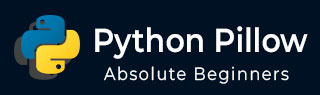
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 处理图像
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 裁切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 连接两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强颜色
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow - 使用 NumPy 进行机器学习
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 模糊图像
模糊图像是图像处理中的一个基本概念,用于降低图像的细节或锐度。模糊的主要目的是使图像看起来更平滑或不那么锐利。模糊可以通过各种数学运算、卷积核或滤镜来实现。
Python 的 Pillow 库在 ImageFilter 模块中提供了几个标准图像滤镜,通过调用 image.filter() 方法对图像执行不同的模糊操作。在本教程中,我们将了解 ImageFilter 模块提供的不同图像模糊滤镜。
将模糊滤镜应用于图像
模糊图像可以通过使用 ImageFilter.BLUR 滤镜来完成,它是 Pillow 库当前版本中提供的内置滤镜选项之一,用于在图像中创建模糊效果。
以下是应用图像模糊的步骤:
使用 Image.open() 函数加载输入图像。
将 filter() 函数应用于加载的 Image 对象,并向函数提供 ImageFilter.BLUR 作为参数。该函数将返回模糊后的图像,作为 PIL.Image.Image 对象。
以下是本章所有示例中使用的输入图像。
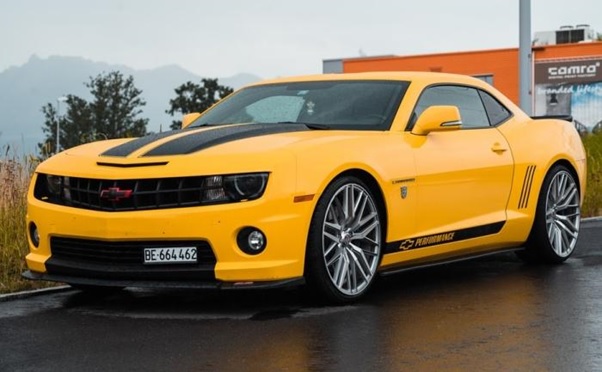
示例
以下是使用 Image.filter() 方法和 ImageFilter.BLUR 内核滤镜的示例。
from PIL import Image, ImageFilter # Open an existing image original_image = Image.open('Images/car_2.jpg') # Apply a blur filter to the image blurred_image = original_image.filter(ImageFilter.BLUR) # Display the original image original_image.show() # Display the blurred mage blurred_image.show()
输出图像
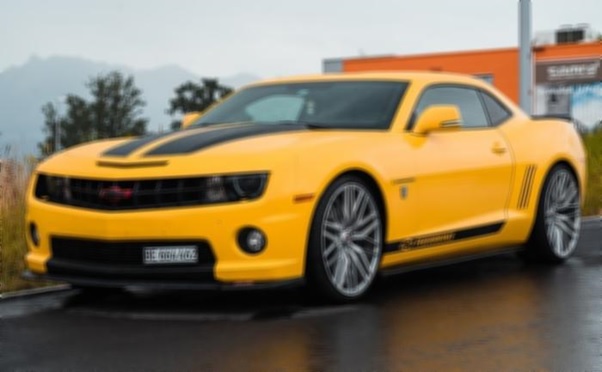
应用 BoxBlur 滤镜
BoxBlur 滤镜用于通过将每个像素的值设置为扩展到每个方向指定像素数的正方形框内像素的平均值来模糊图像。为此,您可以使用 Pillow 的 ImageFilter 模块中的 BoxBlur() 类。
以下是 ImageFilter.BoxBlur() 类的语法:
class PIL.ImageFilter.BoxBlur(radius)
PIL.ImageFilter.BoxBlur() 类接受一个参数:
radius - 此参数指定用于在每个方向上模糊的正方形框的大小。可以将其提供为两个数字的序列(用于 x 和 y 方向)或一个应用于两个方向的数字。
如果 radius 设置为 1,则采用 3x3 正方形框内像素值的平均值(每个方向 1 个像素,总共 9 个像素)。radius 值为 0 不会模糊图像,并返回相同的图像。
示例
以下是一个演示如何使用 BoxBlur() 类进行图像模糊的示例。
from PIL import Image, ImageFilter # Open an existing image original_image = Image.open('Images/car_2.jpg') # Apply a Box Blur filter to the image box_blurred_image = original_image.filter(ImageFilter.BoxBlur(radius=3)) # Display the Box Blurred image box_blurred_image.show()
输出图像
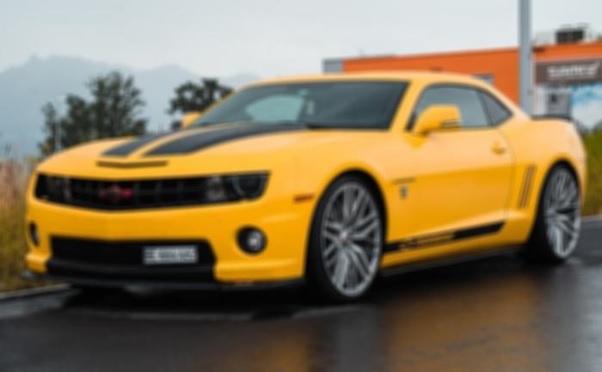
将高斯模糊应用于图像
高斯模糊滤镜用于通过应用高斯模糊来模糊图像,高斯模糊是一种近似高斯分布的特定模糊类型。这可以通过使用 Pillow 的 ImageFilter.GaussianBlur() 类来完成。
以下是 ImageFilter.GaussianBlur() 类的语法:
class PIL.ImageFilter.GaussianBlur(radius=2)
ImageFilter.GaussianBlur() 类接受一个参数:
radius - 此参数指定高斯核的标准差。标准差控制模糊的程度。您可以将其提供为两个数字的序列(用于 x 和 y 方向)或一个应用于两个方向的数字。
示例
以下是一个演示如何使用 GaussianBlur() 类进行图像模糊的示例。
from PIL import Image, ImageFilter # Open an existing image original_image = Image.open('Images/car_2.jpg') # Apply a Gaussian Blur filter to the image gaussian_blurred_image = original_image.filter(ImageFilter.GaussianBlur(radius=2)) # Display the Gaussian Blurred image gaussian_blurred_image.show()
输出图像
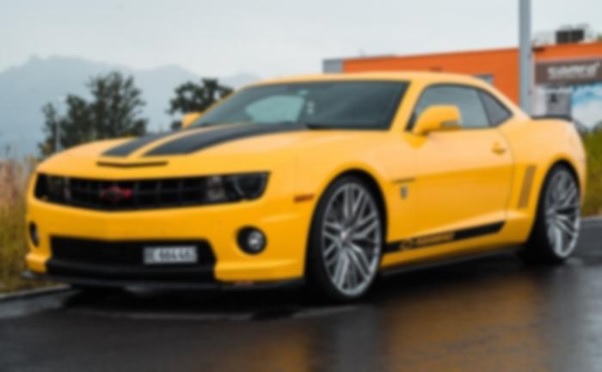