保存到磁盘。
使用 new() 方法写入图像
您可以使用 Image 模块中的new() 方法创建一个具有指定模式和大小的空白图像。这是一种生成具有所需特征的空白或未初始化图像的方法。
以下是Image.new() 的语法:
PIL.Image.new(mode, size, color)
其中,
mode——此参数指定图像的颜色模式,例如彩色图像的“RGB”或灰度图像的“L”。可用的模式取决于 Pillow 的版本和底层图像库的功能(例如,“RGB”、“RGBA”、“L”、“CMYK”等)。
size——此参数是一个元组,指定图像的宽度和高度(以像素为单位)。例如,要创建一个 300x200 像素的图像,我们可以使用 '(300, 200)'。
color' (可选)——此参数指定图像的初始颜色。这是一个可选参数,可以是单个颜色值或多通道图像的元组。对于大多数模式,默认颜色为黑色。
示例
在这个例子中,我们使用 Pillow 库中Image 模块的new() 方法创建一个黑色新图像。
from PIL import Image #Create a new 300x200 pixel RGB image filled with white img = Image.new('RGB', (300, 200), color='black') img.show()
输出
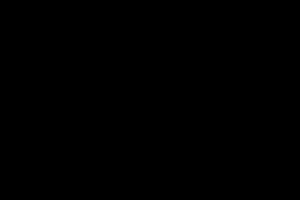
示例
在这个例子中,我们使用 Pillow 的Image 模块中的new() 方法创建一个宽度为 600,高度为 600,颜色为蓝色的新图像。
from PIL import Image #Create a new 300x200 pixel RGB image filled with white img = Image.new('RGB', (500, 200), color='blue') img.show()
输出
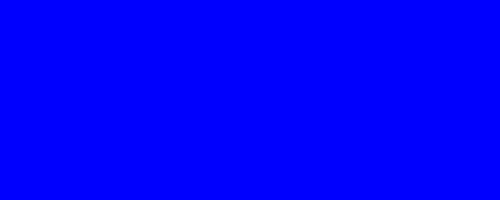
显示图像
显示图像是在屏幕上渲染图像以便您可以查看它。Pillow 提供 show() 方法,使用系统的默认图像查看器来显示图像。
使用 show() 方法显示图像
Image 模块中的show() 方法不需要任何参数或自变量。这很简单。
语法
以下是show() 函数的语法:
PIL.Image.show()
示例
在这个例子中,我们使用Image 模块的show() 函数显示open() 函数的输出。
from PIL import Image #Open an image image = Image.open("Images/hand writing.jpg") #Display the image using the default image viewer image.show()
输出
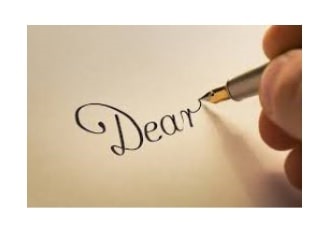
在 Jupyter Notebook 环境中显示图像
如果我们在 Jupyter Notebook 环境中工作,则可以使用 IPython 的display() 函数直接在 Notebook 中显示图像。
以下是display() 函数的语法和参数:
IPython.display.display(image_name)
其中,
image_name——这是要显示的图像的名称。
示例
在这个例子中,我们使用IPython.display 模块的display() 函数显示图像。
from PIL import Image from IPython.display import display #Load the image loading_image = Image.open("Images/tutorialspoint.png") #Show the image display(loading_image)
输出

在 GUI 应用程序中显示
如果我们正在构建图形用户界面 (GUI) 应用程序,则可以使用像 Tkinter 这样的 GUI 工具包(用于桌面应用程序)或像 Flask 或 Django 这样的 Web 框架(用于 Web 应用程序)在用户界面中显示图像。
示例
在这个例子中,我们使用 PIL 模块的 ImageTk 方法来显示图像输出。
import tkinter as tk from PIL import Image, ImageTk def display_image(image_path): root = tk.Tk() root.title("Image Viewer") image = Image.open(image_path) photo = ImageTk.PhotoImage(image) label = tk.Label(root, image=photo) label.image = photo label.pack() root.mainloop() display_image("Images/tutorialspoint.png")
输出
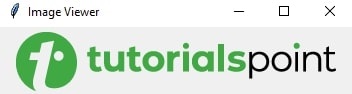
保存图像
在 Pillow 中保存图像是指将打开的图像保存到内存中,并使其在 Python 程序中可用于操作和处理。
Pillow 库的Image 模块提供save() 方法,允许您将指定的图像对象保存到内存或本地系统的指定位置。
使用 save() 函数保存图像
save() 方法允许我们将打开的图像保存为不同的文件格式,例如 JPG、PNG、PDF 等。此函数指定了保存图像的各种选项,包括格式、质量和其他参数。
以下是此函数的语法和参数。
PIL.Image.save(file, format=None, **params)
其中,
file——将保存图像到的文件路径或类文件对象。
format(可选)——保存图像的格式。如果未指定,Pillow 将尝试从文件扩展名确定格式。
params(可选)——取决于我们保存图像的格式的其他参数。
此函数将图像保存到指定位置,并使用指定的文件名。
示例
在这个例子中,我们使用 Pillow 库Image 模块的open() 函数打开指定的图像。然后通过将路径作为输入参数传递给save() 函数,将打开的图像保存到指定位置。
from PIL import Image loading_image = Image.open("pillow.jpg") #Show the image loading_image.show() #Save the image loading_image.save("Images/saved_image.jpg") print("Image saved")
输出
加载的图像:
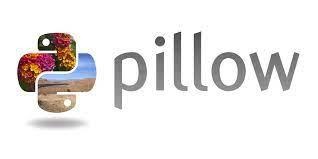
保存的图像:
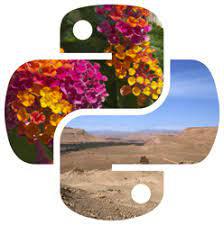
示例
在此示例中,我们使用其他可选参数将图像保存到指定位置。
from PIL import Image #Load the image loading_image = Image.open("Images/tutorialspoint.png") #Show the image loading_image.show() #Save the image loading_image.save("output Image/save_outputimg.png", format = "PNG", quality = 200) print("Image saved")
输出
加载的图像:
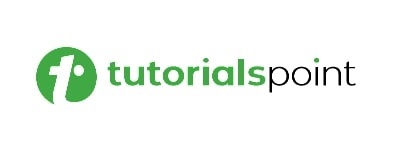
保存的图像:
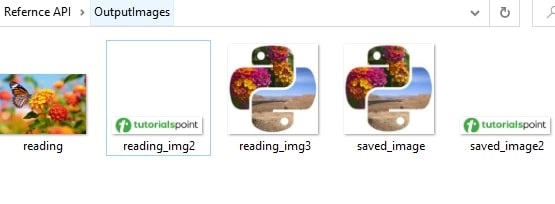
我们可以使用save() 方法以各种格式保存图像,并根据我们的特定用例需要控制质量和其他特定于格式的选项。