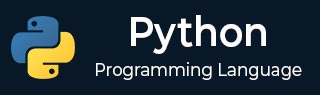
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组项
- Python - 更新元组
- Python - 解包元组
- Python - 循环元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合项
- Python - 添加集合项
- Python - 删除集合项
- Python - 循环集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装器类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 加入线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - 更改字典项
更改字典项
在 Python 中更改字典项是指修改字典中与特定键关联的值。这可能包括更新现有键的值、添加新的键值对或从字典中删除键值对。
字典是可变的,这意味着在创建后可以修改其内容。
修改字典值
修改 Python 字典中的值是指更改与现有键关联的值。为此,您可以直接将新值赋给该键。
示例
在以下示例中,我们定义了一个名为“person”的字典,其中包含键“name”、“age”和“city”及其对应值。然后,我们将与键“age”关联的值修改为 26 -
# Initial dictionary person = {'name': 'Alice', 'age': 25, 'city': 'New York'} # Modifying the value associated with the key 'age' person['age'] = 26 print(person)
它将产生以下输出 -
{'name': 'Alice', 'age': 26, 'city': 'New York'}
更新多个字典值
如果您需要一次更新字典中的多个值,可以使用 update() 方法。此方法用于使用来自另一个字典或键值对的可迭代对象的元素来更新字典。
update() 方法将提供的字典或可迭代对象中的键值对添加到原始字典中,如果它们已存在于原始字典中,则用新值覆盖任何现有键。
示例
在下面的示例中,我们使用 update() 方法修改“persons”字典中与键“age”和“city”关联的值 -
# Initial dictionary person = {'name': 'Alice', 'age': 25, 'city': 'New York'} # Updating multiple values person.update({'age': 26, 'city': 'Los Angeles'}) print(person)
我们得到的输出如下所示 -
{'name': 'Alice', 'age': 26, 'city': 'Los Angeles'}
条件字典修改
Python 字典中的条件修改是指仅在满足特定条件时才更改与键关联的值。
您可以使用 if 语句检查特定条件是否为真,然后再修改与键关联的值。
示例
在此示例中,如果“persons”字典中键“age”的当前值为“25”,则将其关联的值有条件地修改为“26” -
# Initial dictionary person = {'name': 'Alice', 'age': 25, 'city': 'New York'} # Conditionally modifying the value associated with 'age' if person['age'] == 25: person['age'] = 26 print(person)
获得的输出如下所示 -
{'name': 'Alice', 'age': 26, 'city': 'New York'}
通过添加新的键值对修改字典
将新的键值对添加到 Python 字典中是指将新的键及其对应值插入到字典中。
此过程允许您根据需要通过包含其他信息来动态扩展存储在字典中的数据。
示例:使用赋值运算符
您可以通过直接将值赋给新键来将新的键值对添加到字典中,如下所示。在下面的示例中,键“city”与值“New York”一起添加到“person”字典中 -
# Initial dictionary person = {'name': 'Alice', 'age': 25} # Adding a new key-value pair 'city': 'New York' person['city'] = 'New York' print(person)
产生的结果如下 -
{'name': 'Alice', 'age': 25, 'city': 'New York'}
示例:使用 setdefault() 方法
您可以使用 setdefault() 方法将新的键值对添加到字典中,前提是该键尚不存在。
在此示例中,setdefault() 方法仅当键“city”尚不存在时才将新键“city”与值“New York”一起添加到“person”字典中 -
# Initial dictionary person = {'name': 'Alice', 'age': 25} # Adding a new key-value pair 'city': 'New York' person.setdefault('city', 'New York') print(person)
以下是上述代码的输出 -
{'name': 'Alice', 'age': 25, 'city': 'New York'}
通过删除键值对修改字典
从 Python 字典中删除键值对是指从字典中删除特定的键及其对应值。
此过程允许您根据要消除的键选择性地从字典中删除数据。
示例:使用 del 语句
您可以使用 del 语句从字典中删除特定的键值对。在此示例中,del 语句从“person”字典中删除键“age”及其关联的值 -
# Initial dictionary person = {'name': 'Alice', 'age': 25, 'city': 'New York'} # Removing the key-value pair associated with the key 'age' del person['age'] print(person)
上述代码的输出如下所示 -
{'name': 'Alice', 'city': 'New York'}
示例:使用 pop() 方法
您还可以使用pop()方法从字典中删除特定的键值对,并返回与已删除键关联的值。
在这里,pop()方法从“person”字典中删除键“age”及其关联的值 -
# Initial dictionary person = {'name': 'Alice', 'age': 25, 'city': 'New York'} # Removing the key-value pair associated with the key 'age' removed_age = person.pop('age') print(person) print("Removed age:", removed_age)
它将产生以下输出 -
{'name': 'Alice', 'city': 'New York'} Removed age: 25
示例:使用popitem()方法
您也可以使用popitem()方法删除字典中的最后一个键值对,并将其作为元组返回。
现在,popitem()方法从“person”字典中删除最后一个键值对,并将其作为元组返回 -
# Initial dictionary person = {'name': 'Alice', 'age': 25, 'city': 'New York'} # Removing the last key-value pair removed_item = person.popitem() print(person) print("Removed item:", removed_item)
我们得到的输出如下所示 -
{'name': 'Alice', 'age': 25} Removed item: ('city', 'New York')