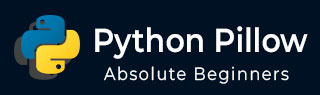
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上添加文字
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 创建彩色图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow - 使用 NumPy 进行机器学习
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 卷积滤镜
在图像处理的上下文中,卷积涉及将一个小矩阵(称为卷积核)的值应用于图像。此过程会产生各种滤镜效果,例如模糊、锐化、浮雕和边缘检测。内核中的每个值代表一个权重或系数。此内核应用于图像中相应像素的邻域,以产生输出图像中相应位置的输出像素值。
Python 的 Pillow 库在其 ImageFilter 模块中提供了一个名为“kernel”的特定类。此类用于创建大小超出传统 5x5 矩阵的卷积核。
创建卷积核
要创建卷积核,您可以使用 ImageFilter 模块中的 Kernel() 类。
需要注意的是,当前版本的 Pillow 支持 3×3 和 5×5 整数和浮点内核。这些内核仅适用于“L”和“RGB”模式的图像。
以下是此 ImageFilter.Kernel() 类的语法:
class PIL.ImageFilter.Kernel(size, kernel, scale=None, offset=0)
以下是类参数的详细信息:
size - 表示内核大小,指定为 (宽度,高度)。在当前版本中,有效大小为 (3,3) 或 (5,5)。
kernel - 包含内核权重的序列。内核在应用于图像之前会垂直翻转。
scale - 表示比例因子。如果提供,则每个像素的结果将除以该值。默认值为内核权重的总和。
offset - 表示偏移值。如果提供,则在除以比例因子后将此值添加到结果中。
示例
此示例演示如何使用 Image.filter() 方法将卷积核滤镜应用于图像。
from PIL import Image, ImageFilter # Create an image object original_image = Image.open('Images/Car_2.jpg') # Apply the Kernel filter filtered_image = original_image.filter(ImageFilter.Kernel((3, 3), (0, -1, 0, -1, 5, -1, 0, -1, 0))) # Display the original image original_image.show() # Display the filtered image filtered_image.show()
输入图像
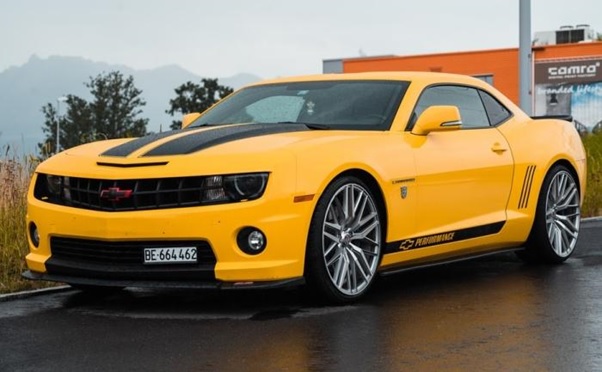
输出
卷积核滤镜的输出:
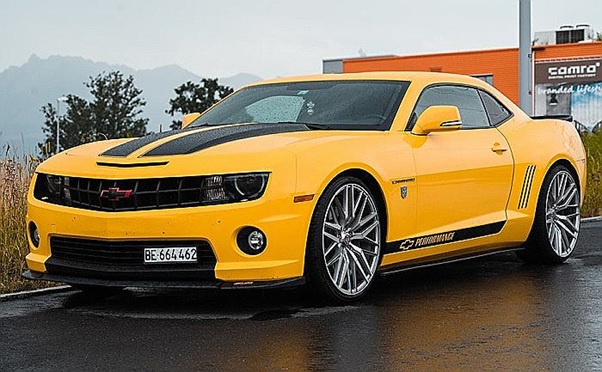
示例
这是一个将 5x5 浮雕卷积核滤镜应用于图像的示例。
from PIL import Image, ImageFilter # Create an image object original_image = Image.open('Images/Car_2.jpg') # Define a 5x5 convolution kernel kernel_5x5 = [-2, 0, -1, 0, 0, 0, -2, -1, 0, 0, -1, -1, 1, 1, 1, 0, 0, 1, 2, 0, 0, 0, 1, 0, 2] # Apply the 5x5 convolution kernel filter filtered_image = original_image.filter(ImageFilter.Kernel((5, 5), kernel_5x5, 1, 0)) # Display the original image original_image.show() # Display the filtered image filtered_image.show()
输入图像
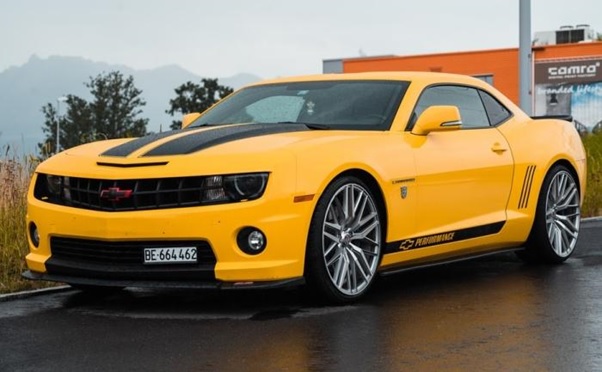
输出图像
大小为 5X5 的卷积核滤镜的输出:
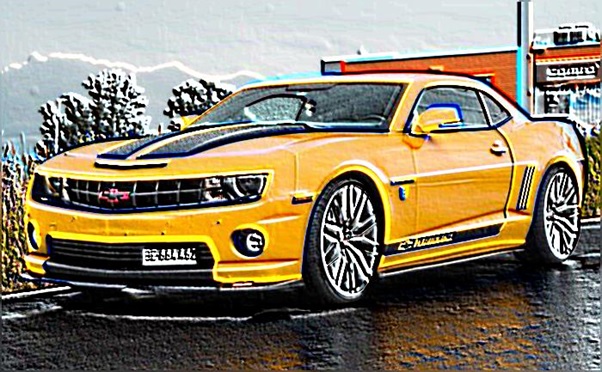
广告