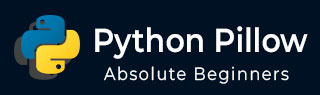
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 图像滚动
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 创建彩色图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 锐化遮罩滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强色彩
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow 与 NumPy 结合使用
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象结合使用
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - ImageDraw.point() 函数
ImageDraw.point() 方法用于绘制点,即图像上指定坐标处的单个像素。Python Pillow 库在其 ImageDraw 模块中提供此 point() 方法,使用 Draw() 类创建的 Draw 对象在图像上绘制点。
语法
以下是该函数的语法:
ImageDraw.point(xy, fill=None)
参数
以下是此函数参数的详细信息:
xy - 此参数表示要绘制的点的坐标。它可以指定为 2 元组序列,例如 [(x, y), (x, y), ...],或者数值序列,例如 [x, y, x, y, ...]。每个元组或数值对对应于点的 (x, y) 坐标。
fill - 用于点的颜色。此参数指定绘制点的颜色。
示例
示例 1
在此示例中,点绘制在指定的坐标处,填充颜色设置为黄色。
from PIL import Image, ImageDraw # Create a new image with a white background image = Image.new("RGB", (700, 300), "green") draw = ImageDraw.Draw(image) # Specify coordinates for points point_coordinates = [(100, 100), (200, 100), (100, 200), (200, 200), (150, 100), (200, 100), (150, 200), (200, 200), (250, 100), (300, 100), (250, 200), (300, 200), (350, 100), (400, 100), (350, 200), (400, 200), (450, 100), (500, 100), (450, 200), (500, 200), (550, 100), (600, 100), (550, 200), (600, 200)] # Set fill color for the points point_fill_color = "yellow" # Draw points on the image draw.point(point_coordinates, fill=point_fill_color) # Display the image image.show() print('The points are drawn successfully...')
输出
The points are drawn successfully...
输出图像
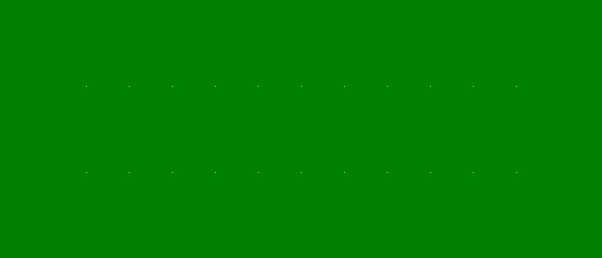
示例 2
以下示例演示了使用循环在黑色背景上以网格图案绘制点。
from PIL import Image, ImageDraw # Create a new image with a black background image_width, image_height = 700, 300 image = Image.new("RGB", (image_width, image_height), "black") draw = ImageDraw.Draw(image) # Set fill color for the points point_fill_color = "yellow" # Define step size and range for coordinates step = 20 x_range = range(step, image_width, step) y_range = range(step, image_height - step, step) # Draw points on the image for n in x_range: for x in y_range: draw.point((n, x), fill=point_fill_color) # Display the image image.show() print('The points are drawn successfully...')
输出
The points are drawn successfully...
输出图像
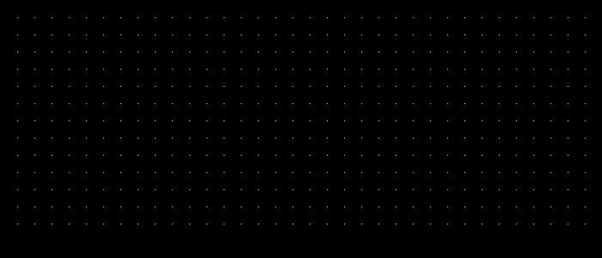
示例 3
此示例演示如何使用 point() 函数在随机位置绘制更多点。
from PIL import Image, ImageDraw import random # Create a new image with a white background image_width, image_height = 700, 300 image = Image.new("RGB", (image_width, image_height), "white") draw = ImageDraw.Draw(image) # Set fill color for the points point_fill_color = "blue" # Draw 1000 randomly positioned points on the image num_points = 200 for temp in range(num_points): x = random.randint(0, image_width - 1) y = random.randint(0, image_height - 1) draw.point((x, y), fill=point_fill_color) # Display the image image.show() print('The points are drawn successfully...')
输出
The points are drawn successfully...
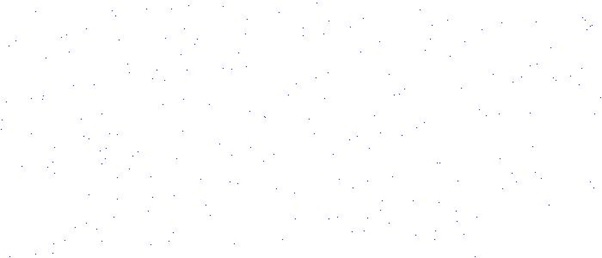
python_pillow_function_reference.htm
广告