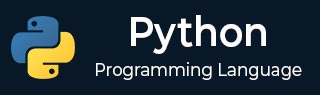
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 修改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组项
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合项
- Python - 添加集合项
- Python - 删除集合项
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 修改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 代码块
- Python - try-finally 代码块
- Python - 抛出异常
- Python - 异常链接
- Python - 嵌套 try 代码块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 加入线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 其他
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
使用 Python 创建语音录音机
在这里,我们将使用 Python 创建一个应用程序(语音录音机),允许用户录制语音。我们将使用 sounddevice 库和 Python 创建此语音录音机应用程序。
使用语音录音机,您可以启动录制过程、暂停它或在必要时取消。录制的声音保存为两种格式 WAV 和 WAVIO。此语音录音机应用程序可用于只需要简单音频录制和回放的应用程序。
所需库
要在 Python 中创建语音录音机,以下为所需的库:
- sounddevice - sounddevice 库将用于捕获音频。
- scipy - 此库将用于使用 write 函数写入 WAV 文件。
- wavio - 此库将用于使用其他选项写入 wav 文件。
- numpy - 此库将用于矩阵或数组计算。
安装
要安装创建语音记录所需的库,您需要使用 PIP 安装这些库。使用以下语句一次安装所有所需的库:
pip install sounddevice scipy wavio numpy
创建语音录音机的步骤
1. 导入库
安装完成后,您需要在代码中导入所有库。要导入所需的库,请使用以下代码语句:
import sounddevice as sd from scipy.io.wavfile import write import wavio as wv import numpy as np import threading
步骤 2:初始化变量
使用以下代码设置采样频率和用于管理录制状态的标志:
- 设置采样频率:
freq = 44100
- 存储录制的音频片段:
recording = []
- 设置默认标志值以检查录制是否处于活动状态:
is_recording = False
- 设置默认标志值以停止录制:
stop_recording = False
步骤 3:定义录制函数
创建一个函数来处理循环中的音频录制,并将每个片段追加到录制列表中。使用以下代码:
def record_audio(): global recording, is_recording, stop_recording is_recording = True while is_recording and not stop_recording: recording_segment = sd.rec(int(freq), samplerate=freq, channels=2) sd.wait() # Wait until the recording is finished recording.append(recording_segment)
步骤 4:定义输入处理函数
实现一个函数来处理用于录制控制(开始、暂停、停止)的用户命令。使用以下代码:
def handle_input(): global is_recording, stop_recording while True: user_input = input("r to START , p to PAUSE and s to STOP the recording, please choice accordingly \n Enter command: ").lower() if user_input == 'p': if is_recording: print("Recording Paused, (you can again start the recording using 'r')") is_recording = False else: print("Recording is not active.") elif user_input == 'r': if not is_recording: print("Recording Resumed (type 'p' to pause or 's' to stop)") threading.Thread(target=record_audio).start() else: print("Already recording.") elif user_input == 's': if is_recording: print("Stopping recording. Thank you for recording :)") is_recording = False stop_recording = True break else: print("Nothing to stop.")
步骤 5:组合并保存录制
停止录制后,将所有录制片段组合成一个音频文件,并将其保存为不同的格式。使用以下代码:
if recording: final_recording = np.concatenate(recording, axis=0) write("recording0.wav", freq, final_recording) wv.write("recording1.wav", final_recording, freq, sampwidth=2) print("Recording saved as 'recording0.wav' and 'recording1.wav'.") else: print("No recording was made.")
创建语音录音机的 Python 代码
以下是使用 Python 创建语音录音机应用程序的完整代码:
import sounddevice as sd from scipy.io.wavfile import write import wavio as wv import numpy as np import threading # Sampling frequency freq = 44100 # Initialize recording variables recording = [] is_recording = False stop_recording = False def record_audio(): global recording, is_recording, stop_recording is_recording = True while is_recording and not stop_recording: recording_segment = sd.rec(int(freq), samplerate=freq, channels=2) sd.wait() recording.append(recording_segment) def handle_input(): global is_recording, stop_recording while True: user_input = input("r to START , p to PAUSE and s to STOP the recording, please choice accordingly \n Enter command: ").lower() if user_input == 'p': if is_recording: print("Recording Paused, (you can again start the recording using 's') ") is_recording = False else: print("Recording is not working activly.") elif user_input == 'r': if not is_recording: print("Recording Resumed (type 'p' to pause or 's' to stop)") threading.Thread(target=record_audio).start() else: print("Already recording.") elif user_input == 's': if is_recording: print("Stopping recording. Thank you for recording :) ") is_recording = False stop_recording = True break else: print(" Nothing to stop.") # Start the input handler in a separate thread input_thread = threading.Thread(target=handle_input) input_thread.start() # Wait for the input thread to complete input_thread.join() # Combine all the recorded segments if recording: final_recording = np.concatenate(recording, axis=0) write("recording0.wav", freq, final_recording) wv.write("recording1.wav", final_recording, freq, sampwidth=2) print("Recording saved as 'recording0.wav' and 'recording1.wav'.") else: print("No recording was made.")
输出
运行此代码后,您将看到按下“r”键开始录制,“p”键暂停,“s”键停止录制。
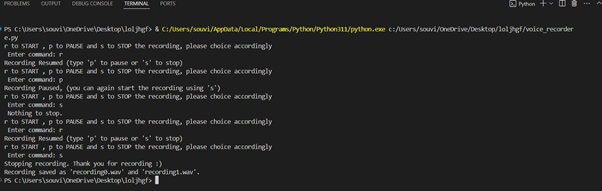
现在您还可以在您的电脑上看到录制的音频文件。
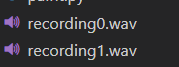
之后,您就可以成功收听您的音频文件了。
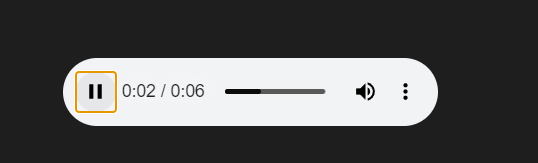
代码解释
- **导入库** - 导入用于录制和处理音频以及管理音频文件的库。
- **初始化变量** - 应设置“采样率”、“录制列表”和“标志”作为录制控制参数。
- **定义 record_audio 函数** - 录制音频片段并将其添加到列表中。
- **定义 handle_input 函数** - 操作用户开始或停止录制过程的指令。
- **启动输入处理线程** - 在线程中管理输入处理函数,以避免游戏冻结。
- **等待输入线程完成** - 在继续执行主线程的后续操作之前,等待输入线程完成执行。
- **合并录制片段** - 将所有录制的音频片段组合成一个轨道或称为片段。
- **以 WAV 格式保存录制** - 通过两种方法将组合后的音频保存为 WAV 文件。
- **打印完成消息** - 让用户知道录制已成功保存。
- **处理无录制场景** - 如果未录制任何音频,则发出警报。
python_reference.htm
广告