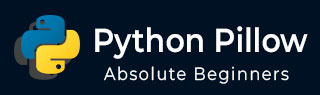
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像上的颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 反锐化蒙版滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强颜色
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow 与 NumPy 结合使用
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象结合使用
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - ImageMath.eval() 函数
Pillow 库在其 ImageMath 模块中提供了一个名为 eval() 的函数,用于评估图像表达式。它允许您对图像执行算术、位运算和逻辑运算等操作。该模块支持标准 Python 表达式语法,并提供 Pillow 库提供的附加函数。
PIL.ImageMath.eval() 函数在给定的环境中评估表达式。此函数支持标准 Python 表达式语法以及用于图像处理的其他函数。
需要注意的是,ImageMath 模块目前仅支持单层图像。要处理多波段图像,应使用 split() 方法或 merge() 函数。
语法
以下是函数的语法:
PIL.ImageMath.eval(expression, environment)
参数
以下是此函数参数的详细信息:
expression - 表示 Python 表达式的字符串。
environment - 字典或关键字参数,将图像名称映射到 Image 实例。
返回值
该函数返回一个图像、一个整数值、一个浮点值或一个像素元组,具体取决于表达式。
示例
示例 1
此示例演示如何使用 ImageMath 模块的 eval() 函数使用标准算术运算符评估表达式。
from PIL import Image, ImageMath # Open an image and convert it to grayscale image1 = Image.open("Images/black rose.jpg").convert('L') # Evaluate the expression 'a + 100' to invert pixel values resultant_image = ImageMath.eval('a + 100', a=image1) # Display the original and resultant images image1.show() resultant_image.show() print('Evaluated the expression with standard arithmetical operator successfully...')
输出
输入图像
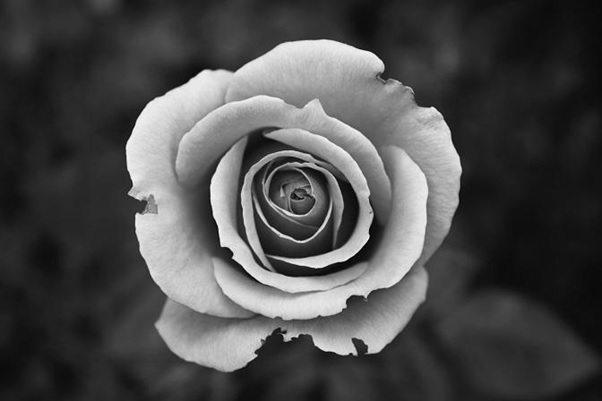
结果图像
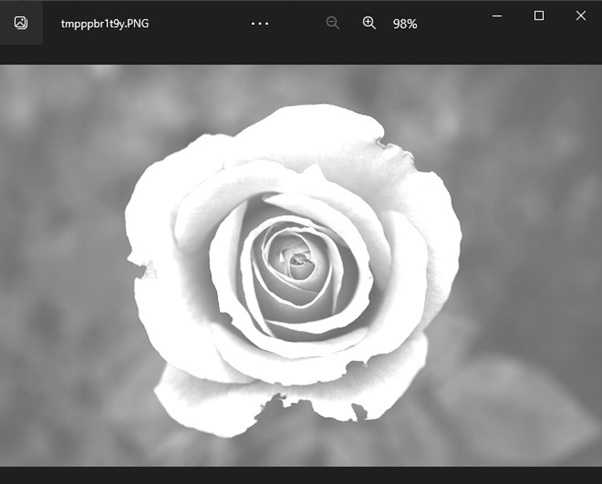
Evaluated the expression with standard arithmetical operator successfully...
示例 2
这是一个示例,演示如何使用 eval() 函数使用位运算符评估表达式。
需要注意的是,位运算符不适用于浮点图像。
from PIL import Image, ImageMath # Open and convert the first grayscale image image1 = Image.open("Images/ColorDots.png").convert('L') # Open and convert the second grayscale image image2 = Image.open("Images/TP-W.png").convert('L') # Apply bitwise AND operation on the two images resultant_image = ImageMath.eval('a & b', a=image1, b=image2) # Display the original images and the result image1.show() image2.show() resultant_image.show() print('Evaluated the expression of the Bitwise operator successfully...')
输出
输入图像 1
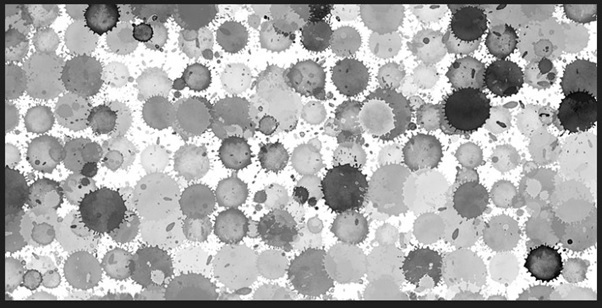
输入图像 2
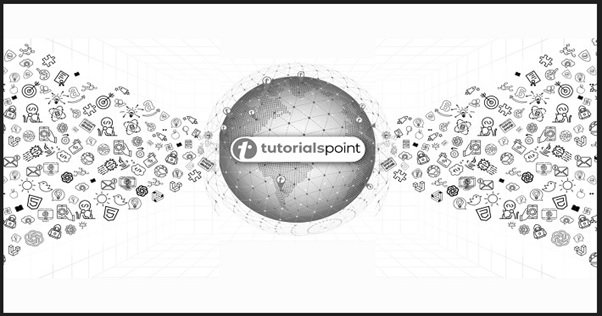
输出图像
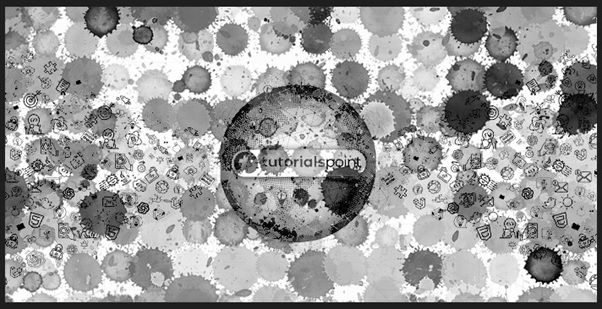
示例 3
以下示例对整个图像应用逻辑“或”运算符。
from PIL import Image, ImageMath # Open and convert the first grayscale image image1 = Image.open("Images/ColorDots.png").convert('L') # Open and convert the second grayscale image image2 = Image.open("Images/TP-W.png").convert('L') # Use the logical "or" operation on the entire images resultant_image = ImageMath.eval('(a or b)', a=image1, b=image2) # Display the original images and the result image1.show() image2.show() resultant_image.show() print('Evaluated the expression using the logical OR successfully...')
输出
输入图像 1
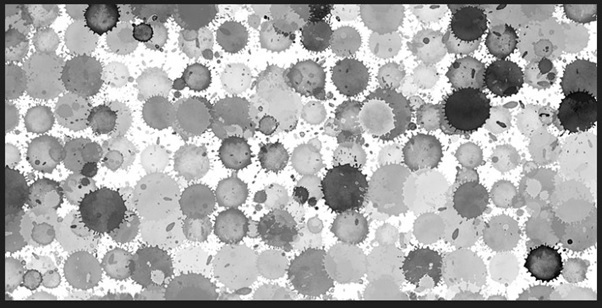
输入图像 2
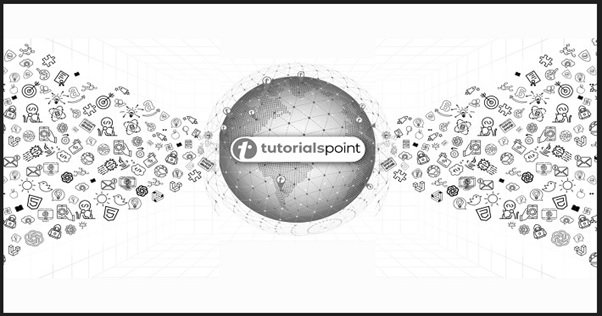
输出图像
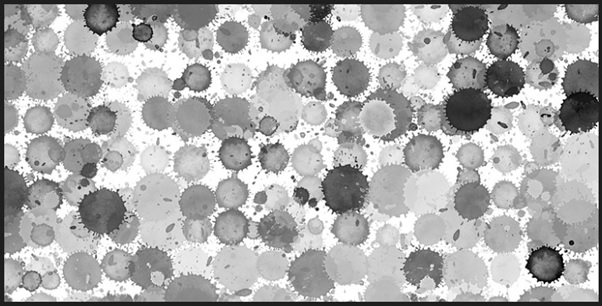
示例 3
以下示例使用 ImageMath.eval() 函数评估包含内置函数的表达式。
from PIL import Image, ImageMath # Open and convert the first grayscale image image1 = Image.open("Images/ColorDots.png").convert('L') # Open and convert the second grayscale image image2 = Image.open("Images/TP-W.png").convert('L') # Evaluate the expression "min(a, b)" using ImageMath resultant_image = ImageMath.eval('min(a, b)', a=image1, b=image2) # Display the original images and the result image1.show() image2.show() resultant_image.show() print('Evaluated the expression using the Built-in Functions successfully...')
输出
输入图像 1
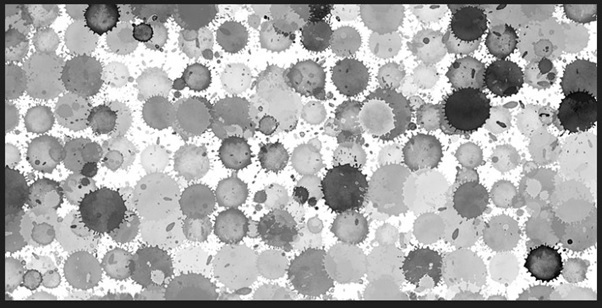
输入图像 2
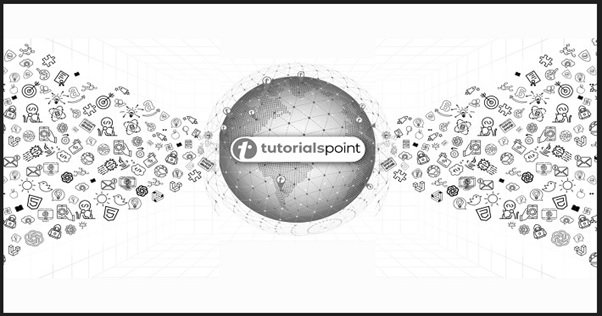
输出图像
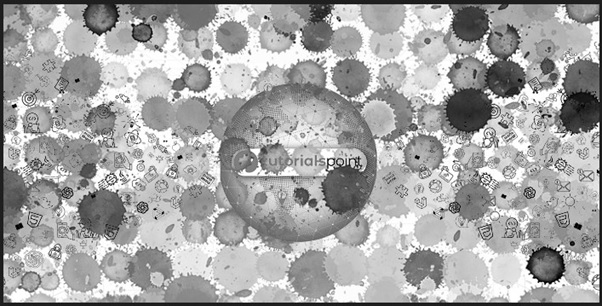
python_pillow_function_reference.htm
广告