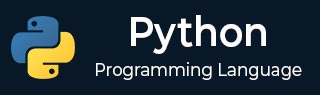
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 对列表进行排序
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 对数组进行排序
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 加入线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - 线程优先级
在 Python 中,目前 **threading** 模块不直接支持线程优先级。与 Java 不同,Python 不支持线程优先级、线程组或某些线程控制机制,例如销毁、停止、挂起、恢复或中断线程。
尽管 Python 线程设计简单,并且松散地基于 Java 的线程 模型。这是由于 Python 的全局解释器锁 (GIL),它管理着 Python 线程。
但是,您可以使用诸如睡眠时长、线程内的自定义调度逻辑或使用管理任务优先级的附加模块等技术来模拟基于优先级的行为。
使用 sleep() 设置线程优先级
您可以通过引入延迟或使用其他机制来控制线程的执行顺序来模拟线程优先级。模拟线程优先级的一种常用方法是调整线程的睡眠时长。
优先级较低的线程睡眠时间较长,而优先级较高的线程睡眠时间较短。
示例
以下是一个简单的示例,演示如何在 Python 线程中使用延迟来自定义线程优先级。在此示例中,Thread-2 在 Thread-1 之前完成,因为它具有较低的优先级值,导致睡眠时间较短。
import threading import time class DummyThread(threading.Thread): def __init__(self, name, priority): threading.Thread.__init__(self) self.name = name self.priority = priority def run(self): name = self.name time.sleep(1.0 * self.priority) print(f"{name} thread with priority {self.priority} is running") # Creating threads with different priorities t1 = DummyThread(name='Thread-1', priority=4) t2 = DummyThread(name='Thread-2', priority=1) # Starting the threads t1.start() t2.start() # Waiting for both threads to complete t1.join() t2.join() print('All Threads are executed')
输出
执行上述程序后,您将获得以下结果:
Thread-2 thread with priority 1 is running Thread-1 thread with priority 4 is running All Threads are executed
在 Windows 上调整 Python 线程优先级
在 Windows 操作系统上,您可以使用 **ctypes** 模块来操作线程优先级,这是 Python 的标准模块之一,用于与 Windows API 交互。
示例
此示例演示了如何在 Windows 系统上使用 **ctypes** 模块手动设置 Python 线程的优先级。
import threading import ctypes import time # Constants for Windows API w32 = ctypes.windll.kernel32 SET_THREAD = 0x20 PRIORITIZE_THE_THREAD = 1 class MyThread(threading.Thread): def __init__(self, start_event, name, iterations): super().__init__() self.start_event = start_event self.thread_id = None self.iterations = iterations self.name = name def set_priority(self, priority): if not self.is_alive(): print('Cannot set priority for a non-active thread') return thread_handle = w32.OpenThread(SET_THREAD, False, self.thread_id) success = w32.SetThreadPriority(thread_handle, priority) w32.CloseHandle(thread_handle) if not success: print('Failed to set thread priority:', w32.GetLastError()) def run(self): self.thread_id = w32.GetCurrentThreadId() self.start_event.wait() while self.iterations: print(f"{self.name} running") start_time = time.time() while time.time() - start_time < 1: pass self.iterations -= 1 # Create an event to synchronize thread start start_event = threading.Event() # Create threads thread_normal = MyThread(start_event, name='normal', iterations=4) thread_high = MyThread(start_event, name='high', iterations=4) # Start the threads thread_normal.start() thread_high.start() # Adjusting priority of 'high' thread thread_high.set_priority(PRIORITIZE_THE_THREAD) # Trigger thread execution start_event.set()
输出
在 Python 解释器中执行此代码时,您将获得以下结果:
high running normal running high running normal running high running normal running high running normal running
使用 Queue 模块优先处理 Python 线程
Python 标准库中的 **queue** 模块在多线程编程中非常有用,此时必须在线程之间安全地交换信息。此模块中的 Priority Queue 类实现了所有必需的锁定语义。
使用优先级队列,条目保持排序(使用 **heapq** 模块),并且首先检索值最低的条目。
Queue 对象具有以下方法来控制队列:
**get()** - get() 从队列中移除并返回一个项目。
**put()** - put() 将项目添加到队列中。
**qsize()** - qsize() 返回当前在队列中的项目数量。
**empty()** - empty() 如果队列为空,则返回 True;否则返回 False。
**full()** - full() 如果队列已满,则返回 True;否则返回 False。
queue.PriorityQueue(maxsize=0)
这是优先级队列的构造函数。maxsize 是一个整数,它设置可以放入队列中的项目的数量上限。如果 maxsize 小于或等于零,则队列大小无限。
值最低的条目首先被检索(值最低的条目是 min(entries) 将返回的条目)。条目的典型模式是以下形式的元组:
(priority_number, data)
示例
此示例演示了如何在queue模块中使用PriorityQueue类来管理两个线程之间的任务优先级。
from time import sleep from random import random, randint from threading import Thread from queue import PriorityQueue queue = PriorityQueue() def producer(queue): print('Producer: Running') for i in range(5): # create item with priority value = random() priority = randint(0, 5) item = (priority, value) queue.put(item) # wait for all items to be processed queue.join() queue.put(None) print('Producer: Done') def consumer(queue): print('Consumer: Running') while True: # get a unit of work item = queue.get() if item is None: break sleep(item[1]) print(item) queue.task_done() print('Consumer: Done') producer = Thread(target=producer, args=(queue,)) producer.start() consumer = Thread(target=consumer, args=(queue,)) consumer.start() producer.join() consumer.join()
输出
执行后,它将产生以下输出:
Producer: Running Consumer: Running (0, 0.15332707626852804) (2, 0.4730737391435892) (2, 0.8679231358257962) (3, 0.051924220435665025) (4, 0.23945882716108446) Producer: Done Consumer: Done