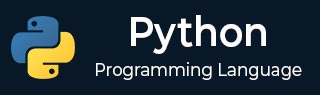
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 排序数组
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 加入线程
- Python - 线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 线程同步
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期和时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
使用 Pygame 在 Python 中开发 Flappy Bird 游戏
在 Flappy Bird 游戏中,玩家通过点击屏幕控制一只鸟,使其在管道之间飞行,而不碰到它们。在这里,我们将使用 pygame 库设计一个 Flappy Bird 游戏。
使用 pygame 设计 Flappy Bird 游戏的步骤
1. 安装 pygame 库
要安装 pygame 库,请使用 PIP 命令。以下是安装它的命令:
pip install pygame
2. 导入库
您需要使用 import 语句导入 pygame 和 random 库。以下是导入这些库的代码语句:
import pygame import random
3. 初始化 Pygame
要初始化 pygame,只需在导入库后调用 pygame.init() 方法。
pygame.init()
4. 设置屏幕
描述屏幕分辨率并通过 pygame.display.set_mode() 方法建立游戏窗口。
5. 定义颜色和属性
您必须设置颜色并为鸟和管道定义属性。
6. 创建绘图函数
创建将在屏幕上为鸟和管道布局的函数。
7. 实现碰撞检测
现在,加入一个函数来确定鸟是否与管道或屏幕边界发生碰撞。
8. 管理管道
开发一个函数,允许进行更改并添加可能需要的新的管道。
9. 运行游戏循环
接收用户的输入,管理游戏状态,识别碰撞并重新绘制界面。
10. 结束游戏
在检测到与 `pygame.quit()` 的帮助下检测到碰撞时退出游戏窗口。
使用 Pygame 开发 Flappy Bird 游戏的 Python 代码
import pygame import random # Initialize Pygame pygame.init() # Screen dimensions SCREEN_WIDTH = 400 SCREEN_HEIGHT = 600 screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT)) pygame.display.set_caption("Flappy Bird") # Colors WHITE = (255, 255, 255) BLACK = (0, 0, 0) GREEN = (0, 255, 0) # Bird properties BIRD_WIDTH = 40 BIRD_HEIGHT = 30 bird_x = 50 bird_y = SCREEN_HEIGHT // 2 bird_velocity = 0 gravity = 0.5 # Gravity flap_strength = -7 # Moderate flap strength # Pipe properties PIPE_WIDTH = 70 PIPE_HEIGHT = 500 PIPE_GAP = 200 # Pipe gap pipe_velocity = -2 # Pipe velocity pipes = [] # Game variables score = 0 clock = pygame.time.Clock() flap_cooldown = 0 # Time before the bird can flap again def draw_bird(): pygame.draw.rect(screen, BLACK, (bird_x, bird_y, BIRD_WIDTH, BIRD_HEIGHT)) def draw_pipes(): for pipe in pipes: pygame.draw.rect(screen, GREEN, pipe['top']) pygame.draw.rect(screen, GREEN, pipe['bottom']) def check_collision(): global score bird_rect = pygame.Rect(bird_x, bird_y, BIRD_WIDTH, BIRD_HEIGHT) for pipe in pipes: if bird_rect.colliderect(pipe['top']) or bird_rect.colliderect(pipe['bottom']): return True if bird_y > SCREEN_HEIGHT or bird_y < 0: return True return False def update_pipes(): global score for pipe in pipes: pipe['top'].x += pipe_velocity pipe['bottom'].x += pipe_velocity if pipe['top'].x + PIPE_WIDTH < 0: pipes.remove(pipe) score += 1 if len(pipes) == 0 or pipes[-1]['top'].x < SCREEN_WIDTH - 300: pipe_height = random.randint(100, SCREEN_HEIGHT - PIPE_GAP - 100) pipes.append({ 'top': pygame.Rect(SCREEN_WIDTH, 0, PIPE_WIDTH, pipe_height), 'bottom': pygame.Rect(SCREEN_WIDTH, pipe_height + PIPE_GAP, PIPE_WIDTH, SCREEN_HEIGHT - pipe_height - PIPE_GAP) }) # Game loop running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.KEYDOWN: if event.key == pygame.K_SPACE and flap_cooldown <= 0: bird_velocity = flap_strength flap_cooldown = 10 # Set cooldown to prevent rapid flapping if event.type == pygame.MOUSEBUTTONDOWN and flap_cooldown <= 0: bird_velocity = flap_strength flap_cooldown = 10 # Set cooldown to prevent rapid flapping # Update bird position bird_velocity += gravity bird_y += bird_velocity # Update pipes update_pipes() # Check for collision if check_collision(): running = False # Manage flap cooldown if flap_cooldown > 0: flap_cooldown -= 1 # Draw everything screen.fill(WHITE) draw_bird() draw_pipes() # Display the score font = pygame.font.SysFont(None, 36) score_text = font.render(f'Score: {score}', True, BLACK) screen.blit(score_text, (10, 10)) pygame.display.flip() clock.tick(30) pygame.quit()
输出
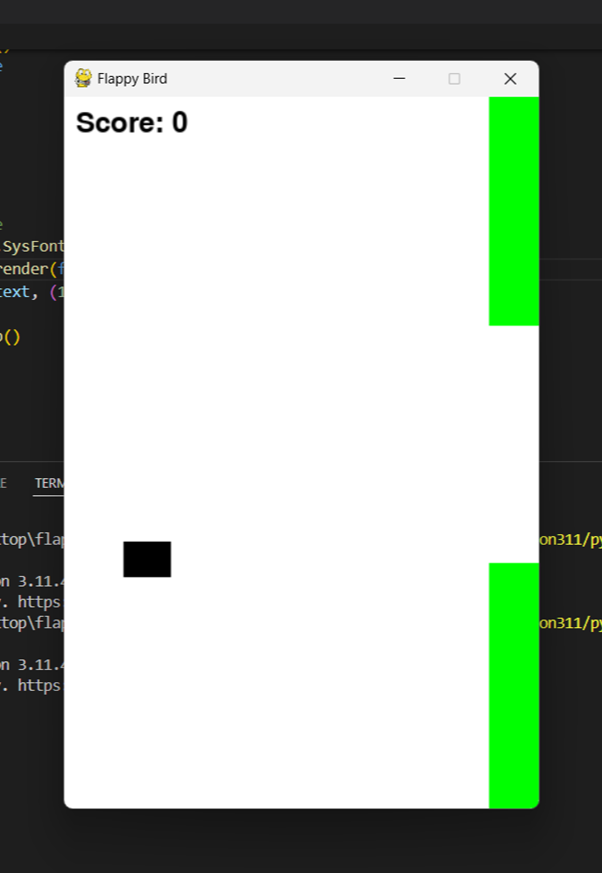
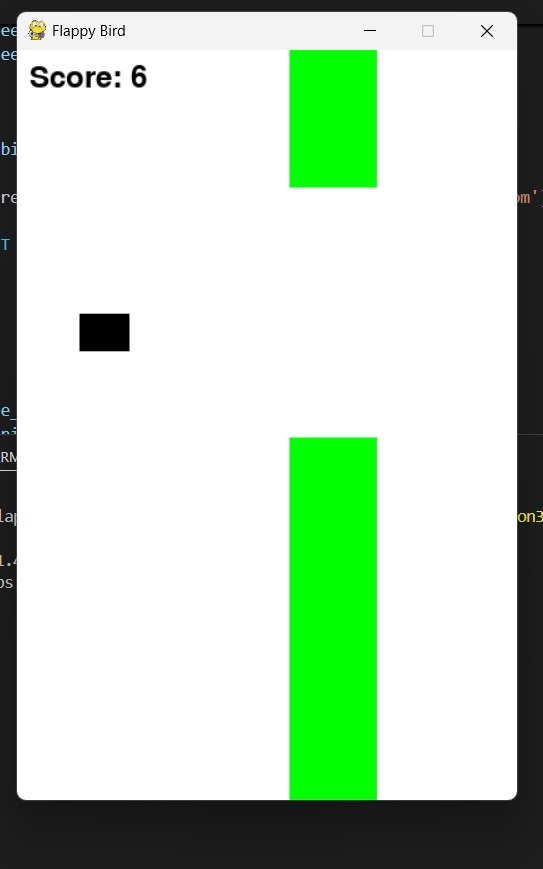
点击鼠标右键,您将获得分数并正确地玩游戏。
Flappy Bird 游戏总结
游戏运行时,会弹出一个标题栏为“Flappy Bird”的窗口,并显示小鸟和管道。小鸟会受到重力的影响,玩家需要使用空格键或鼠标来使小鸟拍打翅膀。管道从屏幕右侧出现并向左移动,玩家需要控制小鸟避免与管道接触。分数位于像素游戏的左上角,随着小鸟穿过管道而增加。
结论
这个使用pygame实现的Flappy Bird游戏是一个简单但非常实用的例子,展示了游戏开发中的一些概念。如果开发者分析并将其应用到自己的游戏中,可以学习到的技能包括精灵移动、碰撞检测和游戏状态管理。还可以加入诸如音效、不同难度级别和改进的图形等功能,使游戏更有趣。
python_projects_from_basic_to_advanced.htm
广告