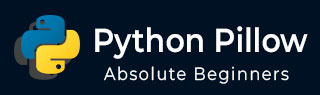
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 连接两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow - 使用 NumPy 进行机器学习
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - ImageChops.subtract() 函数
PIL.ImageChops.subtract() 函数用于从一个图像 (image1) 中减去另一个图像 (image2)。减法完成后,结果将除以指定的比例,然后添加一个偏移量。此操作的数学表示由以下公式给出:
$$\mathrm{out\:=\:((image1\:-\:image2)/scale\:+\:offset)}$$
与 ImageChops.add 类似,由于数据类型为 uint8,因此此操作不可逆。使用 ImageChops.subtract 时,如果结果值为负,则将其剪裁为零。这种剪裁到 [0, 255] 范围会导致数据丢失,并且无法从结果中准确地重建原始图像。
语法
以下是函数的语法:
PIL.ImageChops.subtract(image1, image2, scale=1.0, offset=0)
参数
以下是此函数参数的详细信息:
image1 - 这是第一个输入图像。
image2 - 这是第二个输入图像。
scale(可选,默认值:1.0) - scale 是将 image1 和 image2 相减的结果除以的因子。如果省略此参数,则默认为 1.0。
offset(可选,默认值:0.0) - offset 是在除以 scale 后添加到结果的值。如果不提供偏移值,则默认为 0.0。
返回值
此函数返回 Image 对象。
示例
示例 1
在此示例中,使用 NumPy 数组创建了两个随机的 RGB 图像(image1 和 image2)。然后应用 ImageChops.subtract 函数以查看在执行减法后两个图像的像素值如何在输出图像中表示。
from PIL import Image, ImageChops import numpy as np # Create two random RGB images image1 = Image.fromarray(np.array([(235, 64, 3), (255, 0, 0), (255, 255, 0), (255, 255, 255), (164, 0, 3)]), mode="RGB") print("Pixel values of image1 at (0, 0):", image1.getpixel((0, 0))) image2 = Image.fromarray(np.array([(255, 14, 3), (25, 222, 0), (255, 155, 0), (255, 55, 100), (180, 0, 78)]), mode="RGB") print("Pixel values of image2 at (0, 0):", image2.getpixel((0, 0))) # Subtract the two images result = ImageChops.subtract(image1, image2) print("Pixel values of the result at (0, 0) after subtraction:", result.getpixel((0, 0)))
输出
Pixel values of image1 at (0, 0): (235, 0, 0) Pixel values of image2 at (0, 0): (255, 0, 0) Pixel values of the result at (0, 0) after subtraction: (0, 0, 0)
我们可以观察到输出,(0, 0) 处的像素为 (0, 0, 0),因为减法 (235 - 255) 被剪裁为 0。
示例 2
以下示例使用默认参数 (scale=1.0, offset=0.0) 从第一个图像中减去第二个图像。
from PIL import Image, ImageChops # Open two image files image1 = Image.open('Images/TP logo.jpg') image2 = Image.open('Images/Rose_.jpg') # Subtract the second image from the first with default parameters (scale=1.0, offset=0.0) result = ImageChops.subtract(image1, image2) # Display the input and resulting images image1.show() image2.show() result.show()
输出
输入图像 1
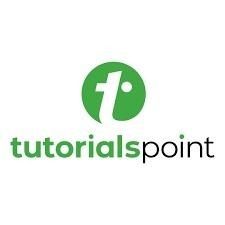
输入图像 2
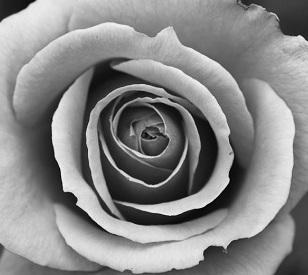
输出图像
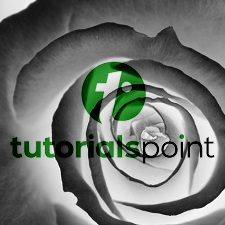
示例 3
这是一个示例,它使用特定的 scale 和 offset 值从第一个图像中减去第二个图像。
from PIL import Image, ImageChops # Open two image files image1 = Image.open('Images/Rose_.jpg') image2 = Image.open('Images/TP logo.jpg') # Subtract the second image from the first with specific scale and offset values result = ImageChops.subtract(image1, image2, scale=5.0, offset=100) # Display the input and resulting images image1.show() image2.show() result.show()
输出
输入图像 1
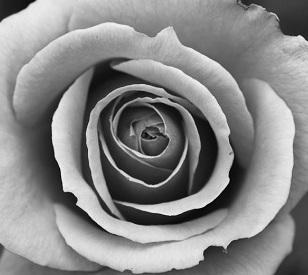
输入图像 2
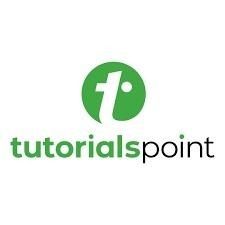
输出图像
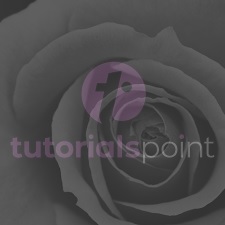