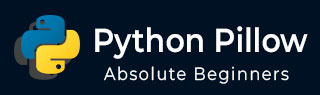
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境搭建
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 创建彩色图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 透视变换应用
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow 与 NumPy 的结合使用
- Python Pillow 与 Tkinter 的 BitmapImage 和 PhotoImage 对象结合使用
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 透视变换应用
在 Pillow 中应用透视变换涉及改变图像的视角或视点。这种变换以模拟视角变化的方式修改图像点的定位,例如旋转、倾斜或扭曲图像。
Pillow 中的透视变换
Pillow 的 transform() 方法允许你对图像应用不同类型的变换。对于透视变换,使用 3x3 变换矩阵来定义变换。此矩阵可以表示旋转、平移、缩放和剪切等操作。对于透视变换,矩阵元素控制每个像素坐标如何变化。
透视变换的矩阵元素
矩阵元素 (a, b, c, d, e, f, g, h) 定义变换:
a 和 d 代表 x 和 y 方向的缩放。
b 和 c 代表剪切或倾斜。
e 和 f 是沿 x 和 y 轴的平移。
g 和 h 是透视系数。
应用透视变换
我们可以直接使用 transform() 方法结合 Image.PERSPECTIVE,并传递四个源点和四个目标点来指定变换。
透视变换会显著扭曲图像。因此,请仔细选择变换矩阵或点,以获得所需的效果,避免过度扭曲。
在 Pillow 中应用透视变换涉及使用变换矩阵或定义源点和目标点来改变图像的透视,模拟角度、方向或视点的变化。理解变换矩阵和点映射对于获得所需的视觉效果至关重要,同时避免图像中出现过度扭曲或不需要的变换。
以下是 transform() 方法的语法和参数。
transformed_image = image.transform(size, method, data, resample=0, fill=0)
其中:
image − 我们要变换的 Pillow Image 对象。
size − 代表变换后图像的输出大小 (宽度, 高度) 的元组。
method − 定义要应用的变换类型,例如 Image.AFFINE、Image.PERSPECTIVE 或 Image.QUAD 等。
data − 根据所使用方法所需变换的数据。
resample (可选) − 要使用的重采样方法,例如 Image.BILINEAR、Image.NEAREST 和 Image.BICUBIC。默认值为 0,即最近邻。
fill (可选) − 变换后图像边界之外区域的填充颜色。默认值为 0,即黑色。
根据变换方法准备数据,对于 Image.AFFINE 变换,它需要一个 6 元素的元组来表示仿射变换矩阵。对于透视变换,使用 Image.PERSPECTIVE,它需要一个 8 元素的元组来表示透视变换矩阵。此外,Image.QUAD 涉及提供源和目标四边形点以进行精确的透视映射。
示例
在这个例子中,我们使用 transform() 方法对给定的输入图像执行透视变换。
from PIL import Image import numpy def find_data(source, target): matrix = [] for s, t in zip(source, target): matrix.append([t[0], t[1], 1, 0, 0, 0, -s[0]*t[0], -s[0]*t[1]]) matrix.append([0, 0, 0, t[0], t[1], 1, -s[1]*t[0], -s[1]*t[1]]) A = numpy.matrix(matrix, dtype=float) B = numpy.array(source).reshape(8) res = numpy.dot(numpy.linalg.inv(A.T * A) * A.T, B) return numpy.array(res).reshape(8) #Open an image image = Image.open("Images/logo_w.png") # image.show() #Define the transformation matrix for perspective transform coeffs = find_data( [(0, 0), (225, 0), (225, 225), (0, 225)], [(15, 115), (140, 20), (140, 340), (15, 250)]) #Applying the perspective transformation transformed_image = image.transform((300, 400), Image.PERSPECTIVE, coeffs, Image.BICUBIC) #Save or display the transformed image transformed_image.save("output Image/transform.png") transformed_image.show()
输出
以下是输入图像:
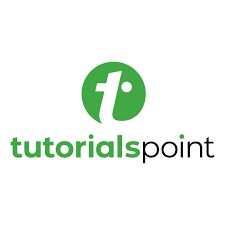
执行上述代码后,将显示以下变换后的图像:
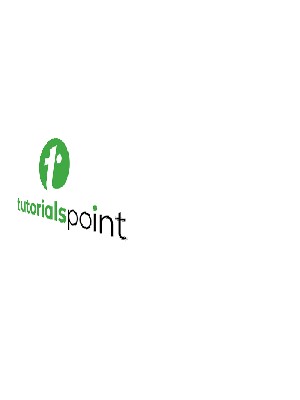
示例
这是一个使用矩阵元素 (a, b, c, d, e, f, g, h) 对另一个图像执行透视变换的另一个示例。
from PIL import Image #Open an image image = Image.open("Images/flowers.jpg") image.show() #Define the transformation matrix for perspective transform matrix = (10, 4, 11, -1, 5, 2, 1, -1) #Apply the perspective transformation transformed_image = image.transform(image.size, Image.PERSPECTIVE, matrix) #Save or display the transformed image transformed_image.save("output Image/transform_image.jpg") transformed_image.show()
输出
执行上述代码后,您将获得以下输出:
输入图像
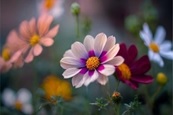
输出透视变换后的图像
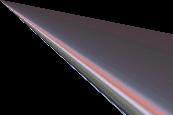
注意
transform() 方法支持多种变换类型,所需变换数据根据所选方法而异。
务必根据所选变换方法提供正确的变换数据(即矩阵或点),以达到预期效果。
Pillow 中的 transform() 方法用途广泛,允许我们对图像执行不同类型的几何变换,从而在图像处理和透视更改方面具有灵活性。