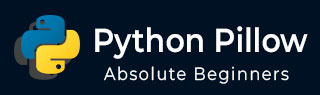
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 图像缩放
- Python Pillow - 图像翻转和旋转
- Python Pillow - 图像裁剪
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 图像剪切和粘贴
- Python Pillow - 图像滚动
- Python Pillow - 在图像上添加文字
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 创建彩色图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 颜色识别
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow - 使用 NumPy 进行机器学习
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 颜色识别
在图像处理和分析的背景下,识别颜色是指识别、分类和提取不同图像上的颜色信息的过程。它涉及分析像素数据以确定图像中特定的颜色或颜色模式。
Python Pillow 库为此目的提供了有价值的工具。本教程探讨了两个基本函数,即getcolors()和getdata(),它们在有效分析图像中存在的颜色信息方面起着至关重要的作用。
使用 getcolors() 函数识别颜色
getcolors() 函数返回图像中使用的颜色列表,颜色以图像的模式表示。例如,在 RGB 图像中,该函数返回一个包含红色、绿色和蓝色颜色值的元组。对于基于调色板 (P) 的图像,它返回调色板中颜色的索引。使用此函数的语法如下:
Image.getcolors(maxcolors=256)
其中,maxcolors 参数用于指定要检索的最大颜色数。它返回一个未排序的元组列表,每个元组包含出现次数和相应的颜色像素值。如果超过此 maxcolors 限制,则该方法返回 None(默认限制:256 种颜色)。
示例
此示例使用Image.getcolors() 函数识别图像中最常见的颜色。它从图像中提取前 10 种最常见的颜色,并创建一个调色板来可视化和显示这些颜色及其各自的计数。
from PIL import Image, ImageDraw # Open an image input_image = Image.open('Images/colorful-shapes.jpg') # Get the palette of the top 10 most common colors palette = sorted(input_image.getcolors(maxcolors=100000), reverse=True)[:10] cols = 2 rows = ((len(palette) - 1) // cols) + 1 cellHeight = 70 cellWidth = 200 imgHeight = cellHeight * rows imgWidth = cellWidth * cols result_image = Image.new("RGB", (imgWidth, imgHeight), (0, 0, 0)) draw = ImageDraw.Draw(result_image) for idx, (count, color) in enumerate(palette): y0 = cellHeight * (idx // cols) y1 = y0 + cellHeight x0 = cellWidth * (idx % cols) x1 = x0 + (cellWidth // 4) draw.rectangle([x0, y0, x1, y1], fill=color, outline='black') draw.text((x1 + 1, y0 + 10), f"Count: {count}", fill='white') # Display the input image input_image.show('Input Image') # Display the color chart result_image.show('Output identified Colors')
输入图像
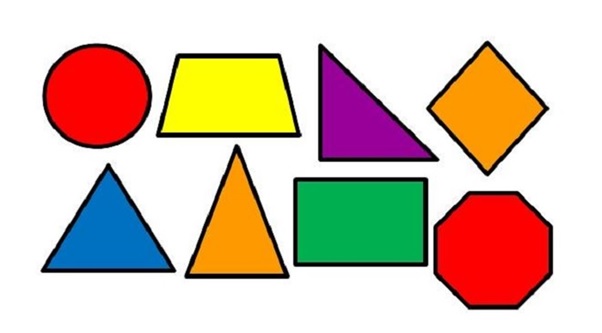
输出识别的颜色
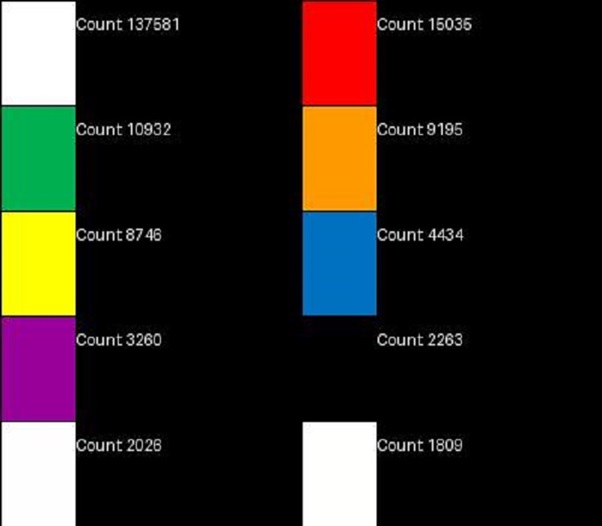
需要注意的是,当图像中的颜色数量大于 maxcolors 参数时,getcolors() 函数返回 None。它主要适用于“RGB”图像,这可能并非适用于所有用例。
对于需要更多灵活性或处理不同颜色模式的图像的情况,结合使用Image.getdata() 函数和其他 Python 库可能更方便。
使用 getdata() 函数识别颜色
getdata() 函数是 Pillow Image 模块中的另一个函数,它允许用户访问图像的内容作为包含像素值的序列对象。序列对象是扁平化的,这意味着每一行的值都直接跟在零行的值之后,依此类推。虽然此方法返回的序列对象是 Pillow 的内部数据类型,但它只能支持某些序列操作,例如 Python 列表,以便更方便地进行操作和打印。以下是函数的语法:
Image.getdata(band=None)
参数band 用于指定要返回的波段(默认值:所有波段)。要检索单个波段,请提供索引值(例如,从“RGB”图像中获取“R”波段,则提供 0)。该函数返回一个类似序列的对象,可以将其转换为更熟悉的数据结构以进行进一步处理。
示例
这是一个示例,它使用 Image.getdata() 函数识别图像中的颜色并计算每种颜色的出现次数。
from PIL import Image from collections import defaultdict # Open an imagefrom PIL import Image from collections import defaultdict # Open an image im = Image.open('Images/sea1.jpg') # Create a defaultdict to store color counts colors = defaultdict(int) # Iterate through the image pixels and count each color for pixel in im.getdata(): colors[pixel] += 1 # Sort the colors by count in descending order ordered_colors = sorted(colors.items(), key=lambda x: x[1], reverse=True) # Print the top 10 colors and their counts for color, count in ordered_colors[:10]: print(f"Color: {color}, Count: {count}") from PIL import Image from collections import defaultdict # Open an image im = Image.open('Images/sea1.jpg') # Create a defaultdict to store color counts colors = defaultdict(int) # Iterate through the image pixels and count each color for pixel in im.getdata(): colors[pixel] += 1 # Sort the colors by count in descending order ordered_colors = sorted(colors.items(), key=lambda x: x[1], reverse=True) # Print the top 10 colors and their counts for color, count in ordered_colors[:10]: print(f"Color: {color}, Count: {count}") im = Image.open('Images/sea1.jpg') # Create a defaultdict to store color counts colors = defaultdict(int) # Iterate through the image pixels and count each color for pixel in im.getdata(): colors[pixel] += 1 # Sort the colors by count in descending order ordered_colors = sorted(colors.items(), key=lambda x: x[1], reverse=True) # Print the top 10 colors and their counts for color, count in ordered_colors[:10]: print(f"Color: {color}, Count: {count}")
输出
Color: (255, 255, 255), Count: 82 Color: (0, 33, 68), Count: 73 Color: (0, 74, 139), Count: 71 Color: (0, 78, 144), Count: 69 Color: (0, 77, 143), Count: 62 Color: (0, 63, 107), Count: 59 Color: (0, 34, 72), Count: 56 Color: (0, 36, 72), Count: 52 Color: (0, 30, 58), Count: 51 Color: (1, 26, 56), Count: 51
示例
下面的示例使用 Image.getdata() 函数和 Python collections 库来识别图像中最常见的颜色并计算该颜色的出现次数。
from PIL import Image from collections import Counter # Open an image image = Image.open('Images/Road.jpg') # Get a Counter dictionary of colors and their frequencies colors = Counter(image.getdata()) # Get a set of unique colors unique_colors = set(colors) # Get the number of unique colors num_unique_colors = len(unique_colors) # Get the color with the highest frequency most_frequent_color = max(colors, key=colors.get) print(f"Unique Colors: {num_unique_colors}") print(f"Most Frequent Color: {most_frequent_color} with a frequency of {colors[most_frequent_color]}")
输出
Unique Colors: 18323 Most Frequent Color: (26, 27, 31) with a frequency of 5598