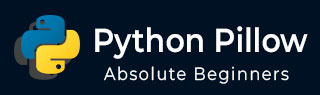
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境搭建
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 图像缩放
- Python Pillow - 图像翻转和旋转
- Python Pillow - 图像裁剪
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 图像合并
- Python Pillow - 图像剪切和粘贴
- Python Pillow - 图像滚动
- Python Pillow - 在图像上添加文字
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 逆向锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow 与 NumPy 结合使用
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象结合使用
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - 图像合成
什么是图像合成?
在 Pillow 中,图像合成涉及将两张或多张图像组合起来以创建新的图像。此过程通常包括根据某些标准(例如透明度或特定混合模式)将一张图像的像素值与另一张图像的像素值混合。
Pillow 提供了 `Image.alpha_composite()` 方法用于图像合成,尤其是在处理 Alpha(透明度)通道时。它通常用于将一张图像叠加到另一张图像上,添加水印或创建特殊效果。
以下是与 Pillow 中的图像合成相关的关键概念:
图像合成
通过将一张图像叠加到另一张图像上来组合图像以创建新的图像。
图像合成通常用于添加水印或创建特殊效果。
Alpha 通道
Alpha 通道表示图像中每个像素的透明度。
具有 Alpha 通道的图像可以更无缝地合成,从而实现平滑混合。
`Image.alpha_composite()` 方法
Pillow 中的 `Image.alpha_composite()` 方法用于使用它们的 Alpha 通道合成两张图像。它接受两个 `Image` 对象作为输入,并返回一个包含合成结果的新 `Image`。
以下是 `Image.alpha_composite()` 方法的语法和参数:
PIL.Image.alpha_composite(image1, image2)
其中:
`image1` - 将第二张图像合成到的背景图像。
`image2` - 将合成到背景图像上的前景图像。
示例
此示例演示如何使用 `Image.alpha_composite()` 方法合成两张图像。
from PIL import Image #Open or create the background image background = Image.open("Images/decore.png") #Open or create the foreground image with transparency foreground = Image.open("Images/library_banner.png") #Ensure that both images have the same size if background.size != foreground.size: foreground = foreground.resize(background.size) #Perform alpha compositing result = Image.alpha_composite(background, foreground) # Display the resulting image result.show()
待使用的图像
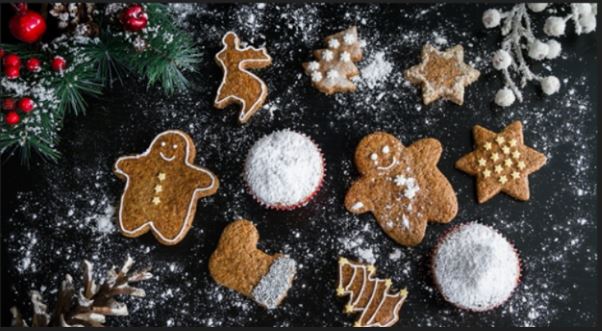
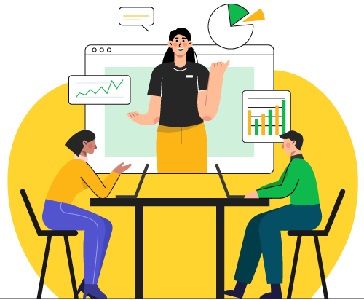
输出
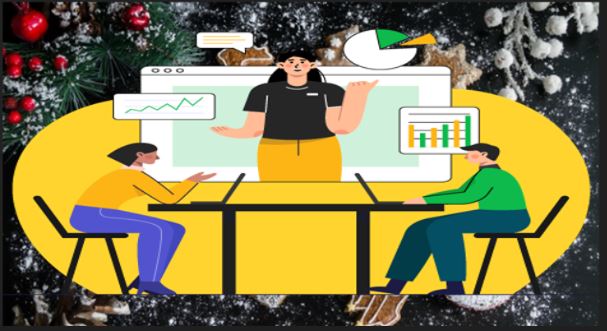
使用图像合成添加水印
为图像添加水印是图像合成任务中的一个应用。您可以将水印叠加到图像上的特定位置和透明度。这是通过为水印创建一个新图层并使用 `Image.alpha_composite()` 方法将其与底层图像混合来实现的。
示例
此示例演示如何使用 `Image.alpha_composite()` 方法将水印添加到图像中。水印图像以可调节的透明度放置在底层图像的顶部。
from PIL import Image # Load the images and convert them to RGBA image = Image.open('Images/yellow_car.jpg').convert('RGBA') watermark = Image.open('Images/reading_img2.png').convert('RGBA') # Create an empty RGBA layer with the same size as the image layer = Image.new('RGBA', image.size, (0, 0, 0, 0)) layer.paste(watermark, (20, 20)) # Create a copy of the layer and adjust the alpha transparency layer2 = layer.copy() layer2.putalpha(128) # Merge the layer with its transparent copy using the alpha mask layer.paste(layer2, (0, 0), layer2) # Composite the original image with the watermark layer result = Image.alpha_composite(image, layer) # Display the resulting image result.show()
待使用的图像
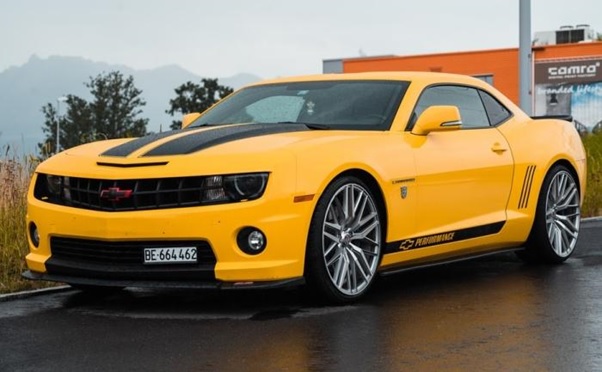
水印图像

输出
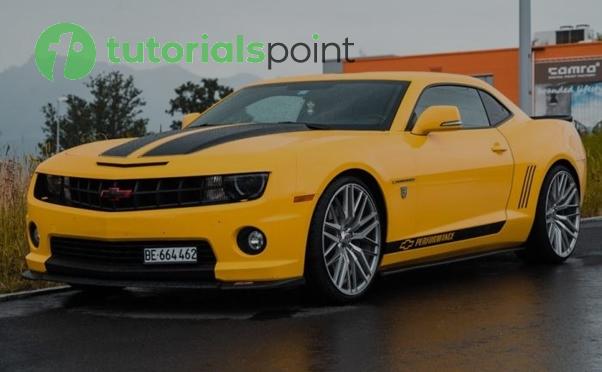
广告