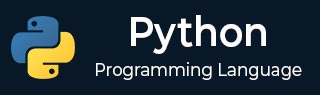
- Python 基础
- Python - 首页
- Python - 概述
- Python - 历史
- Python - 特性
- Python vs C++
- Python - Hello World 程序
- Python - 应用领域
- Python - 解释器
- Python - 环境搭建
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 字面量
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 位运算符
- Python - 成员运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if else
- Python - 嵌套 if
- Python - Match-Case 语句
- Python - 循环
- Python - for 循环
- Python - for-else 循环
- Python - while 循环
- Python - break 语句
- Python - continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数与模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅限关键字参数
- Python - 位置参数
- Python - 仅限位置参数
- Python - 可变参数
- Python - 变量作用域
- Python - 函数注解
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 字符串切片
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表元素
- Python - 修改列表元素
- Python - 添加列表元素
- Python - 删除列表元素
- Python - 遍历列表
- Python - 列表推导式
- Python - 对列表排序
- Python - 复制列表
- Python - 合并列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组
- Python - 访问元组元素
- Python - 更新元组
- Python - 解包元组
- Python - 遍历元组
- Python - 合并元组
- Python - 元组方法
- Python - 元组练习
- Python 集合
- Python - 集合
- Python - 访问集合元素
- Python - 添加集合元素
- Python - 删除集合元素
- Python - 遍历集合
- Python - 合并集合
- Python - 复制集合
- Python - 集合运算符
- Python - 集合方法
- Python - 集合练习
- Python 字典
- Python - 字典
- Python - 访问字典元素
- Python - 修改字典元素
- Python - 添加字典元素
- Python - 删除字典元素
- Python - 字典视图对象
- Python - 遍历字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组元素
- Python - 添加数组元素
- Python - 删除数组元素
- Python - 遍历数组
- Python - 复制数组
- Python - 反转数组
- Python - 对数组排序
- Python - 合并数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python - OS 文件/目录方法
- Python - OS 路径方法
- 面向对象编程
- Python - OOPs 概念
- Python - 类与对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态
- Python - 方法重写
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误与异常
- Python - 语法错误
- Python - 异常
- Python - try-except 块
- Python - try-finally 块
- Python - 抛出异常
- Python - 异常链
- Python - 嵌套 try 块
- Python - 用户自定义异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 合并线程
- Python - 为线程命名
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 库
- NumPy 教程
- Pandas 教程
- SciPy 教程
- Matplotlib 教程
- Django 教程
- OpenCV 教程
- Python 杂项
- Python - 日期与时间
- Python - 数学
- Python - 迭代器
- Python - 生成器
- Python - 闭包
- Python - 装饰器
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式化
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送邮件
- Python - 扩展
- Python - 工具/实用程序
- Python - GUI
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用元类进行元编程
- Python - 模拟和存根
- Python - 猴子补丁
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - Humanize 包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
- Python 有用资源
- Python - 问答
- Python - 在线测验
- Python - 快速指南
- Python - 参考
- Python - 速查表
- Python - 项目
- Python - 有用资源
- Python - 讨论
- Python 编译器
- NumPy 编译器
- Matplotlib 编译器
- SciPy 编译器
用 Python 实现 FLAMES 游戏
FLAMES 游戏是一款有趣且流行的游戏,它使用简单的算法来确定两个名字之间的关系。FLAMES 是以下可能关系的首字母缩写:友情 (Friendship)、爱情 (Love)、好感 (Affection)、婚姻 (Marriage)、敌意 (Enemy) 和兄弟姐妹 (Sibling)。本文将演示如何使用 Python 实现 FLAMES 游戏,并提供对所用概念的解释以及代码示例及其输出。
使用的概念
- 字符串操作 - 从两个字符串中提取和比较字符。
- 数据结构 - 使用列表和集合来管理和比较字符。
- 循环和条件语句 - 遍历字符并应用条件来确定结果。
方法 1:基本实现
步骤 1:基本设置
我们将首先编写一个 Python 函数,该函数根据两个输入名称计算 FLAMES 结果。该方法包括 -
- 删除空格并将名称转换为小写。
- 计算两个名称中每个字符的频率。
- 计算取消后剩余的字符。
- 使用计数来确定 FLAMES 结果。
示例
def flames_game_basic(name1, name2): # Remove spaces and convert to lowercase name1 = name1.replace(" ", "").lower() name2 = name2.replace(" ", "").lower() # Remove common characters for char in name1[:]: if char in name2: name1 = name1.replace(char, "", 1) name2 = name2.replace(char, "", 1) # Count the remaining characters count = len(name1 + name2) # FLAMES outcome based on the count flames = ["Friendship", "Love", "Affection", "Marriage", "Enemy", "Sibling"] while len(flames) > 1: split_index = (count % len(flames)) - 1 if split_index >= 0: flames = flames[split_index + 1:] + flames[:split_index] else: flames = flames[:len(flames) - 1] return f"The relationship is: {flames[0]}" # Test the basic FLAMES game print(flames_game_basic("Alice", "Bob"))
输出
The relationship is: Affection
解释
- 字符串操作 - 程序删除空格并将两个名称转换为小写以保持一致性。
- 删除公共字符 - 删除两个名称之间的公共字符。
- 计算剩余字符 - 计算剩余字符的总数。
- 确定 FLAMES 结果 - 使用计数循环遍历“FLAMES”中的字母以确定关系。
方法 2:带有输入验证的高级实现
在这种方法中,我们通过添加输入验证来增强基本实现,以确保名称是字母数字并且非空。此外,程序允许多轮游戏。
示例
以下是高级实现代码 -
def flames_game_advanced(): while True: name1 = input("Enter the first name: ").strip() name2 = input("Enter the second name: ").strip() # Validate inputs if not name1.isalpha() or not name2.isalpha(): print("Invalid input! Names should only contain alphabets and should not be empty.") continue # Remove common characters for char in name1[:]: if char in name2: name1 = name1.replace(char, "", 1) name2 = name2.replace(char, "", 1) # Count the remaining characters count = len(name1 + name2) # FLAMES outcome based on the count flames = ["Friendship", "Love", "Affection", "Marriage", "Enemy", "Sibling"] while len(flames) > 1: split_index = (count % len(flames)) - 1 if split_index >= 0: flames = flames[split_index + 1:] + flames[:split_index] else: flames = flames[:len(flames) - 1] print(f"The relationship is: {flames[0]}") # Ask if the player wants to play again another = input("Do you want to play again? (yes/no): ").strip().lower() if another != 'yes': break # Run the advanced FLAMES game flames_game_advanced()
输出
Enter the first name: Alice Enter the second name: Bob The relationship is: Marriage Do you want to play again? (yes/no): no
解释
- 输入验证 - 程序确保两个名称仅包含字母字符并且非空。
- 增强的用户交互 - 程序允许用户通过在每一轮之后询问他们是否要再次玩来玩多轮游戏。
结论
FLAMES 游戏是练习 Python 编程技能(包括字符串操作、循环和条件语句)的一种引人入胜的方式。本文探讨了 FLAMES 游戏的基本实现和高级实现,为进一步增强提供了坚实的基础,例如添加更复杂的关系结果或集成图形用户界面 (GUI)。
python_projects_from_basic_to_advanced.htm
广告