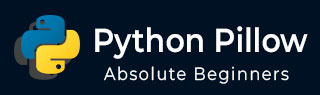
- Python Pillow 教程
- Python Pillow - 首页
- Python Pillow - 概述
- Python Pillow - 环境设置
- 基本图像操作
- Python Pillow - 图像处理
- Python Pillow - 调整图像大小
- Python Pillow - 翻转和旋转图像
- Python Pillow - 裁剪图像
- Python Pillow - 为图像添加边框
- Python Pillow - 识别图像文件
- Python Pillow - 合并图像
- Python Pillow - 图像剪切和粘贴
- Python Pillow - 图像滚动
- Python Pillow - 在图像上写入文本
- Python Pillow - ImageDraw 模块
- Python Pillow - 合并两张图像
- Python Pillow - 创建缩略图
- Python Pillow - 创建水印
- Python Pillow - 图像序列
- Python Pillow 颜色转换
- Python Pillow - 图像颜色
- Python Pillow - 使用颜色创建图像
- Python Pillow - 将颜色字符串转换为 RGB 颜色值
- Python Pillow - 将颜色字符串转换为灰度值
- Python Pillow - 通过更改像素值来更改颜色
- 图像处理
- Python Pillow - 降噪
- Python Pillow - 更改图像模式
- Python Pillow - 图像合成
- Python Pillow - 使用 Alpha 通道
- Python Pillow - 应用透视变换
- 图像滤镜
- Python Pillow - 为图像添加滤镜
- Python Pillow - 卷积滤镜
- Python Pillow - 模糊图像
- Python Pillow - 边缘检测
- Python Pillow - 浮雕图像
- Python Pillow - 增强边缘
- Python Pillow - 非锐化掩膜滤镜
- 图像增强和校正
- Python Pillow - 增强对比度
- Python Pillow - 增强锐度
- Python Pillow - 增强颜色
- Python Pillow - 校正色彩平衡
- Python Pillow - 去噪
- 图像分析
- Python Pillow - 提取图像元数据
- Python Pillow - 识别颜色
- 高级主题
- Python Pillow - 创建动画 GIF
- Python Pillow - 批量处理图像
- Python Pillow - 转换图像文件格式
- Python Pillow - 为图像添加填充
- Python Pillow - 颜色反转
- Python Pillow 与 NumPy
- Python Pillow 与 Tkinter BitmapImage 和 PhotoImage 对象
- Image 模块
- Python Pillow - 图像混合
- Python Pillow 有用资源
- Python Pillow - 快速指南
- Python Pillow - 函数参考
- Python Pillow - 有用资源
- Python Pillow - 讨论
Python Pillow - ImageOps.posterize() 函数
PIL.ImageOps.posterize 函数用于减少图像中每个颜色通道的位数,有效地限制颜色调色板。
语法
以下是该函数的语法:
PIL.ImageOps.posterize(image, bits)
参数
以下是该函数参数的详细信息:
image - 要进行色调分离的图像。
bits - 每个通道要保留的位数 (1-8)。bits 值决定每个通道的颜色级别数,范围为 1 到 8。
返回值
该函数返回一个新的图像对象,其中输入图像已使用指定的位数进行色调分离。
示例
示例 1
以下示例演示如何创建一个每个通道只有 4 位的新图像。
from PIL import Image, ImageOps # Open an image file input_image = Image.open("Images/flowers.jpg") # Posterize the image with 4 bits per channel posterized_image = ImageOps.posterize(input_image, bits=4) # Display the original and posterized images input_image.show() posterized_image.show()
输出
输入图像
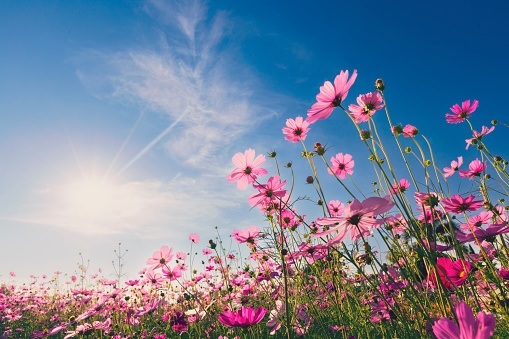
输出图像
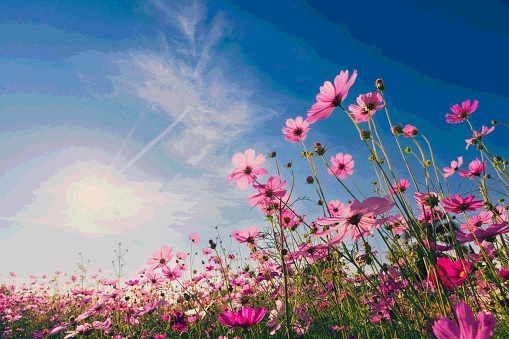
示例 2
这是另一个使用 PIL.ImageOps.posterize 函数处理不同图像的示例。
from PIL import Image, ImageOps # Open an image file input_image = Image.open("Images/Tajmahal_2.jpg") # Posterize the image with 2 bits per channel posterized_image = ImageOps.posterize(input_image, bits=2) # Display the original and posterized images input_image.show() posterized_image.show()
输出
输入图像
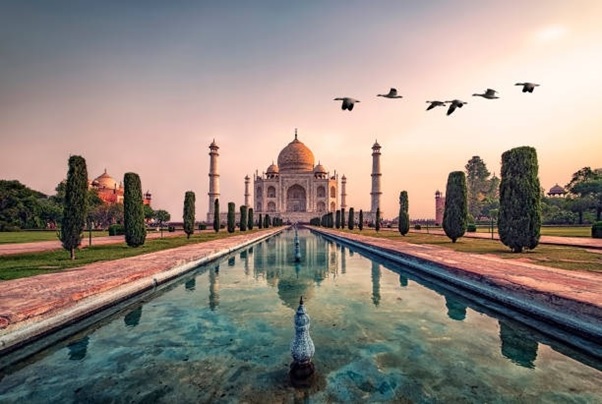
输出图像
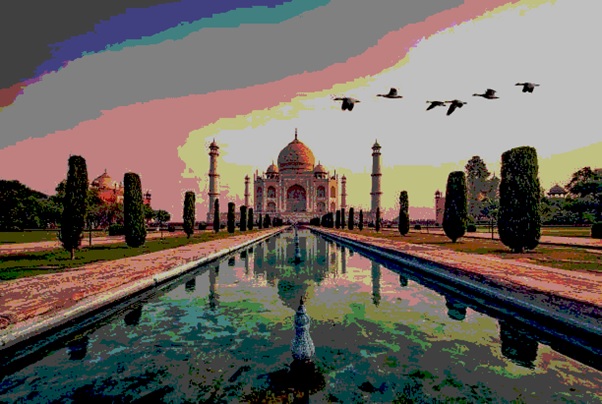
示例 3
在此示例中,代码对图像执行不同位值的色调分离。我们使用 Matplotlib 来显示结果。
from PIL import Image, ImageOps import matplotlib.pyplot as plt # Open the original image original_image = Image.open('Images/flowers_1.jpg') # Create subplots for original and posterized images num_bits_list = [1, 2, 3, 4, 5, 6, 7, 8] fig, axes = plt.subplots(3, 3, figsize=(10, 15)) # Display the original image axes[0, 0].imshow(original_image) axes[0, 0].set_title('Original Image') axes[0, 0].axis('off') # Perform posterization with different bit values and display the images for idx, num_bits in enumerate(num_bits_list, start=1): posterized_image = ImageOps.posterize(original_image, bits=num_bits) # Display the posterized images axes[idx // 3, idx % 3].imshow(posterized_image) axes[idx // 3, idx % 3].set_title(f'Posterized {num_bits} bits') axes[idx // 3, idx % 3].axis('off') plt.tight_layout() plt.show()
输出
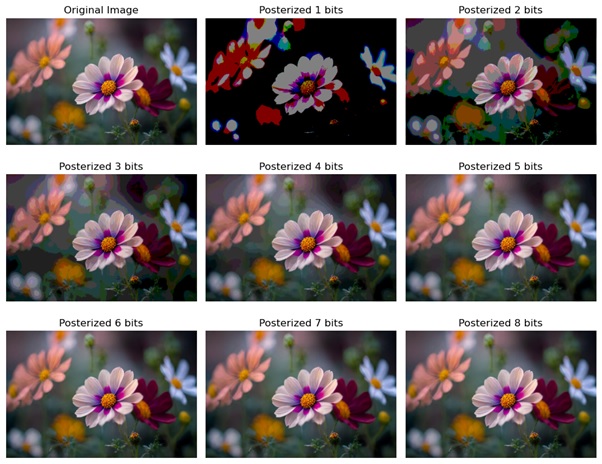
python_pillow_function_reference.htm
广告