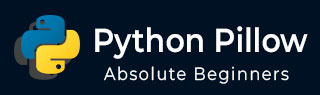
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 处理图像
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 连接两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 逆滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强颜色
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow - 使用 NumPy 进行机器学习
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 创建动画 GIF
GIF(图形交换格式)是一种位图图像格式,由 CompuServe(一家在线服务提供商)的一个团队在美籍计算机科学家史蒂夫·威尔海特(Steve Wilhite)的领导下开发。GIF 最初并非设计为动画媒介。然而,它能够在一个文件中存储多张图像,这使得它成为表示动画序列帧的合乎逻辑的选择。为了支持动画的呈现,GIF89a 规范引入了图形控制扩展(GCE)。此扩展允许指定每个帧的时间延迟,从而有效地允许从一系列图像创建视频剪辑。
在动画 GIF 中,每个帧都由其自己的 GCE 引入,指定在绘制帧后应发生的时间延迟。此外,在文件开头定义的全局信息用作所有帧的默认设置,简化了动画设置和行为的管理。
Python 的 Pillow 库可以读取 GIF87a 和 GIF89a 格式的 GIF 文件。默认情况下,它以 GIF87a 格式写入 GIF 文件,除非使用了 GIF89a 功能或输入文件已经是 GIF89a 格式。保存的文件使用 LZW 编码。
使用 Python Pillow 创建动画 GIF
可以使用 Pillow 的 Image.save() 函数创建动画 GIF。以下是调用 save() 函数以保存 GIF 文件时的语法和可用选项:
语法
Image.save(out, save_all=True, append_images=[im1, im2, ...])
选项
save_all - 如果设置为 true,则保存图像的所有帧。否则,仅保存多帧图像的第一帧。
append_images - 此选项允许将图像列表附加为附加帧。列表中的图像可以是单帧或多帧图像。此功能支持 GIF、PDF、PNG、TIFF 和 WebP 格式,以及 ICO 和 ICNS 格式。当提供相关大小的图像时,它们将用于代替缩小主图像。
include_color_table - 确定是否包含本地颜色表。
interlace - 指定图像是否交错。默认情况下,启用交错,除非图像的宽度或高度小于 16 像素。
disposal - 指示在显示图形后如何处理图形。可以将其设置为 0(未指定处理)、1(不处理)、2(恢复为背景颜色)或 3(恢复为先前内容)等值。您可以为常量处理传递单个整数,或传递列表/元组以分别为每个帧设置处理。
palette - 此选项允许您为保存的图像使用指定的调色板。调色板应作为包含 RGBRGB... 格式的调色板条目的字节或字节数组对象提供。它不应超过 768 个字节。或者,您可以将调色板作为 PIL.ImagePalette.ImagePalette 对象传递。
optimize - 如果设置为 true,则尝试通过消除未使用的颜色来压缩调色板。当调色板可以压缩到下一个较小的 2 的幂元素时,此优化很有用。
可以提供诸如透明度、持续时间、循环和注释之类的其他选项来控制动画 GIF 的特定方面。
示例
这是一个示例,演示了如何通过生成具有不同颜色的单个帧并保存它们来创建 GIF 动画。
import numpy as np from PIL import Image # Function to create a new image with a specified width, height, and color def create_image(width, height, color): return Image.new("RGBA", (width, height), color) # Set the width and height of the images width, height = 300, 300 # Define the colors for the images colors = [(64, 64, 3), (255, 0, 0), (255, 255, 0), (255, 255, 255), (164, 0, 3)] # Create a list of images using a list comprehension images = [create_image(width, height, color) for color in colors] # Save the images as a GIF with specified parameters images[0].save("Output.gif", save_all=True, append_images=images[1:], duration=1000/2, loop=0)
输出
The animated GIFs file is saved successfully...
您可以在工作目录中看到保存的动画 GIF:
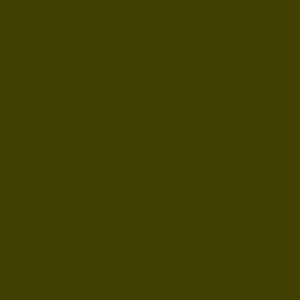
示例
以下示例获取现有图像文件的列表,通过按顺序保存这些图像来创建动画 GIF。
from PIL import Image # List of file paths for existing images image_paths = ['Images/book_1.jpg', 'Images/book_2.jpg', 'Images/book_3.jpg', 'Images/book_4.jpg'] # Create a list of image objects from the provided file paths image_list = [Image.open(path) for path in image_paths] # Save the first image as an animated GIF output_path = \Book_Animation.gif' image_list[0].save( output_path, save_all=True, append_images=image_list[1:], # Append the remaining images duration=1000, # Frame duration in milliseconds loop=0 ) print('The animated GIF file has been created and saved successfully...')
输出
The animated GIF file has been created and saved successfully...
下图显示了保存在工作目录中的动画 GIF:
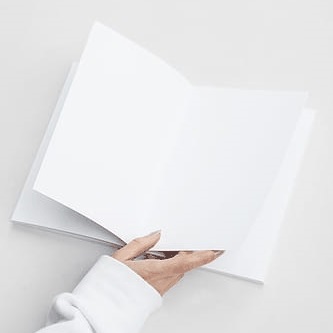
示例
此示例通过将现有 GIF 文件的最后一帧复制几次,然后将其另存为新的 GIF 文件来修改现有 GIF 文件。在此示例中,我们将使用 ImageSequence 模块来迭代输入 GIF 文件中的每个帧。
from PIL import Image, ImageSequence # Open the existing GIF file input_image = Image.open("Book_Animation.gif") # Create an empty list to store the frames of the GIF frames = [] # Iterate over the frames of the GIF and append them to the frames list for frame in ImageSequence.Iterator(input_image): frames.append(frame) # Duplicate the last frame three times to extend the animation for i in range(3): frames.append(frames[-1]) # Save the frames as a new GIF file ("newGif.gif"): output_path = "newGif.gif" frames[0].save( output_path, save_all=True, append_images=frames[1:], optimize=False, duration=40, # Set the frame duration to 40 milliseconds loop=0 )
输出
下图显示了保存在工作目录中的动画 GIF:
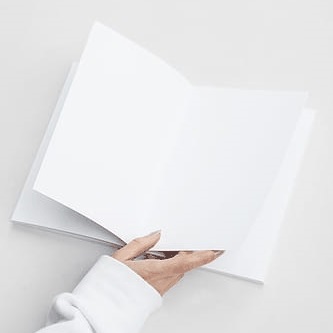