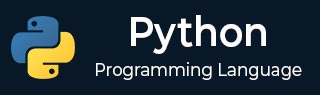
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - match-case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数 & 模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串拼接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类 & 对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误 & 异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 加入线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - Socket 编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期 & 时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - Monkey Patching
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python - 类方法
方法属于类的对象,用于执行特定操作。我们可以将 Python 方法分为三类:类方法、实例方法和静态方法。
Python 的类方法是一种绑定到类而不是类实例的方法。它可以在类本身而不是类的实例上调用。
我们大多数人经常将类方法与静态方法混淆。记住,虽然两者都在类上调用,但静态方法无法访问“cls”参数,因此它无法修改类状态。
与类方法不同,实例方法可以访问对象的实例变量。它还可以访问类变量,因为类变量对所有对象都是通用的。
在 Python 中创建类方法
在 Python 中创建类方法有两种方法:
- 使用 classmethod() 函数
- 使用 @classmethod 装饰器
使用 classmethod() 函数
Python 有一个内置函数classmethod(),它可以将实例方法转换为类方法,该类方法只能用类引用调用,而不能用对象调用。
语法
classmethod(instance_method)
示例
在 Employee 类中,定义一个带有“self”参数(对调用对象的引用)的 showcount() 实例方法。它打印 empCount 的值。接下来,将该方法转换为可以通过类引用访问的类方法 counter()。
class Employee: empCount = 0 def __init__(self, name, age): self.__name = name self.__age = age Employee.empCount += 1 def showcount(self): print (self.empCount) counter = classmethod(showcount) e1 = Employee("Bhavana", 24) e2 = Employee("Rajesh", 26) e3 = Employee("John", 27) e1.showcount() Employee.counter()
输出
使用对象调用 showcount(),使用类调用 count(),两者都显示员工计数的值。
3 3
使用 @classmethod 装饰器
使用@classmethod()装饰器是定义类方法的首选方法,因为它比先声明实例方法然后将其转换为类方法更方便。
语法
@classmethod def method_name(): # your code
示例
类方法充当备选构造函数。定义一个带有构造新对象所需参数的newemployee()类方法。它返回构造的对象,这与__init__()方法的作用相同。
class Employee: empCount = 0 def __init__(self, name, age): self.name = name self.age = age Employee.empCount += 1 @classmethod def showcount(cls): print (cls.empCount) @classmethod def newemployee(cls, name, age): return cls(name, age) e1 = Employee("Bhavana", 24) e2 = Employee("Rajesh", 26) e3 = Employee("John", 27) e4 = Employee.newemployee("Anil", 21) Employee.showcount()
现在有四个 Employee 对象。如果我们运行上述程序,它将显示 Employee 对象的计数:
4
在类方法中访问类属性
类属性是属于类并且其值在该类的所有实例之间共享的那些变量。
要在类方法中访问类属性,请使用cls 参数,后跟点 (.) 表示法和属性的名称。
示例
在这个例子中,我们在类方法中访问类属性。
class Cloth: # Class attribute price = 4000 @classmethod def showPrice(cls): return cls.price # Accessing class attribute print(Cloth.showPrice())
运行上述代码后,将显示以下输出:
4000
动态地向类添加类方法
Python 的 setattr() 函数用于动态设置属性。如果要向类添加类方法,请将方法名称作为参数值传递给 setattr() 函数。
示例
以下示例演示如何动态地向 Python 类添加类方法。
class Cloth: pass # class method @classmethod def brandName(cls): print("Name of the brand is Raymond") # adding dynamically setattr(Cloth, "brand_name", brandName) newObj = Cloth() newObj.brand_name()
执行以上代码后,将显示以下输出:
Name of the brand is Raymond
动态删除类方法
Python 的 del 运算符用于动态删除类方法。如果尝试访问已删除的方法,代码将引发 AttributeError。
示例
在下面的示例中,我们使用 del 运算符删除名为“brandName”的类方法。
class Cloth: # class method @classmethod def brandName(cls): print("Name of the brand is Raymond") # deleting dynamically del Cloth.brandName print("Method deleted")
执行以上代码后,将显示以下输出:
Method deleted