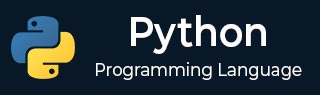
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - If 语句
- Python - If else
- Python - 嵌套 If
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - While 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 对列表进行排序
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 对数组进行排序
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 加入线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - 上下文管理器
Python 中的上下文管理器提供了一种强大且安全的方式来有效管理资源。Python 中的上下文管理器是一个对象,它定义了一个与with语句一起使用的运行时上下文。它确保自动执行设置和清理操作。
例如,在处理文件操作时,上下文管理器会处理文件的打开和关闭,确保正确管理资源。
上下文管理器的工作原理?
Python 上下文管理器通过实现__enter__()和__exit__()方法(或异步操作的异步等效方法)来工作。这些方法确保正确获取和释放资源。此外,Python 的contextlib模块进一步简化了自定义上下文管理器的创建。
示例
以下是一个简单的示例,演示了上下文管理器如何在 Python 中与文件操作一起工作。
with open('example.txt', 'w') as file: file.write('Hello, Tutorialspoint!')
在此示例中,文件以写入模式打开,然后在退出with语句内的代码块时自动关闭。
Python 上下文管理器类型
Python 支持同步和异步上下文管理器。每种类型都有需要实现的特定方法来管理上下文的生命周期。
同步上下文管理器
同步上下文管理器使用__enter__()和__exit__()方法实现。
1. __enter__() 方法
当执行进入 with 语句的上下文时,将调用__enter__(self)方法。此方法应返回要在 with 代码块内使用的资源。
示例
以下是如何使用__enter__()和__exit__()方法创建我们自己的上下文管理器的简单示例。
class MyContextManager: def __enter__(self): print("Entering the context") return self def __exit__(self, exc_type, exc_value, traceback): print("Exiting the context") with MyContextManager(): print("body")
执行以上代码,您将得到以下输出 -
Entering the context body Exiting the context
2. __exit__() 方法
__exit__(self, exc_type, exc_value, traceback) 方法在执行离开 with 语句上下文时被调用。它可以处理任何发生的异常,并返回一个布尔标志,指示是否应该抑制异常。
此示例演示了如何创建我们自己的上下文管理器以及 __exit__() 方法如何处理异常。
class MyContextManager: def __enter__(self): print("Entering the context") return self def __exit__(self, exc_type, exc_value, traceback): print("Exiting the context") if exc_type: print("An exception occurred") return True # Suppress exception with MyContextManager(): print("body") name = "Python"/3 #to raise an exception
在执行以上代码时,您将获得以下输出 -
Entering the context body Exiting the context An exception occurred
异步上下文管理器
与同步上下文管理器类似,异步上下文管理器也使用两个方法实现,即 __aenter__() 和 __aexit__()。这些用于async with 语句中。
__aenter__(self) 方法 - 它必须返回一个可等待对象,当进入上下文时将被等待。
__aexit__(self, exc_type, exc_value, traceback) 方法 - 它必须返回一个可等待对象,当退出上下文时将被等待。
示例
以下是创建异步上下文管理器类的示例 -
import asyncio class AsyncContextManager: async def __aenter__(self): print("Entering the async context class") return self async def __aexit__(self, exc_type, exc_value, traceback): print("Exiting the async context class") if exc_type: print("Exception occurred") return True async def main(): async with AsyncContextManager(): print("Inside the async context") name = "Python"/3 #to raise an exception asyncio.run(main())
执行以上代码,您将获得以下输出 -
Entering the async context class Inside the async context Exiting the async context class Exception occurred
创建自定义上下文管理器
Python 标准库中的contextlib 模块提供了更轻松地创建上下文管理器的实用程序。
使用 contextlib.contextmanager() 函数
contextlib.contextmanager() 函数是一个装饰器,允许您为 with 语句上下文管理器创建工厂函数。它消除了定义单独的类或分别实现__enter__() 和__exit__() 方法的需要。
示例
这是一个使用 contextlib.contextmanager 创建上下文管理器函数的示例。
from contextlib import contextmanager @contextmanager def my_context_manager(): print("Entering the context manager method") try: yield finally: print("Exiting the context manager method") with my_context_manager(): print("Inside the context")
执行以上代码,您将获得以下输出 -
Entering the context manager method Inside the context Exiting the context manager method
使用 contextlib.asynccontextmanager() 函数
contextlib 模块还提供 asynccontextmanager,专门用于创建异步上下文管理器。它类似于 contextmanager,并且消除了定义单独的类或分别实现__aenter__() 和__aexit__() 方法的需要。
示例
这是一个演示使用 contextlib.asynccontextmanager() 创建异步上下文管理器函数的示例。
import asyncio from contextlib import asynccontextmanager @asynccontextmanager async def async_context_manager(): try: print("Entering the async context") # Perform async setup tasks if needed yield finally: # Perform async cleanup tasks if needed print("Exiting the async context") async def main(): async with async_context_manager(): print("Inside the async context") await asyncio.sleep(1) # Simulating an async operation # Run the asyncio event loop asyncio.run(main())
执行以上代码,您将获得以下输出 -
Entering the async context Inside the async context Exiting the async context