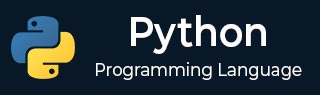
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策制定
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - match-case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 列表排序
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 等待线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 其他
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python 字典 get() 方法
Python 字典 get() 方法用于检索与指定键对应的值。
此方法接受键和值,其中值是可选的。如果字典中找不到键,但指定了值,则此方法将检索指定的值。另一方面,如果字典中找不到键并且也没有指定值,则此方法将检索 None。
在使用字典时,通常的做法是检索字典中指定键的值。例如,如果您经营一家书店,您可能希望了解您收到了多少本书的订单。
语法
以下是Python 字典 get() 方法的语法:
dict.get(key, value)
参数
此方法接受如下所示的两个参数
key − 这是要在字典中搜索的键。
value (optional) − 如果指定的键不存在,则返回此值。其默认值为 None。
返回值
此方法返回给定键的值。
如果键不可用且值也没有给出,则它返回默认值 None。
如果未给出键但指定了值,则它返回此值。
示例
如果作为参数传递给 get() 方法的键存在于字典中,则它将返回其对应的值。
以下示例显示了 Python 字典 get() 方法的用法。这里创建了一个包含键:“Name”和“Age”的字典 'dict'。然后,将键 'Age' 作为参数传递给该方法。因此,其对应的值 '7' 从字典中检索。
# creating the dictionary dict = {'Name': 'Zebra', 'Age': 7} # printing the value of the given key print ("Value : %s" % dict.get('Age'))
运行上述程序时,它会产生以下结果:
Value : 7
示例
如果作为参数传递的键不存在于字典中,并且也没有指定值,则此方法将返回默认值 None。
在这里,键 'Roll_No' 作为参数传递给 get() 方法。此键在字典 'dict' 中找不到,并且也没有指定值。因此,使用 dict.get() 方法返回默认值 'None'。
# creating the dictionary dict = {'Name': 'Rahul', 'Age': 7} # printing the value of the given key print ("The value is: ", dict.get('Roll_No'))
以下是上述代码的输出:
The value is: None
示例
如果我们传递一个键及其值作为参数,而该键不存在于字典中,则此方法将返回指定的值。
在下面的代码中,键 'Education' 和值 'Never' 作为参数传递给 dict.get() 方法。由于在字典 'dict' 中找不到给定的键,因此返回当前值。
# creating the dictionary dict = {'Name': 'Zebra', 'Age': 7} res = dict.get('Education', "Never") print ("Value : %s" % res)
执行上述代码时,我们将得到以下输出:
Value : Never
示例
在下面给出的示例中,创建了一个嵌套字典 'dict_1'。然后使用嵌套的 get() 方法返回给定键的值。
dict_1 = {'Universe' : {'Planet' : 'Earth'}} print("The dictionary is: ", dict_1) # using nested get() method result = dict_1.get('Universe', {}).get('Planet') print("The nested value obtained is: ", result)
上述代码的输出如下:
The dictionary is: {'Universe': {'Planet': 'Earth'}} The nested value obtained is: Earth