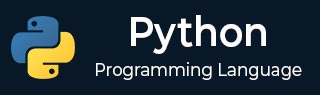
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - match-case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 连接线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - Monkey Patching
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - 内存管理
在 Python 中,**内存管理**是自动的,它涉及处理一个私有堆,其中包含所有 Python 对象和数据结构。Python 内存管理器在内部确保高效地分配和释放此内存。本教程将探讨 Python 的内存管理机制,包括垃圾收集、引用计数以及变量如何在栈和堆中存储。
内存管理组件
Python 的内存管理组件在 Python 程序执行期间提供高效且有效的内存资源利用。Python 有三个内存管理组件:
- 私有堆 (Private Heap):充当所有 Python 对象和数据的存储主区域。它由 Python 内存管理器内部管理。
- 原始内存分配器 (Raw Memory Allocator):这个低级组件直接与操作系统交互,以在 Python 的私有堆中保留内存空间。它确保 Python 的数据结构和对象有足够的存储空间。
- 对象专用分配器 (Object-Specific Allocators):在原始内存分配器之上,一些对象专用分配器管理不同类型对象的内存,例如整数、字符串、元组和字典。
Python 中的内存分配
Python 主要通过两种方式管理内存分配:栈和堆。
栈 - 静态内存分配
在静态内存分配中,内存是在编译时分配的,并存储在栈中。这通常用于函数调用栈和变量引用。栈是一个用于存储局部变量和函数调用信息的内存区域。它基于后进先出 (LIFO) 原则工作,其中最近添加的项目是最先被移除的。
栈通常用于存储基本数据类型的变量,例如数字、布尔值和字符。这些变量具有固定的内存大小,在编译时已知。
示例
让我们来看一个示例,来说明基本类型变量是如何存储在栈中的。在上面的示例中,名为 x、y 和 z 的变量是名为 example_function() 函数中的局部变量。它们存储在栈中,当函数执行完成时,它们会自动从栈中移除。
def my_function(): x = 5 y = True z = 'Hello' return x, y, z print(my_function()) print(x, y, z)
执行上述程序后,您将获得以下输出:
(5, True, 'Hello') Traceback (most recent call last): File "/home/cg/root/71937/main.py", line 8, in <module> print(x, y, z) NameError: name 'x' is not defined
堆 - 动态内存分配
动态内存分配在运行时为非基本类型的对象和数据结构分配内存。这些对象的实际数据存储在堆中,而对它们的引用存储在栈中。
示例
让我们观察一个创建列表的示例,它动态地在堆中分配内存。
a = [0]*10 print(a)
输出
执行上述程序后,您将获得以下结果:
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
Python 中的垃圾收集
Python 中的垃圾收集是自动释放不再被对象使用的内存的过程,使其可用于其他对象。Python 的垃圾收集器在程序执行期间运行,并在对象的引用计数降至零时激活。
引用计数
Python 的主要垃圾收集机制是引用计数。Python 中的每个对象都维护一个引用计数,该计数跟踪指向它的别名(或引用)的数量。当对象的引用计数降至零时,垃圾收集器将释放该对象。
引用计数的工作原理如下:
- 引用计数增加− 当创建对对象的新的引用时,引用计数会增加。
- 引用计数减少− 当对对象的引用被移除或超出作用域时,引用计数会减少。
示例
这是一个演示 Python 中引用计数工作的示例。
import sys # Create a string object name = "Tutorialspoint" print("Initial reference count:", sys.getrefcount(name)) # Assign the same string to another variable other_name = "Tutorialspoint" print("Reference count after assignment:", sys.getrefcount(name)) # Concatenate the string with another string string_sum = name + ' Python' print("Reference count after concatenation:", sys.getrefcount(name)) # Put the name inside a list multiple times list_of_names = [name, name, name] print("Reference count after creating a list with 'name' 3 times:", sys.getrefcount(name)) # Deleting one more reference to 'name' del other_name print("Reference count after deleting 'other_name':", sys.getrefcount(name)) # Deleting the list reference del list_of_names print("Reference count after deleting the list:", sys.getrefcount(name))
输出
执行上述程序后,您将获得以下结果:
Initial reference count: 4 Reference count after assignment: 5 Reference count after concatenation: 5 Reference count after creating a list with 'name' 3 times: 8 Reference count after deleting 'other_name': 7 Reference count after deleting the list: 4