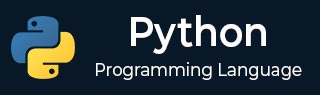
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策制定
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 修改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组项
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合项
- Python - 添加集合项
- Python - 删除集合项
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 修改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 线程连接
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - 线程同步
在 Python 中,当多个线程并发地操作共享资源时,同步它们的访问以维护数据完整性和程序正确性非常重要。Python 中的线程同步可以通过使用 **threading** 模块提供的各种同步原语来实现,例如锁、条件、信号量和屏障,以控制对共享资源的访问并协调多个线程的执行。
在本教程中,我们将学习 Python 的 **threading** 模块提供的各种同步原语。
使用锁进行线程同步
Python 的 threading 模块中的锁对象提供了最简单的同步原语。它们允许线程在代码的关键部分获取和释放锁,确保一次只有一个线程可以执行受保护的代码。
通过调用 **Lock()** 方法创建一个新的锁,该方法返回一个锁对象。可以使用 **acquire(blocking)** 方法获取锁,该方法强制线程同步运行。可选的 blocking 参数允许您控制线程是否等待获取锁,并使用 **release()** 方法释放锁。
示例
以下示例演示了如何在 Python 中使用锁(threading.Lock() 方法)来同步线程,确保多个线程安全正确地访问共享资源。
import threading counter = 10 def increment(theLock, N): global counter for i in range(N): theLock.acquire() counter += 1 theLock.release() lock = threading.Lock() t1 = threading.Thread(target=increment, args=[lock, 2]) t2 = threading.Thread(target=increment, args=[lock, 10]) t3 = threading.Thread(target=increment, args=[lock, 4]) t1.start() t2.start() t3.start() # Wait for all threads to complete for thread in (t1, t2, t3): thread.join() print("All threads have completed") print("The Final Counter Value:", counter)
输出
执行上述代码时,会产生以下输出:
All threads have completed The Final Counter Value: 26
用于同步 Python 线程的条件对象
条件变量允许线程等待,直到被另一个线程通知。它们对于提供线程之间的通信非常有用。wait()方法用于阻塞一个线程,直到它被另一个线程通过notify()或notify_all()通知。
示例
此示例演示了Condition对象如何使用notify()和wait()方法来同步线程。
import threading counter = 0 # Consumer function def consumer(cv): global counter with cv: print("Consumer is waiting") cv.wait() # Wait until notified by increment print("Consumer has been notified. Current Counter value:", counter) # increment function def increment(cv, N): global counter with cv: print("increment is producing items") for i in range(1, N + 1): counter += i # Increment counter by i # Notify the consumer cv.notify() print("Increment has finished") # Create a Condition object cv = threading.Condition() # Create and start threads consumer_thread = threading.Thread(target=consumer, args=[cv]) increment_thread = threading.Thread(target=increment, args=[cv, 5]) consumer_thread.start() increment_thread.start() consumer_thread.join() increment_thread.join() print("The Final Counter Value:", counter)
输出
执行上述程序后,将产生以下输出:
Consumer is waiting increment is producing items Increment has finished Consumer has been notified. Current Counter value: 15 The Final Counter Value: 15
使用join()方法同步线程
Python的threading模块中的join()方法用于等待所有线程完成执行。这是一种同步主线程与其他线程完成的简单方法。
示例
这演示了使用join()方法同步线程,以确保主线程在继续执行之前等待所有启动的线程完成其工作。
import threading import time class MyThread(threading.Thread): def __init__(self, threadID, name, counter): threading.Thread.__init__(self) self.threadID = threadID self.name = name self.counter = counter def run(self): print("Starting " + self.name) print_time(self.name, self.counter, 3) def print_time(threadName, delay, counter): while counter: time.sleep(delay) print("%s: %s" % (threadName, time.ctime(time.time()))) counter -= 1 threads = [] # Create new threads thread1 = MyThread(1, "Thread-1", 1) thread2 = MyThread(2, "Thread-2", 2) # Start the new Threads thread1.start() thread2.start() # Join the threads thread1.join() thread2.join() print("Exiting Main Thread")
输出
执行上述程序后,将产生以下输出:
Starting Thread-1 Starting Thread-2 Thread-1: Mon Jul 1 16:05:14 2024 Thread-2: Mon Jul 1 16:05:15 2024 Thread-1: Mon Jul 1 16:05:15 2024 Thread-1: Mon Jul 1 16:05:16 2024 Thread-2: Mon Jul 1 16:05:17 2024 Thread-2: Mon Jul 1 16:05:19 2024 Exiting Main Thread
其他同步原语
除了上述同步原语之外,Python的threading模块还提供:−
- RLocks(可重入锁):锁的一种变体,允许线程在释放之前多次获取相同的锁,这在递归函数或嵌套函数调用中很有用。
- 信号量:类似于锁,但带有一个计数器。线程可以获取信号量,直到初始化时定义的某个限制。信号量对于限制对具有固定容量的资源的访问很有用。
- 屏障:允许固定数量的线程在屏障点同步,并且只有在所有线程都到达该点后才能继续执行。屏障对于协调必须全部完成某个执行阶段才能继续执行的线程组很有用。