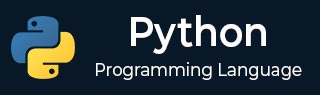
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 循环遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 循环遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 循环遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 循环遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 循环遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 文件重命名和删除
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 线程连接
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络编程
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUIs
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - Monkey Patching
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
Python 文件 seek() 方法
Python 文件seek()方法将文件的游标设置在当前文件的指定位置。文件的游标用于存储文件读取和写入操作的当前位置;此方法可以向前或向后移动此文件游标。
例如,每当我们打开一个文件进行读取时,文件游标总是位于 0 位置。当我们遍历文件内容时,它会逐渐递增。但是,某些情况下需要从文件的特定位置读取文件。这就是此方法发挥作用的地方。
让我们看看使用 seek() 方法的各种情况:
- 如果使用 'a' 或 'a+' 以追加模式打开文件,则在下次写入时将撤消任何 seek() 操作。
- 如果仅使用 'a' 以追加模式打开文件进行写入,则此方法本质上是一个无操作,但对于以启用读取的追加模式(模式 'a+')打开的文件仍然有用。
- 如果使用 't' 以文本模式打开文件,则只有 tell() 返回的偏移量才是合法的。使用其他偏移量会导致未定义的行为。
- 所有文件对象都是可查找的。
语法
以下是 Python 文件seek()方法的语法:
fileObject.seek(offset[, whence])
参数
offset − 这是在文件中移动读/写指针的位置数。
whence − (可选)默认为 0;这意味着绝对文件定位,其他值为 1,这意味着相对于当前位置查找,2 意味着相对于文件末尾查找。
返回值
此方法不返回值。它只是将游标设置在指定的偏移量。
示例
考虑一个包含 5 行的演示文件“foo.txt”。
This is 1st line This is 2nd line This is 3rd line This is 4th line This is 5th line
以下示例显示了 Python 文件 seek() 方法的用法。我们试图将指针设置在文件的开头。因此,我们将偏移量参数传递给此方法为 0。
# Open a file fo = open("foo.txt", "r+") print("Name of the file: ", fo.name) # Assuming file has following 5 lines # This is 1st line # This is 2nd line # This is 3rd line # This is 4th line # This is 5th line line = fo.readline() print("Read Line:", line) # Again set the pointer to the beginning fo.seek(0, 0) line = fo.readline() print("Read Line:", line) # Close opened file fo.close()
当我们运行上面的程序时,它会产生以下结果:
Name of the file: foo.txt Read Line: This is 1st line Read Line: This is 1st line
示例
也可以相对于其在文件中的当前位置设置指针的新位置。为此,我们必须始终以二进制模式打开文件:它可以是读取二进制或写入二进制模式。然后,我们将要从当前位置移动的位置数作为偏移量参数传递,并将值 1 作为 whence 参数传递给该方法。
# Open a file fo = open("foo.txt", "rb") print("Name of the file: ", fo.name) # Set the pointer to the new position relative to current position fo.seek(18, 1) line = fo.read() print("File Contents:", line) # Close opened file fo.close()
执行给定程序后产生的输出将采用二进制形式。但可以使用 decode() 方法将其解码为字符串。
Name of the file: foo.txt File Contents: b'This is 2nd line\r\nThis is 3rd line\r\nThis is 4th line\r\nThis is 5th line'
示例
但是,在某些情况下,我们需要将指针设置在文件的末尾;例如,使用读写 (r+) 模式将信息追加到现有文件中。让我们看下面的一个示例,它在名为“foo.txt”的文件上演示了这一点。
# Open a file fo = open("foo.txt", "r+") print("Name of the file: ", fo.name) # Set the pointer to the ending fo.seek(0, 2) fo.write("This is the end of file") # Again set the pointer at the beginning fo.seek(0, 0) line = fo.read() print("File Contents:", line) # Close opened file fo.close()
一旦我们编译并运行上面的程序,输出将如下所示:
Name of the file: foo.txt File Contents: This is 1st line This is 2nd line This is 3rd line This is 4th line This is 5th lineThis is the end of file
示例
正如我们上面已经讨论过的,文件的游标也可以从文件的末尾向后移动;或者从某个位置。在给定的示例中,我们以读取二进制 (rb) 模式打开文件“foo.txt”。文件游标通过将 whence 参数设置为 2 来设置在文件末尾,并且通过将负值作为偏移量参数传递给该方法,我们试图将文件游标向后移动。
# Open a file fo = open("foo.txt", "rb") print("Name of the file: ", fo.name) # Move the pointer backwards using negative offset fo.seek(-36, 2) line = fo.read() print("File Contents:", line) # Close opened file fo.close()
如果我们编译并运行上面的程序,文件内容将从文件末尾开始读取,直到指定的位置。
Name of the file: foo.txt File Contents: b'\r\nThis is 4th line\r\nThis is 5th line'
示例
tell() 方法与 seek() 方法配合使用。在下面的例子中,我们尝试使用 seek() 方法将文件光标设置到特定位置,然后使用 tell() 方法检索这个设置的位置。
# Open a file fo = open("foo.txt", "r") print("Name of the file: ", fo.name) # Move the pointer backwards using negative offset fo.seek(18, 0) line = fo.read() print("File Contents:", line) #Using tell() method retrieve the cursor position from the ending print("File cursor is present at position", fo.tell()) # Close opened file fo.close()
运行上面的程序后,输出显示为:
Name of the file: foo.txt File Contents: This is 2nd line This is 3rd line This is 4th line This is 5th line File cursor is present at position 88