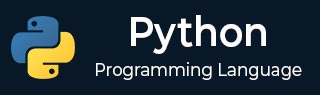
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 修改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组项
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合项
- Python - 添加集合项
- Python - 删除集合项
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 修改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 合并线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python for-else 循环
Python - For Else 循环
Python 支持将一个可选的else 块与for 循环关联。如果else 块与for 循环一起使用,则只有当 for 循环正常终止时才会执行它。
当 for 循环在没有遇到break 语句的情况下完成所有迭代时,for 循环会正常终止,这允许我们在满足特定条件时退出循环。
For Else 循环流程图
下面的流程图说明了for-else 循环的使用:
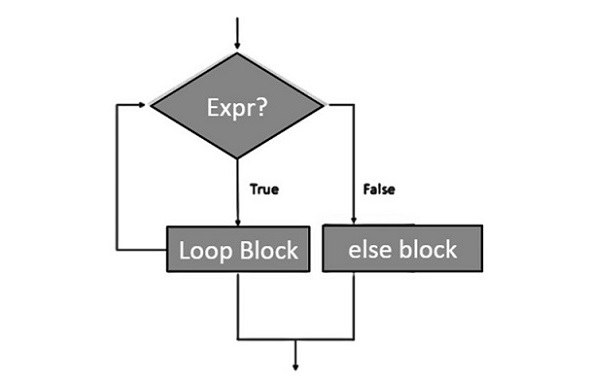
For Else 循环语法
以下是带可选 else 块的 for 循环的语法:
for variable_name in iterable: #stmts in the loop . . . else: #stmts in else clause . .
For Else 循环示例
以下示例说明了在Python中将 else 语句与 for 语句结合使用的情况。在计数小于 5 之前,会打印迭代计数。当它变为 5 时,else 块中的 print 语句会在控制权传递到主程序中的下一条语句之前执行。
for count in range(6): print ("Iteration no. {}".format(count)) else: print ("for loop over. Now in else block") print ("End of for loop")
执行此代码将产生以下输出:
Iteration no. 1 Iteration no. 2 Iteration no. 3 Iteration no. 4 Iteration no. 5 for loop over. Now in else block End of for loop
不带 break 语句的 For-Else 结构
如本教程前面所述,else 块仅在循环正常终止时(即不使用 break 语句)才会执行。
示例
在下面的程序中,我们使用不带 break 语句的 for-else 循环。
for i in ['T','P']: print(i) else: # Loop else statement # there is no break statement in for loop, hence else part gets executed directly print("ForLoop-else statement successfully executed")
执行上述程序将生成以下输出:
T P ForLoop-else statement successfully executed
带 break 语句的 For-Else 结构
如果循环被强制终止(使用break语句),解释器会忽略else语句,因此会跳过它的执行。
示例
以下程序演示了else条件在break语句中的工作方式。
for i in ['T','P']: print(i) break else: # Loop else statement # terminated after 1st iteration due to break statement in for loop print("Loop-else statement successfully executed")
执行上述程序将生成以下输出:
T
带break语句和if条件的For-Else
如果我们将for-else结构与break语句和if条件一起使用,for循环将遍历迭代器,并且在这个循环中,可以使用if块来检查特定条件。如果循环在不遇到break语句的情况下完成,则执行else块中的代码。
示例
以下程序演示了else条件在break语句和条件语句中的工作方式。
# creating a function to check whether the list item is a positive # or a negative number def positive_or_negative(): # traversing in a list for i in [5,6,7]: # checking whether the list element is greater than 0 if i>=0: # printing positive number if it is greater than or equal to 0 print ("Positive number") else: # Else printing Negative number and breaking the loop print ("Negative number") break # Else statement of the for loop else: # Statement inside the else block print ("Loop-else Executed") # Calling the above-created function positive_or_negative()
执行上述程序将生成以下输出:
Positive number Positive number Positive number Loop-else Executed
广告