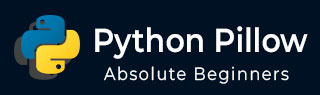
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow 与 NumPy 的结合使用
- Python Pillow 与 Tkinter 的 BitmapImage 和 PhotoImage 对象结合使用
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 使用颜色创建图像
什么是使用颜色创建图像?
在 Pillow(Python Imaging Library,现在称为 Pillow)中使用颜色创建图像,涉及创建填充特定颜色的新图像。在 Pillow 中使用颜色创建图像,需要生成特定大小的图像并用所需颜色填充它。此过程允许我们生成纯色图像,这对于创建背景、占位符或简单图形等各种用途非常有用。
在 Pillow 中,我们有名为 `new()` 的方法,用于使用颜色创建图像。在开始时,我们已经看到了 `Image` 模块中可用的 `new()` 方法的语法和参数。
使用定义的颜色创建图像需要遵循几个步骤。让我们逐一查看它们。
导入必要的模块
要使用 Pillow,我们需要导入所需的模块,通常是 `Image` 和 `ImageDraw`。`Image` 模块提供用于创建和操作图像的函数,而 `ImageDraw` 用于在图像上绘制形状和文本。
定义图像大小和颜色
确定我们要创建的图像的尺寸(即宽度和高度),并指定要使用的颜色。颜色可以通过多种方式定义,例如 RGB 元组或颜色名称。
使用指定的大小和颜色创建一个新图像
使用 `Image.new()` 方法创建一个新图像。我们可以指定图像模式,可以是“RGB”、“RGBA”、“L”(灰度)以及其他模式,具体取决于我们的需求。我们还可以为图像提供大小和颜色。
可选:在图像上绘制
如果我们想向图像添加形状、文本或其他元素,则可以使用 `ImageDraw` 模块。这允许我们使用各种方法(如 `draw.text()`、`draw.rectangle()` 等)在图像上绘制。
保存或显示图像
我们可以使用 `save()` 方法将创建的图像保存到特定格式(例如 PNG、JPEG)的文件中。或者,我们可以使用 `show()` 方法显示图像,该方法会在默认图像查看器中打开图像。
示例
在这个示例中,我们使用 `Image` 模块的 `new()` 方法创建一个纯红色图像。
from PIL import Image, ImageDraw #Define image size (width and height) width, height = 400, 300 #Define the color in RGB format (e.g., red) color = (255, 0, 0) #Red #Create a new image with the specified size and color image = Image.new("RGB", (width, height), color) #Save the image to a file image.save("output Image/colored_image.png") #Show the image (opens the default image viewer) image.show()
输出
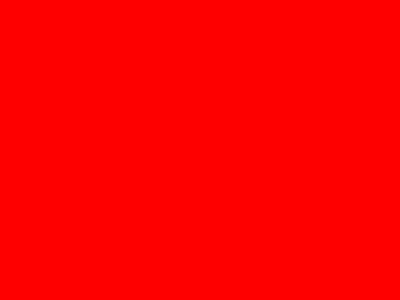
示例
在这个示例中,我们使用了可选功能,即使用 `ImageDraw` 模块的 `Draw()` 方法添加文本。
from PIL import Image, ImageDraw #Define image size (width and height) width, height = 400, 300 #Define the color in RGB format (e.g., red) color = (255, 0, 0) #Red #Create a new image with the specified size and color image = Image.new("RGB", (width, height), color) #Optional: If you want to draw on the image, use ImageDraw draw = ImageDraw.Draw(image) draw.text((10, 10), "Hello, Welcome to Tutorialspoint", fill=(255, 255, 255)) #Draw white text at position (10, 10) #Save the image to a file image.save("output Image/colored_image.png") #Show the image (opens the default image viewer) image.show()
输出
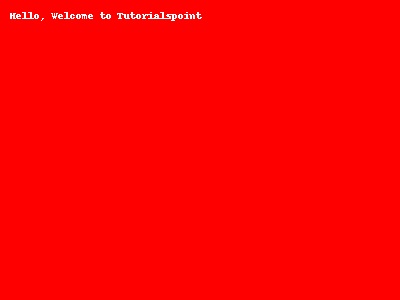