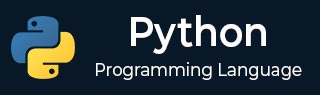
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策制定
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 对列表进行排序
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 对数组进行排序
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 线程连接
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - 读取文件
从文件读取涉及打开文件、读取其内容,然后关闭文件以释放系统资源。Python 提供了几种从文件读取的方法,每种方法都适合不同的用例。
打开文件以进行读取
打开文件是读取其内容的第一步。在 Python 中,您可以使用 open() 函数打开文件。此函数至少需要一个参数,即文件名,以及可选的模式,该模式指定打开文件的目的。
要打开文件以进行读取,您可以使用模式 'r'。这是默认模式,因此如果您只需要从文件读取,则可以省略它。
使用 read() 方法读取文件
read() 方法用于在 Python 中读取文件的内容。它将文件的全部内容作为单个字符串读取。当您需要一次处理整个文件时,此方法特别有用。
语法
以下是 Python 中 read() 方法的基本语法:
file_object.read(size)
其中,
- file_object 是 open() 函数返回的文件对象。
- size 是要从文件读取的字节数。此参数是可选的。如果省略或设置为负值,则该方法会读取到文件末尾。
示例
在下面的示例中,我们以读取模式打开文件“example.txt”。然后,我们使用 read() 方法读取文件的全部内容:
# Open the file in read mode file = open('example.txt', 'r') # Read the entire content of the file content = file.read() # Print the content print(content) # Close the file file.close()
执行上述代码后,我们将获得以下输出:
welcome to Tutorialspoint.
使用 readline() 方法读取文件
readline() 方法用于一次从文件中读取一行。当您需要逐行处理文件时,此方法很有用,尤其是在处理大型文件时,一次读取全部内容是不切实际的。
语法
以下是 Python 中 readline() 方法的基本语法:
file_object.readline(size)
其中,
- file_object 是 open() 函数返回的文件对象。
- size 是一个可选参数,指定从行中读取的最大字节数。如果省略或设置为负值,则该方法会读取到行尾。
示例
在下面的示例中,我们以读取模式打开文件“example.txt”。然后,我们使用 readline() 方法读取文件的首行:
# Open the file in read mode file = open('example.txt', 'r') # Read the first line of the file line = file.readline() # Print the line print(line) # Close the file file.close()
以下是上述代码的输出:
welcome to Tutorialspoint.
使用 readlines() 方法读取文件
readlines() 方法读取文件中的所有行,并将它们作为字符串列表返回。列表中的每个字符串都表示文件中的单行,包括每行末尾的换行符。
当您需要一次处理或分析文件的全部行时,此方法特别有用。
语法
以下是 Python 中 readlines() 方法的基本语法:
file_object.readlines(hint)
其中,
- file_object 是 open() 函数返回的文件对象。
- hint 是一个可选参数,指定要读取的字节数。如果指定,它会读取最多指定字节的行,不一定读取整个文件。
示例
在此示例中,我们以读取模式打开文件“example.txt”。然后,我们使用 readlines() 方法读取文件中的所有行,并将它们作为字符串列表返回:
# Open the file in read mode file = open('example.txt', 'r') # Read all lines from the file lines = file.readlines() # Print the lines for line in lines: print(line, end='') # Close the file file.close()
以下是上述代码的输出:
welcome to Tutorialspoint. Hi Surya. How are you?.
使用“with”语句
Python 中的“with”语句用于异常处理。在处理文件时,使用“with”语句可以确保在读取后正确关闭文件,即使发生异常也是如此。
当您使用with语句打开文件时,即使块内发生错误,文件也会在块结束时自动关闭。
示例
下面是一个使用with语句打开、读取和打印文件内容的简单示例:
# Using the with statement to open a file with open('example.txt', 'r') as file: content = file.read() print(content)
我们得到以下输出:
welcome to Tutorialspoint. Hi Surya. How are you?.
以二进制模式读取文件
默认情况下,文件对象的读/写操作是在文本字符串数据上执行的。如果我们想要处理不同类型的文件,例如媒体文件(mp3)、可执行文件(exe)或图片(jpg),我们必须通过在读/写模式中添加'b'前缀以二进制模式打开文件。
写入二进制文件
假设test.bin文件已以二进制模式写入:
# Open the file in binary write mode with open('test.bin', 'wb') as f: data = b"Hello World" f.write(data)
示例
要读取二进制文件,我们需要以'rb'模式打开它。然后在打印之前解码read()方法的返回值:
# Open the file in binary read mode with open('test.bin', 'rb') as f: data = f.read() print(data.decode(encoding='utf-8'))
它将产生以下输出:
Hello World
从文件中读取整数数据
要将整数数据写入二进制文件,应使用to_bytes()方法将整数对象转换为字节。
将整数写入二进制文件
下面是如何将整数写入二进制文件的示例:
# Convert the integer to bytes and write to a binary file n = 25 data = n.to_bytes(8, 'big') with open('test.bin', 'wb') as f: f.write(data)
从二进制文件中读取整数
要从二进制文件中读取回整数数据,请使用from_bytes()方法将read()函数的输出转换回整数:
# Read the binary data from the file and convert it back to an integer with open('test.bin', 'rb') as f: data = f.read() n = int.from_bytes(data, 'big') print(n)
从文件中读取浮点数数据
为了处理二进制文件中的浮点数数据,我们需要使用Python标准库中的struct模块。此模块有助于在Python值和表示为Python字节对象的C结构之间进行转换。
将浮点数写入二进制文件
要将浮点数数据写入二进制文件,我们使用struct.pack()方法将浮点数转换为字节对象:
import struct # Define a floating-point number x = 23.50 # Pack the float into a binary format data = struct.pack('f', x) # Open the file in binary write mode and write the packed data with open('test.bin', 'wb') as f: f.write(data)
从二进制文件中读取浮点数
要从二进制文件中读取浮点数数据,我们使用struct.unpack()方法将字节对象转换回浮点数:
import struct # Open the file in binary read mode with open('test.bin', 'rb') as f: # Read the binary data from the file data = f.read() # Unpack the binary data to retrieve the float x = struct.unpack('f', data)[0] # Print the float value print(x)
使用“r+”模式读取和写入文件
当文件以读取模式(使用'r'或'rb')打开时,除非文件关闭并以不同的模式重新打开,否则无法写入数据。要同时执行读写操作,我们将'+'字符添加到mode参数中。使用'w+'或'r+'模式可以启用write()和read()方法,而无需关闭文件。
File对象还支持seek()函数,该函数允许将读/写指针重新定位到文件中的任何所需字节位置。
语法
以下是seek()方法的语法:
fileObject.seek(offset[, whence])
参数
offset - 这是文件内读/写指针的位置。
whence - 这是可选的,默认为0,表示绝对文件定位,其他值为1,表示相对于当前位置查找,2表示相对于文件末尾查找。
示例
以下程序以'r+'模式(读写模式)打开文件,在文件中查找特定位置,并从该位置读取数据:
# Open the file in read-write mode with open("foo.txt", "r+") as fo: # Move the read/write pointer to the 10th byte position fo.seek(10, 0) # Read 3 bytes from the current position data = fo.read(3) # Print the read data print(data)
执行上述代码后,我们将获得以下输出:
rat
在Python中同时读取和写入文件
当文件以写入模式(使用'w'或'a')打开时,无法从中读取,尝试这样做会引发UnsupportedOperation错误。
类似地,当文件以读取模式(使用'r'或'rb')打开时,不允许写入它。要在读取和写入之间切换,通常需要关闭文件并以所需的模式重新打开它。
要同时执行读写操作,可以将'+'字符添加到mode参数中。使用'w+'或'r+'模式可以启用write()和read()方法,而无需关闭文件。
此外,File对象支持seek()函数,该函数允许您将读/写指针重新定位到文件中的任何所需字节位置。
示例
在此示例中,我们以'r+'模式打开文件并将数据写入文件。seek(0)方法将指针重新定位到文件开头:
# Open the file in read-write mode with open("foo.txt", "r+") as fo: # Write data to the file fo.write("This is a rat race") # Rewind the pointer to the beginning of the file fo.seek(0) # Read data from the file data = fo.read() print(data)
从特定偏移量读取文件
我们可以使用seek()方法将文件的当前位置设置为指定的偏移量。
- 如果文件使用'a'或'a+'以追加模式打开,则在下次写入时将撤消任何seek()操作。
- 如果文件仅以追加模式使用'a'打开以进行写入,则此方法本质上是无操作的,但对于以启用读取的追加模式打开的文件(模式'a+')仍然有用。
- 如果文件使用't'以文本模式打开,则只有tell()返回的偏移量才是合法的。使用其他偏移量会导致未定义的行为。
请注意,并非所有文件对象都是可查找的。
示例
以下示例演示如何使用seek()方法对文件执行同时读写操作。文件以w+模式(读写模式)打开,添加了一些数据,然后在特定位置读取和修改文件:
# Open a file in read-write mode fo = open("foo.txt", "w+") # Write initial data to the file fo.write("This is a rat race") # Seek to a specific position in the file fo.seek(10, 0) # Read a few bytes from the current position data = fo.read(3) print("Data read from position 10:", data) # Seek back to the same position fo.seek(10, 0) # Overwrite the earlier contents with new text fo.write("cat") # Rewind to the beginning of the file fo.seek(0, 0) # Read the entire file content data = fo.read() print("Updated file content:", data) # Close the file fo.close()
以下是上述代码的输出:
Data read from position 10: rat Updated file content: This is a cat race