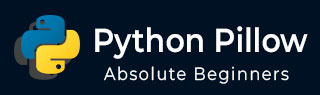
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 剪切和粘贴图像
- Python Pillow - 滚动图像
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像颜色
- Python Pillow - 创建彩色图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 不锐化掩膜滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强颜色
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow 与 NumPy 的结合使用
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象结合使用
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - ImageOps.Colorize() 函数
PIL.ImageOps.colorize() 函数用于将灰度图像着色。它计算一个颜色楔形,将源图像中的所有黑色像素映射到第一种颜色,将所有白色像素映射到第二种颜色。如果指定了中间色 (mid),则使用三色映射。黑白参数应作为 RGB 元组或颜色名称提供。可以选择通过包含 mid 参数来进行三色映射。对于每种颜色,可以使用诸如 blackpoint 的参数定义映射位置,该参数表示一个整数,指示应将相应颜色映射到的位置。必须为这些参数保持逻辑顺序,确保 blackpoint 小于或等于 midpoint,而 midpoint 小于或等于 whitepoint(如果指定了 mid)。
语法
以下是该函数的语法:
PIL.ImageOps.colorize(image, black, white, mid=None, blackpoint=0, whitepoint=255, midpoint=127)
参数
以下是该函数参数的详细信息:
image - 要着色的灰度图像。
black - 用于黑色输入像素的颜色,指定为 RGB 元组或颜色名称。
white - 用于白色输入像素的颜色,指定为 RGB 元组或颜色名称。
mid - 用于中间色输入像素的颜色,指定为 RGB 元组或颜色名称(三色映射可选)。
blackpoint - 整数值 [0, 255],表示黑色颜色的映射位置。
whitepoint - 整数值 [0, 255],表示白色颜色的映射位置。
midpoint - 整数值 [0, 255],表示中间色的映射位置(三色映射可选)。
返回值
该函数返回表示着色结果的图像。
示例
示例 1
这是一个使用基本参数的 ImageOps.colorize() 函数使用方法示例。
from PIL import Image, ImageOps # Open a grayscale image gray_image = Image.open("Images/flowers_1.jpg").convert("L") # Define colors for mapping red = (255, 0, 0) cyan = (0, 255, 255) # Apply colorize operation colorized_image = ImageOps.colorize(gray_image, black=red, white=cyan) # Display the original and colorized images gray_image.show() colorized_image.show()
输出
输入图像
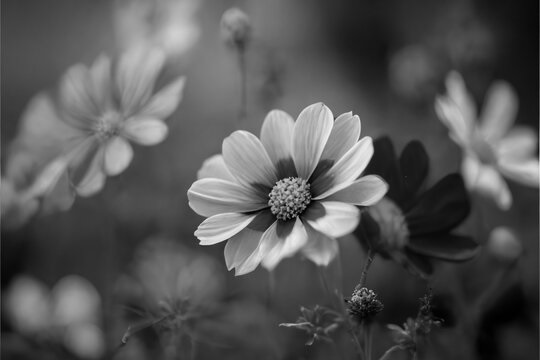
输出图像
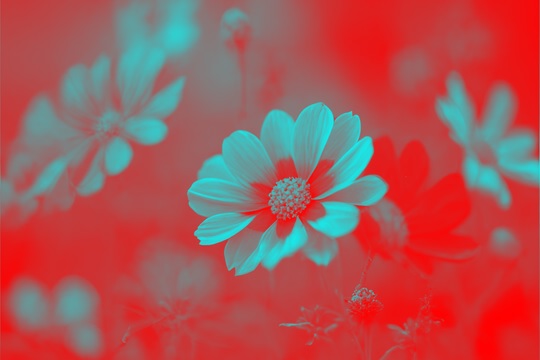
示例 2
此示例演示了使用可选的三色映射对灰度图像进行着色。
from PIL import Image, ImageOps # Open a grayscale image gray_image = Image.open("Images/Car_2.jpg").convert("L") # Define colors for mapping black_color = 'yellow' white_color = 'red' mid_color = 'cyan' # color name (optional for three-color mapping) # Define mapping positions blackpoint = 50 whitepoint = 200 midpoint = 127 # Apply colorize operation colorized_image = ImageOps.colorize(gray_image, black_color, white_color, mid_color, blackpoint, whitepoint, midpoint) # Display the original and colorized images gray_image.show() colorized_image.show()
输出
输入图像
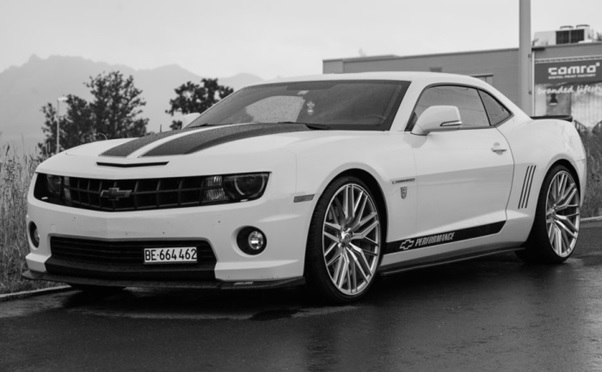
输出图像
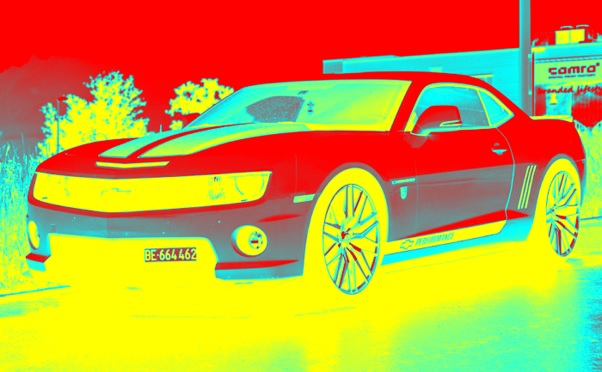
示例 3
此示例演示如何使用不同的颜色和映射位置应用 colorize 函数以实现独特的着色结果。
from PIL import Image, ImageOps # Open a grayscale image gray_image = Image.open("Images/Car_2.jpg").convert("L") # Define colors for mapping (RGB tuple) warm_black = (10, 10, 10) cool_white = (200, 220, 255) neutral_gray = (128, 128, 128) # Define mapping positions blackpoint = 30 whitepoint = 200 midpoint = 120 # Apply colorize operation with custom colors and mapping positions colorized_image = ImageOps.colorize(gray_image, warm_black, cool_white, neutral_gray, blackpoint, whitepoint, midpoint) # Display the original and colorized images gray_image.show() colorized_image.show()
输出
输入图像
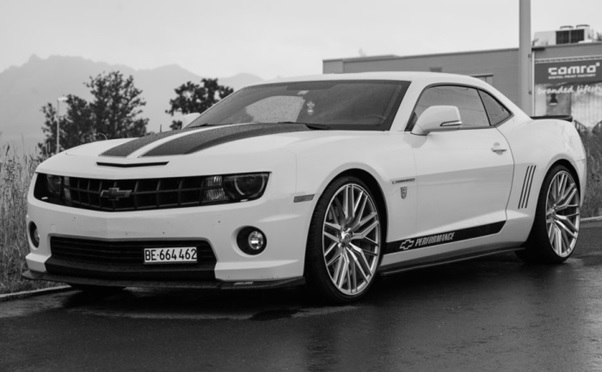
输出图像
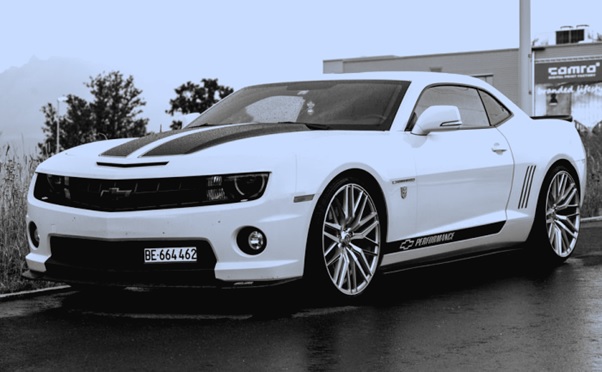
python_pillow_function_reference.htm
广告