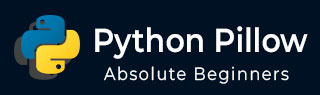
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 处理图像
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 连接两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强颜色
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow - 使用 Numpy 进行机器学习
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 提取图像元数据
图像元数据指的是与数字图像相关联的信息。这些元数据可以包含各种详细信息,例如相机型号、拍摄日期和时间、位置信息(GPS 坐标)和关键词。
在这种情况下,提取图像元数据是从图像中检索这些底层信息。一种广泛使用的图像元数据类型是 EXIF 数据,它是“可交换图像文件格式”的缩写。这种标准化格式由佳能、美能达/索尼和尼康等组织建立,封装了一套关于图像的综合规范,包括相机设置、曝光参数等。
需要注意的是,所有 EXIF 数据都是元数据,但并非所有元数据都是 EXIF 数据。EXIF 是一种特定类型的元数据,您正在查找的数据也可能属于其他元数据类型,例如 IPTC 或 XMP 数据。
提取基本图像元数据
Python Pillow Image 对象提供了一种简单的方法来访问基本图像元数据,包括文件名、尺寸、格式、模式等。
示例
此示例演示了如何使用 Pillow Image 对象的属性从图像中提取各种基本元数据。
from PIL import Image # Path to the image image_path = "Images/dance-cartoon.gif" # Read the image data using Pillow image = Image.open(image_path) # Access and print basic image metadata print("Filename:", image.filename) print("Image Size:", image.size) print("Image Height:", image.height) print("Image Width:", image.width) print("Image Format:", image.format) print("Image Mode:", image.mode) # Check if the image is animated (for GIF images) if hasattr(image, "is_animated"): print("Image is Animated:", image.is_animated) print("Frames in Image:", image.n_frames)
输出
Filename: Images/dance-cartoon.gif Image Size: (370, 300) Image Height: 300 Image Width: 370 Image Format: GIF Image Mode: P Image is Animated: True Frames in Image: 12
提取高级图像元数据
Python Pillow 库提供了访问和管理图像元数据的工具,特别是通过Image.getexif()函数和ExifTags模块。
Image.getexif()函数从图像中检索 EXIF 数据,其中包含大量关于图像的有价值的信息。使用此函数的语法如下:
Image.getexif()
该函数返回一个 Exif 对象,此对象提供对 EXIF 图像数据的读写访问。
PIL.ExifTags.TAGS字典用于解释 EXIF 数据。此字典将 16 位整数 EXIF 标记枚举映射到人类可读的描述性字符串名称,使元数据更容易理解。此字典的语法如下:
PIL.ExifTags.TAGS: dict
示例
以下示例演示了如何使用 Pillow 的getexif()方法访问和打印图像文件的 EXIF 元数据。它还展示了如何使用 TAGS 字典将标记 ID 映射到人类可读的标记名称。
from PIL import Image from PIL.ExifTags import TAGS # The path to the image image_path = "Images/flowers_canon.JPG" # Open the image using the PIL library image = Image.open(image_path) # Extract EXIF data exif_data = image.getexif() # Iterate over all EXIF data fields for tag_id, data in exif_data.items(): # Get the tag name, instead of the tag ID tag_name = TAGS.get(tag_id, tag_id) print(f"{tag_name:25}: {data}")
输出
GPSInfo : 10628 ResolutionUnit : 2 ExifOffset : 360 Make : Canon Model : Canon EOS 80D YResolution : 72.0 Orientation : 8 DateTime : 2020:10:25 15:39:08 YCbCrPositioning : 2 Copyright : CAMERAMAN_SAI XResolution : 72.0 Artist : CAMERAMAN_SAI
广告