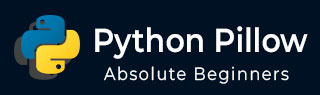
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境搭建
- 基本图像操作
- Python Pillow - 处理图像
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上写文字
- Python Pillow - ImageDraw 模块
- Python Pillow - 连接两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 处理 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 逆向锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强颜色
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow - 使用 NumPy 进行机器学习
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 处理 Alpha 通道
图像中的 Alpha 通道是一个表示每个像素透明度或不透明度的组件。它通常用于 RGBA 图像,其中每个像素由其红色、绿色、蓝色和 Alpha 值定义。此通道决定了图像中显示多少背景。简单来说,我们可以说 Alpha 值为 0 的像素完全透明,而 Alpha 值为255的像素完全不透明。
以下是具有不同透明度级别的图像的视觉表示 -

以下是与 Pillow 中 Alpha 通道相关的关键概念的细分 -
RGBA 模式
具有 Alpha 通道的图像通常以RGBA模式表示。
RGBA图像中的每个像素都有四个组件,即红色、绿色、蓝色和 Alpha。
透明度级别
Alpha 通道值通常在 0 到 255 之间。
0表示完全透明,即完全透明或不可见。
255表示完全不透明,即完全不透明或不可见。
访问 Alpha 通道
我们可以使用 Pillow Image 模块的split()函数访问 Alpha 通道。此函数用于分离图像的各个颜色通道。它将图像分解为其组成通道,使我们能够分别访问和操作每个通道。
使用split()函数分割通道提供了分别处理和操作各个颜色或 Alpha 通道的灵活性,从而可以进行精确的图像调整和处理。
当在图像对象上调用 split() 函数时,它会返回一个包含各个通道图像的元组。对于 RGBA 图像,元组包含红色、绿色、蓝色和 Alpha 通道。对于灰度或单通道图像,split() 函数仍然返回四个相同通道的相同副本。通过专门访问 Alpha 通道,您可以直接操作图像的透明度。
以下是 split() 函数的语法和参数 -
r,g,b,a = image.split()
其中,
image - 这是使用 Image.open() 函数打开的图像对象。
r, g, b, a - 这些变量分别接收表示红色、绿色、蓝色和 Alpha(即透明度)通道的单独波段。
示例
在此示例中,我们使用split()函数来分割和访问 Alpha 通道。
from PIL import Image # Open an image img_obj = Image.open('Images/colordots.png') #Split the image into RGBA channels red, green, blue, alpha = img_obj.split() # Alpha (transparency) channel alpha.show()
将要使用的输入 RGBA 图像
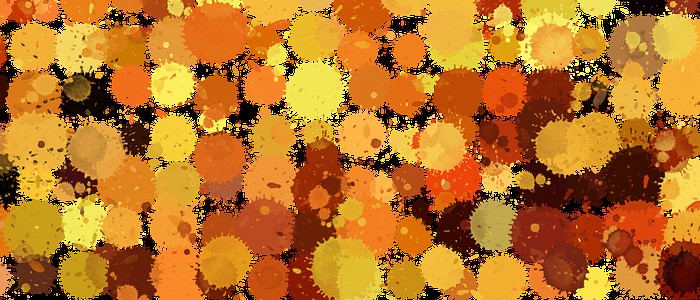
输出 Alpha 通道
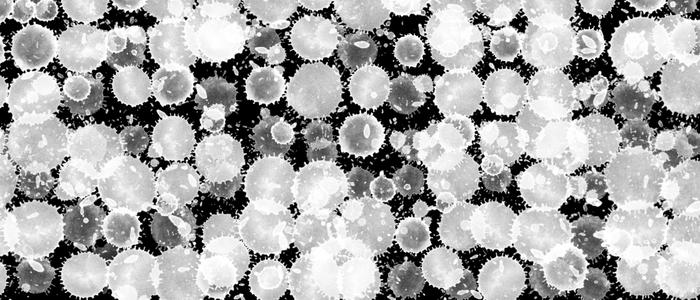
创建具有 Alpha 通道的图像
要创建具有 Alpha 通道的图像,我们可以使用new()函数。此函数提供诸如模式、宽度、高度和用于自定义图像的 RGBA 值之类的选项。最后一个参数的最后一个值定义图像的透明度,即 Alpha。
示例
在此示例中,我们将使用new()函数创建具有 Alpha 通道的图像。
from PIL import Image # Create an RGBA image with Semi-transparent green image = Image.new('RGBA', (700, 300), (10, 91, 51, 100)) # Display the resultant image with alpha channel image.show()
执行上述程序后,您将获得如下所示的 RGBA 输出 -
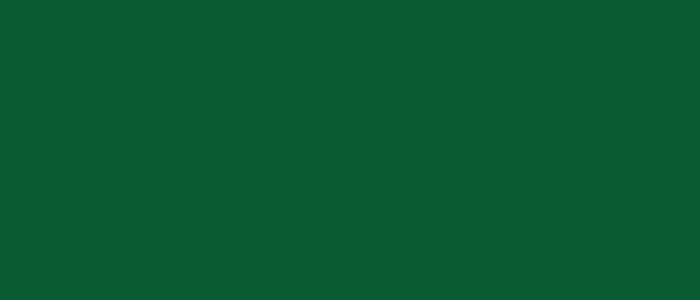
修改 Alpha 通道
可以使用putpixel()函数设置像素的各个 Alpha 值,以对图像的 Alpha 通道应用修改。
Python Pillow 的putpixel()函数用于更改图像中指定位置的单个像素的颜色。此函数允许我们通过提供其坐标和所需颜色来直接修改特定像素的颜色。
以下是 putpixel() 函数的语法和参数。
image.putpixel((x, y), color)
image - 这是使用 Image.open() 函数打开的图像对象。
(x, y) - 这些是我们想要设置颜色的像素的坐标。x 表示水平位置,y 表示垂直位置。
color - 我们想要分配给指定像素的颜色值。颜色值的格式取决于图像模式。
示例
在此示例中,我们使用 Pillow 库的putpixel()函数修改给定输入图像的像素的 Alpha 值。
from PIL import Image # Open an image image = Image.open("Images/colordots.png") width, height = image.size # Modify alpha values of pixels for y in range(height): for x in range(width): r, g, b, a = image.getpixel((x, y)) image.putpixel((x, y), (r, g, b, int(a * 0.5))) # Reduce alpha by 50% # Display the modified image image.show()
输入图像
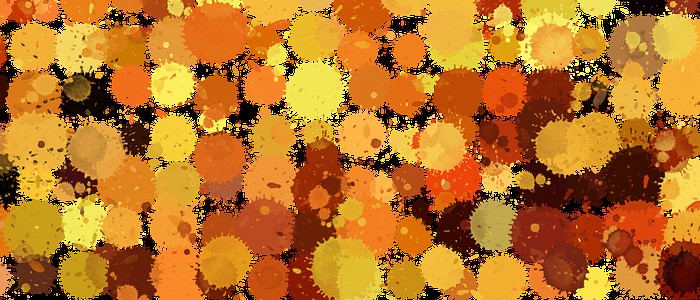
输出修改后的图像
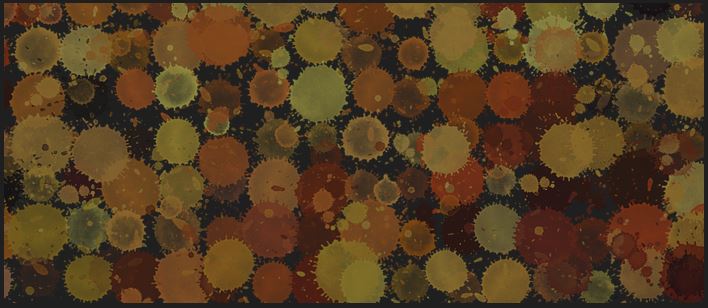
注意
putpixel() 函数直接修改内存中的图像。它对于对单个像素进行少量修改很有用。
对于大规模像素操作或更复杂的处理,我们必须考虑使用其他 Pillow 函数,这些函数可以对更大的区域或整个图像进行操作,以获得更好的性能。
使用 Alpha 通道合成图像
Image.alpha_composite()函数用于合成两张具有 Alpha 通道的图像。此函数根据其 Alpha 通道混合图像。
示例
在此示例中,我们使用Image.alpha_composite()函数根据其 Alpha 通道混合两张图像。
from PIL import Image # Open two images img1 = Image.open('Images/tp_logo.png') img2 = Image.open('Images/colordots_2.png') # Composite the images composite = Image.alpha_composite(img1, img2) # Display the result composite.show()
输入图像 -
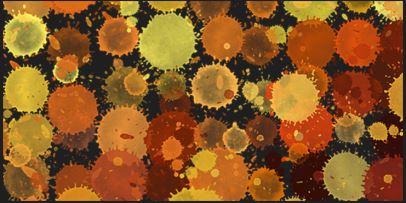

输出 Alpha 合成图像 -
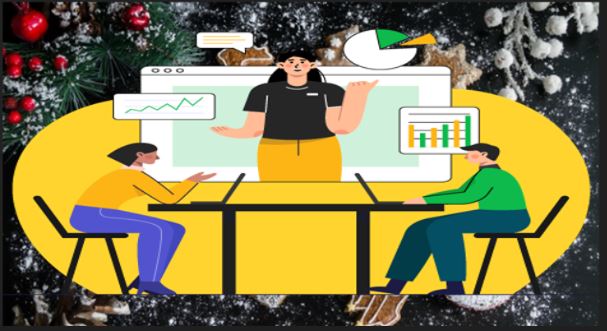
保存具有 Alpha 通道的图像
为了保留透明度,请以支持 Alpha 通道的格式(如 PNG)保存图像。JPEG 等格式不支持 Alpha 通道,并将丢弃透明度信息。我们可以使用 Pillow 中提供的save()函数。
示例
在此示例中,我们使用Image.save()函数保存具有 Alpha 通道的图像。
from PIL import Image # Create a new image with alpha channel new_img = Image.new('RGBA', (700, 300), (255, 0, 0, 128)) # Save the image with alpha channel new_img.save("output Image/new_img.png", "PNG") print("The image with alpha channel is saved.")
执行上述代码后,您将在当前工作目录中找到生成的 PNG 文件“new_img.png” -
The image with alpha channel is saved.