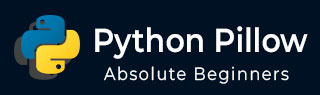
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 图像缩放
- Python Pillow - 图像翻转和旋转
- Python Pillow - 图像裁剪
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 图像剪切和粘贴
- Python Pillow - 图像滚动
- Python Pillow - 在图像上添加文字
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像颜色
- Python Pillow - 创建彩色图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow - 使用 NumPy 进行机器学习
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 边缘检测
边缘检测是使用数学算法识别图像中这些边界或轮廓的过程。边缘检测旨在定位图像中强度突然变化的点,这通常对应于两个区域之间的边缘或边界。一般来说,边缘定义为图像中两个不同区域之间的边界或轮廓。这些区域在强度、颜色、纹理或任何其他视觉特征方面可能有所不同。边缘可以表示图像中的重要特征,例如物体边界、形状和纹理。
边缘检测是许多图像处理应用程序中的一个重要步骤,例如目标识别、分割、跟踪和增强。在本教程中,我们将学习使用 Python Pillow 库检测图像边缘的不同方法。
将边缘检测滤镜应用于图像
可以使用ImageFilter.FIND_EDGES滤镜检测图像中的边缘,这是 Pillow 库当前版本中用于检测图像边缘的内置滤镜选项之一。
以下是检测图像边缘的步骤:
使用 Image.open() 函数加载输入图像。
将 filter() 函数应用于加载的 Image 对象,并将 ImageFilter.FIND_EDGES 作为参数提供给该函数。该函数将返回检测到的边缘作为 PIL.Image.Image 对象。
示例
以下示例演示如何使用ImageFilter.FIND_EDGES滤镜内核和 filter() 方法来检测图像中的边缘。
from PIL import Image, ImageFilter # Open the image image = Image.open('Images/compass.jpg') # Apply edge detection filter edges = image.filter(ImageFilter.FIND_EDGES) # Display the original image image.show() # Display the edges-detected image edges.show()
输入图像
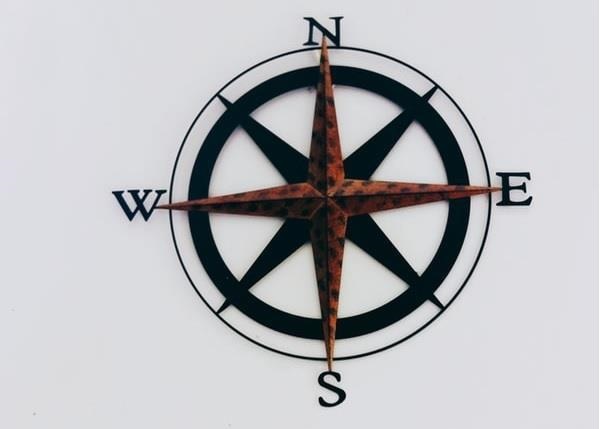
输出图像
检测到的边缘:
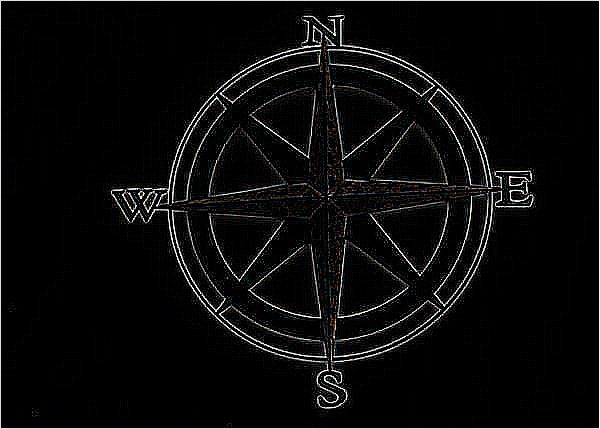
示例
这是一个使用 ImageFilter.FIND_EDGES 滤镜内核检测图像边缘的示例。然后,它应用 ImageFilter.MaxFilter() 类来平滑检测到的边缘。
import matplotlib.pyplot as plt from PIL import Image, ImageFilter # Open the image and convert it to grayscale im = Image.open('Images/compass.jpg').convert('L') # Detect edges edges = im.filter(ImageFilter.FIND_EDGES) # Make fatter edges fatEdges = edges.filter(ImageFilter.MaxFilter) # Make very fat edges veryFatEdges = edges.filter(ImageFilter.MaxFilter(7)) # Create subplots for displaying the images fig, axes = plt.subplots(2, 2, figsize=(12, 10)) ax = axes.ravel() # Original image ax[0].imshow(im, cmap='gray') ax[0].set_title('Original') # Detected edges ax[1].imshow(edges, cmap='gray') ax[1].set_title('Edges') # Fatter edges ax[2].imshow(fatEdges, cmap='gray') ax[2].set_title('Fatter Edges') # Very fat edges ax[3].imshow(veryFatEdges, cmap='gray') ax[3].set_title('Very Fat Edges') for ax in axes.flatten(): ax.axis('off') # Display the images plt.tight_layout() plt.show()
输出图像
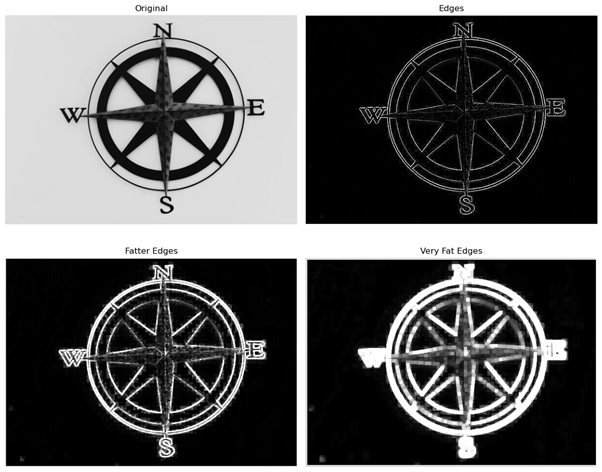
使用 ImageFilter.Kernel() 类检测边缘
此类用于创建卷积内核。
在这种方法中,我们将定义一个具有某些指定内核值 (-1, -1, -1, -1, 8, -1, -1, -1, -1) 的 3X3 卷积内核。我们将对图像执行边缘检测。
示例
以下示例演示如何使用卷积矩阵和 ImageFilter.kernel() 类对图像执行边缘检测。
from PIL import Image, ImageFilter # Open the image and convert it to grayscale image = Image.open('Images/compass.jpg').convert('L') # Calculate edges using a convolution matrix kernel = ImageFilter.Kernel((3, 3), (-1, -1, -1, -1, 8, -1, -1, -1, -1), scale=1, offset=0) edges = image.filter(kernel) # Display the original image image.show() # Display the edges edges.show()
输入图像
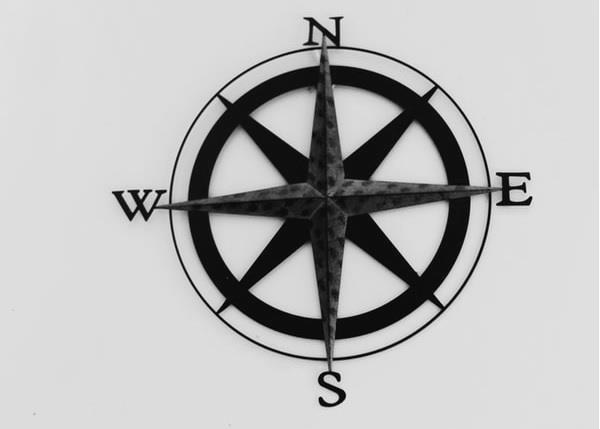
输出图像
检测到的边缘:
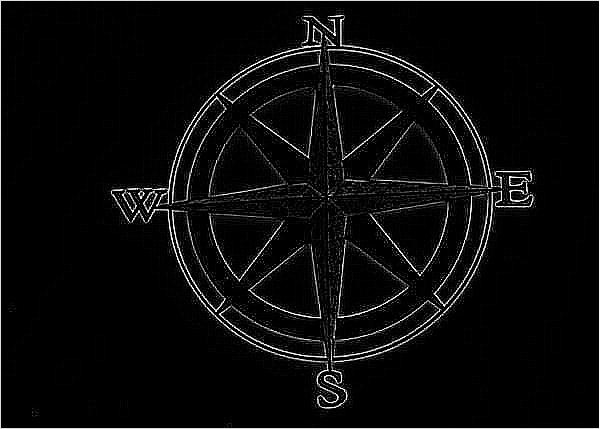
广告